Schema URI
www.springframework.org/schema/beans
Schema XSD
www.springframework.org/schema/beans/spring-beans-2.5.xsd
The beans namespace is the core Spring namespace and the one you'll use most when configuring Spring. The root element is the <beans> element. It typically contains one or more <bean> elements, but it may include elements from other namespaces and may not even include a <bean> element at all.
Spring XML Diagram Key
The Spring XML diagrams use the following notations to indicate required elements, cardinality, and containment:

Bean Namespace Diagram
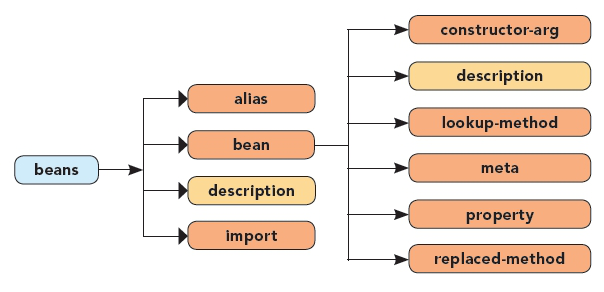
Bean Namespace Elements
Element |
Description |
<alias> |
Creates an alias for a bean definition. |
<bean> |
Defines a bean in the Spring container. |
<constructor-arg> |
Injects a value or a bean reference into an argument of the bean's constructor. Commonly known as constructor injection. |
<description> |
Used to describe a Spring context or an individual bean. Although ignored by the container, <description> can be used by tools that document Spring contexts. |
<import> |
Imports another Spring context definition. |
<lookup-method> |
Enables getter-injection by way of method replacement. Specifies a method that will be overridden to return a specific bean. Commonly known as getter-injection. |
<meta> |
Allows for meta-configuration of the bean. Only useful when there are beans configured that interprets and acts on the meta information. |
<property> |
Injects a value or a bean reference into a specific property of the bean. Commonly known as setter-injection. |
<replaced-method> |
Replaces a method of the bean with a new implementation. |
The <bean> Element Distilled
Even though there are several XML elements that can be used to configure a Spring context, the one you'll probably use the most often is the <bean> element. Therefore, it only seems right that you get to know the attributes of <bean> in detail.
Attribute |
Description |
abstract |
If true, the bean is abstract and will not be instantiated by the Spring container. |
autowire |
Declares how and if a bean should be autowired. Valid values are byType, byName, constructor, autodetect, or no for no autowiring. |
autowire-candidate |
If false, the bean is not a candidate for autowiring into another bean. |
class |
The fully-qualified class name of the bean. |
dependency-check |
Determines how Spring should enforce property setting on the bean. simple indicates that all primitive type properties should be set; objects indicates that all complex type properties should be set. Other value values are default, none, or all. |
depends-on |
Identifies a bean that should be instantiated by the container before this bean is instantiated. |
destroy-method |
Specifies a method that should be invoked when a bean is unloaded from the container. |
factory-bean |
Used with factory-method, specifies a bean that provides a factory method to create this bean. |
factory-method |
The name of a method that will be used instead of the constructor to instantiate this bean. |
id |
The identity of this bean in the Spring container. |
init-method |
The name of a method that should be invoked once the bean has been instantiated and injected. |
lazy-init |
If true the bean will be lazily instantiated. If false, the bean will be eagerly instantiated. |
name |
The name of the bean. This is a weaker alternative to id. |
parent |
Specifies a bean from whom this bean will inherit its configuration. |
scope |
Sets the scope of the bean. By default, all beans are singleton-scoped. Other scopes include prototype, request, and session. |
Bean Namespace Example
The following Spring XML configures two beans, one injected into the other:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd">
<bean id="pirate" class="Pirate">
<constructor-arg value="Long John Silver" />
<property name="map" ref="treasureMap" />
</bean>
<bean id="treasureMap" class="TreasureMap" />
</beans>
The first bean is given "pirate" as its ID and is of type "Pirate." It is to be constructed through a constructor that takes a String as an argument, in this case, it will be constructed with "Long John Silver" as that value. In addition, its "map" property is wired with a reference to the "treasureMap" bean, which is defined as being an instance of TreasureMap.

Don't put all your beans in one XML file.
Once your application gets beyond the trivial stage, you'll likely have an impressive amount of XML in your Spring configuration. There's no reason to put all of that configuration in a single XML file. Keep your Spring configuration more manageable by splitting it across several XML files. Then assemble them all together when creating the application context or by using the <import> element:
<import resource="service-layer-config.xml" />
<import resource="data-layer-config.xml" />
<import resource="transaction-config.xml" />
{{ parent.title || parent.header.title}}
{{ parent.tldr }}
{{ parent.linkDescription }}
{{ parent.urlSource.name }}