Linq performance with Count() and Any()
Join the DZone community and get the full member experience.
Join For Free Recently I was using resharper to refactor some of my code and found that it was suggesting to use any() extension method instead of count() method in List<T>. I was really keen about performance and found that resharper was right. There is huge performance difference between any() and count() if you are using count() just to check whether list is empty or not.
Here in the above code you can see that I created ‘Customer’ class which has simple two properties ‘CustomerId’ and ‘CustomerName’. Then in Main method I have created a list of customers and used for loop and object intializer to fill customers list. After that I have written a code to measure time for count and any with ‘Stop watch’ class and printing CPU ticks. It’s pretty simple.
Now let’s run this console application to see output.
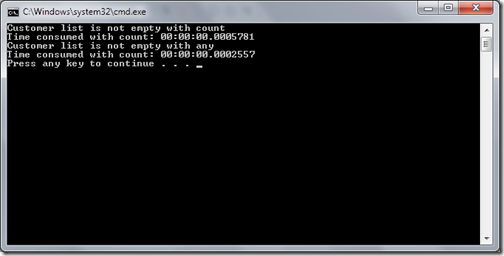
Hope you like it. Stay tuned for more...
Difference between Count() and Any():
In Count() method code will traverse all the list and get total number of objects in list while in Any() will return after examining first element in the sequence. So in list where we have many object it will be significant execution time if we use count().Example:
Let’s take an example to illustrate this scenario. Following is a code for that.using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; namespace ConsoleApplication3 { class Program { static void Main() { //Creating List of customers List<Customer> customers = new List<Customer>(); for (int i = 0; i <= 100; i++) { Customer customer = new Customer { CustomerId = i, CustomerName = string.Format("Customer{0}", i) }; customers.Add(customer); } //Measuring time with count Stopwatch stopWatch = new Stopwatch(); stopWatch.Start(); if (customers.Count > 0) { Console.WriteLine("Customer list is not empty with count"); } stopWatch.Stop(); Console.WriteLine("Time consumed with count: {0}", stopWatch.Elapsed); //Measuring time with any stopWatch.Restart(); if (customers.Any()) { Console.WriteLine("Customer list is not empty with any"); } stopWatch.Stop(); Console.WriteLine("Time consumed with count: {0}", stopWatch.Elapsed); } } public class Customer { public int CustomerId { get; set; } public string CustomerName { get; set; } } }
Here in the above code you can see that I created ‘Customer’ class which has simple two properties ‘CustomerId’ and ‘CustomerName’. Then in Main method I have created a list of customers and used for loop and object intializer to fill customers list. After that I have written a code to measure time for count and any with ‘Stop watch’ class and printing CPU ticks. It’s pretty simple.
Now let’s run this console application to see output.
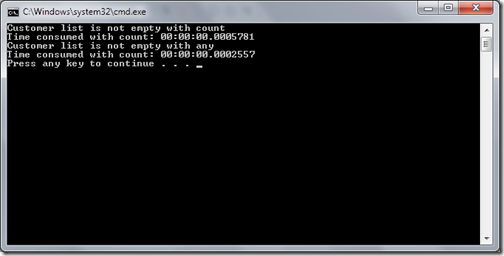
Conclusion:
Here in the above example you can see there is huge performance benefit of using any() instead of count() when we are checking whether list<T> is empty or not.Hope you like it. Stay tuned for more...
Extension method
Object (computer science)
application
Printing
Console (video game CLI)
Execution (computing)
IT
Measure (physics)
Published at DZone with permission of Jalpesh Vadgama, DZone MVB. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments