10 Examples of ConcurrentHashMap in Java
Below are some of the frequent operations around Java's ConcurrentHashMap, like how to create a ConcurrentHashMap, how to update a key or value.
Join the DZone community and get the full member experience.
Join For FreeIn this article, I have shared some of the frequently used examples of ConcurrentHashMap in Java-like how to create a ConcurrentHashMap, how to update a key or value, how to delete a key-value pair, how to check if a key exists in ConcurrentHashMap or not, how to add new key-value pairs, and how to retrieve values from ConcurrentHashMap in Java.
Once you have gone through these examples, you will have a better understanding of ConcurrentHashMap and you will be more confident in using them in your Java program without causing subtle bugs that are hard to find and fix.
10 Examples of ConcurrentHashMap in Java
Without wasting any more of your time, here are 10 useful examples of ConcurrentHashMap in Java. By these examples, you will learn how to work with ConcurrentHashMap in Java, like creating a map, inserting key-value pairs, updating a key-value pair, deleting a mapping, check if a key or value exists in the map, iterating over keys or values, and so on.
1. How to Create a ConcurrentHashMap With Default Capacity
The first thing first, let's learn how to create a concurrent hashmap in Java. Here is an example of creating an empty ConcurrentHashMapw ith default capacity.
xxxxxxxxxx
ConcurrentHashMap programmingLanguages = new ConcurrentHashMap();
System.out.println("Empty ConcurrentHashMap : " + programmingLanguages);
2. How to Add Objects Into ConcurrentHashMap
Once you have created a ConcurrentHashMap, it's time to add some mapping. let's store some keys and values into a ConcurrentHashMap in Java. If you look at the below code its no different than the HashMa examples of adding mapping which we have seen before, the only difference is that its thread-safe.
xxxxxxxxxx
programmingLanguages.put("Java", Integer.valueOf(18));
programmingLanguages.put("Scala", Integer.valueOf(10));
programmingLanguages.put("C++", Integer.valueOf(31));
programmingLanguages.put("C", Integer.valueOf(41));
System.out .println("ConcurrentHashMap with four mappings : " + programmingLanguages);
3. How to Check if a Key Exists in ConcurrentHashMap or not
Now that you have added mapping, its time to check is key exists in the ConcurrentHashMap or not. This time we will use the containsKey() method from the Map interface which is also available on CHM because CHM implements the Map interface.
xxxxxxxxxx
boolean isJavaExist = programmingLanguages.containsKey("Java");
boolean isPythonExist = programmingLanguages.containsKey("Python");
System.out.printf("Does Programming language Map has %s? %b %n", "Java",
isJavaExist);
System.out.printf("Does Programming language Map contains %s? %b %n", "Python",
isPythonExist);
4. How to Retrieve Values From ConcurrentHashMap in Java
Here is an example of retrieving values from ConcurrentHashMap in Java. This example is very similar to any other map like HashMap or HashTable, as we are using the same get() method to retrieve values from ConcurrentHashMap in Java.
xxxxxxxxxx
int howOldIsJava = programmingLanguages.get("Java");
int howOldIsC = programmingLanguages.get("C");
System.out.printf("How old is Java programming langugae? %d years %n", howOldIsJava);
System.out.printf("How old is C langugae? %d years %n", howOldIsC);
5. How to Check if a Value Exists in ConcurrentHashMap
Here is an example of checking if a value exists in ConcurrentHashMap or not. Again, this example is very similar to the HashMap containsValue() example we have seen before.
xxxxxxxxxx
boolean is41Present = programmingLanguages.containsValue(Integer.valueOf(41));
boolean is31Present = programmingLanguages.containsValue(Integer.valueOf(31));
System.out.printf("Does value 41 is present in ConcurrentHashMap? %b %n", is41Present);
System.out.printf("Does value 31 is present in ConcurrentHashMap? %b %n", is31Present);
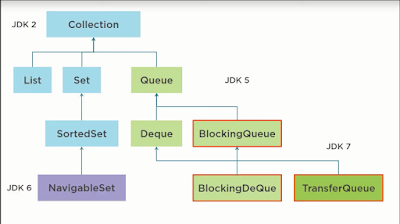
6. How to Find Size of ConcurrentHashMap in Java
You can use the size() method to find out how many key-value pairs are present in the ConcurrentHashMap. The size() method returns the total number of mappings.
xxxxxxxxxx
int numberOfMappings = programmingLanguages.size();
System.out.printf("ConcurrentHashMap %s, contains %d mappings %n",
programmingLanguages, numberOfMappings);
7. How to Loop Over ConcurrentHashMap in Java?
There are multiple ways to loop over a ConcurrentHashMap in Java. In fact, you can use all the four ways to iterate over the Map with ConcurrentHashMap as well. Ultimately, it also implements the java.utill.Map interface hence it obeys the contract of Map
xxxxxxxxxx
Set> entrySet = programmingLanguages.entrySet();
for (Map.Entry mapping : entrySet) {
System.out.printf("Key : %s, Value: %s %n", mapping.getKey(), mapping.getValue());
}
8. PutIfAbsent Example - Adding Keys Only if It's Not Present in ConcurrentHashMap?
This is a useful method which can be used to only insert element if it's not already present in the map or dictionary. This is also a common ConcurrentHashMap interview question which is often asked to beginners.
xxxxxxxxxx
System.out.printf("Before : %s %n", programmingLanguages);
programmingLanguages.putIfAbsent("Java", 22);
System.out.printf("After : %s %n", programmingLanguages);
programmingLanguages.put("Python", 23);
System.out.printf("After : %s %n", programmingLanguages);
9. How to Replace a Mapping in ConcurrentHashMap?
You can use the replace method to update the value of a key in ConcurrentHashMap. This method takes both key and value and updates the old value with the new one as shown below:
xxxxxxxxxx
programmingLanguages.replace("Java", 20);
System.out.println("ConcurrentHashMap After replace : " + programmingLanguages);
10. How to Remove Key-Values From ConcurrentHashMap in Java?
You can use the remove() method of ConcurrentHashMap to remove the mapping from the Map. This method will remove both key and values and the size of ConcurrentHashMap will decrease by one as shown in the following example:
xxxxxxxxxx
programmingLanguages.remove("C++");
System.out.println("ConcurrentHashMap After remove : " + programmingLanguages)
After running this code the mapping for the "C++" key will be removed.
11. How to Remove Keys, While Iterating Over ConcurrentHashMap
Here is the code example of removing keys while iterating over ConcurrentHashMap in Java. Again, it's no different than removing keys from HashMap as we are using the same remove() method from the Map interface which is also inherited by ConcurrentHashMap class in Java.
xxxxxxxxxx
Iterator keys = programmingLanguages.keySet().iterator();
while (keys.hasNext()) {
System.out.printf("Removing key %s from ConcurrentHashMap %n", keys.next());
keys.remove();
}
The remove() method removes the current Key from the ConcurrentHashMap, just like Iterator does for List, Set, and Map.
12. How to Check if ConcurrentHashMap Is Empty in Java?
You can use the isEmpty() method of ConcurrentHashMap to check if the given Map is empty or not. This method will return true if ConcurrentHashMap doesn't have any mapping as shown in the following example:
xxxxxxxxxx
boolean isEmpty = programmingLanguages.isEmpty();
System.out.printf("Is ConcurrentHashMap %s is empty? %b ", programmingLanguages, isEmpty);
ConcurrentHashMap Examples Java
Here is the complete Java Program which you can copy-paste in Eclipse or run it from the command line to play with:
xxxxxxxxxx
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
/** * Java program to demonstrate how to use Concurrent HashMap in Java by simple examples. * * @author javin */
public class ConcurrentHashMapExamples{
public static void main(String args[]) {
// Creates a ConcurrentHashMap with default capacity
ConcurrentHashMap programmingLanguages = new ConcurrentHashMap();
System.out.println("Empty ConcurrentHashMap : " + programmingLanguages); // Adding objects into ConcurrentHashMap
programmingLanguages.put("Java", Integer.valueOf(18));
programmingLanguages.put("Scala", Integer.valueOf(10));
programmingLanguages.put("C++", Integer.valueOf(31));
programmingLanguages.put("C", Integer.valueOf(41));
System.out.println("ConcurrentHashMap with four mappings : " +
programmingLanguages);
// Checking if a key exists in ConcurrentHashMap or not
boolean isJavaExist = programmingLanguages.containsKey("Java");
boolean isPythonExist = programmingLanguages.containsKey("Python");
System.out.printf("Does Programming language Map has %s? %b %n", "Java",
isJavaExist);
System.out.printf("Does Programming language Map contains %s? %b %n",
"Python", isPythonExist);
// Retrieving values from ConcurrentHashMap in Java
int howOldIsJava = programmingLanguages.get("Java");
int howOldIsC = programmingLanguages.get("C");
System.out.printf("How old is Java programming langugae? %d years %n",
howOldIsJava);
System.out.printf("How old is C langugae? %d years %n", howOldIsC);
// Checking if a value exists in ConcurrentHashMap
boolean is41Present = programmingLanguages.containsValue(
Integer.valueOf(41));
boolean is31Present = programmingLanguages.containsValue(
Integer.valueOf(31));
System.out.printf("Does value 41 is present in ConcurrentHashMap? %b %n",
is41Present);
System.out.printf("Does value 31 is present in ConcurrentHashMap? %b %n",
is31Present);
// Finding Size of ConcurrentHashMap
int numberOfMappings = programmingLanguages.size();
System.out.printf("ConcurrentHashMap %s, contains %d mappings %n",
programmingLanguages, numberOfMappings);
// Loop over ConcurrentHashMap in Java
Set> entrySet = programmingLanguages.entrySet();
for (Map.Entry mapping : entrySet) {
System.out.printf("Key : %s, Value: %s %n", mapping.getKey(),
mapping.getValue());
}
//PutIfAbsent Example - Adding keys only if its not present in
//ConcurrentHashMap
System.out.printf("Before : %s %n", programmingLanguages);
programmingLanguages.putIfAbsent("Java", 22); // Already exists
System.out.printf("After : %s %n", programmingLanguages);
programmingLanguages.put("Python", 23); // Added
System.out.printf("After : %s %n", programmingLanguages);
// Replacing a Mapping in ConcurrentHashMap
programmingLanguages.replace("Java", 20);
System.out.println("ConcurrentHashMap After replace : " +
programmingLanguages);
// Removing key values from ConcurrentHashMap
programmingLanguages.remove("C++");
System.out.println("ConcurrentHashMap After remove : " +
programmingLanguages);
// Removing Keys, while Iterating over ConcurrentHashMap
Iterator keys = programmingLanguages.keySet().iterator();
while (keys.hasNext()) {
System.out.printf("Removing key %s from ConcurrentHashMap %n",
keys.next());
keys.remove();
}
// How to check if ConcurrentHashMap is empty
boolean isEmpty = programmingLanguages.isEmpty();
System.out.printf("Is ConcurrentHashMap %s is empty? %b ",
programmingLanguages, isEmpty);
}
}
Output:
xxxxxxxxxx
Empty ConcurrentHashMap : {}
ConcurrentHashMap with four mappings : {C=41, Scala=10, Java=18, C++=31}
Does Programming language Map has Java? true
Does the Programming language Map contain Python? false
How old is Java programming language? 18 years
How old is C language? 41 years
Does value 41 is present in ConcurrentHashMap? true
Does value 31 is present in ConcurrentHashMap? true
ConcurrentHashMap {C=41, Scala=10, Java=18, C++=31}, contains 4 mappings
Key: C, Value: 41
Key: Scala, Value: 10
Key: Java, Value: 18
Key : C++, Value: 31
Before : {C=41, Scala=10, Java=18, C++=31}
After : {C=41, Scala=10, Java=18, C++=31}
After : {C=41, Python=23, Scala=10, Java=18, C++=31}
ConcurrentHashMap After replace : {C=41, Python=23, Scala=10, Java=20, C++=31}
ConcurrentHashMap After remove : {C=41, Python=23, Scala=10, Java=20}
Removing key C from ConcurrentHashMap
Removing key Python from ConcurrentHashMap
Removing key Scala from ConcurrentHashMap
Removing key Java from ConcurrentHashMap
Is ConcurrentHashMap {} is empty? true
That's all about ConcurrentHashMap examples in Java. As I said, after going through these examples, you will have a better understanding of How ConcurrentHashMap works and how to use it properly. Now you have a good idea of how to create, add, update, search, and delete entries on a ConcurrentHashMap in Java.
Thanks for reading this article so far. If you like this article then please share it with your friends and colleagues. If you have any questions or feedback then please drop a note.
Published at DZone with permission of Javin Paul, DZone MVB. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments