JSON to XML Transformation Using DataWeave
DataWeave can be used to transform JSON to XML. This example looks at an API that returns information about books in JSON with a backend that only accepts XML input.
Join the DZone community and get the full member experience.
Join For FreeIn this blog, I am going to explain how to transform JSON to XML using DataWeave. I will cover the use of variables and functions. I will also show the use of a couple of operators in DataWeave to achieve the desired output.
Let's take an example of an API that returns information about books in JSON. My backend only accepts XML input. The XML contains the total number of books. Books are sorted by the year in which they were published. Price for a particular book is displayed only if it is greater than 30.00.
Input JSON is in the following format:
[{
"title" : "Only Time Will Tell",
"author" : "Jeffrey Archer",
"year" : "2011",
"price" : "30.00"
}, {
"title" : "Private India: City on Fire",
"author" : "James Patterson",
"author" : "Ashwin Sanghi",
"year" : "2014",
"price" : "49.99"
}, {
"title" : "Harry Potter and the Goblet of Fire",
"author" : "J K. Rowling",
"year" : "2005",
"price" : "29.99"
}, {
"title" : "Twilight",
"author" : "Stephenie Meyer",
"year" : "2007",
"price" : "39.95"
}
]
Output XML is in this format:
<bks:bookstore xmlns:bks="http://example.com/bookstore">
<bks:totalBooks>4</bks:totalBooks>
<bk:book xmlns:bk="http://example.com/books" title="http://example.com/books/book?title='Harry Potter and the Goblet of Fire'">
<year>2005</year>
<author>J K. Rowling</author>
</bk:book>
<bk:book xmlns:bk="http://example.com/books" title="http://example.com/books/book?title='Twilight'">
<year>2007</year>
<price>39.95</price>
<author>Stephenie Meyer</author>
</bk:book>
<bk:book xmlns:bk="http://example.com/books" title="http://example.com/books/book?title='Only Time Will Tell'">
<year>2011</year>
<author>Jeffrey Archer</author>
</bk:book>
<bk:book xmlns:bk="http://example.com/books" title="http://example.com/books/book?title='Private India: City on Fire'">
<year>2014</year>
<price>49.99</price>
<author>James Patterson</author>
<author>Ashwin Sanghi</author>
</bk:book>
</bks:bookstore>
For achieving the desired result, execute the following steps.
1. Create a New Project in Anypoint Studio
Then, add HTTP Connector and configure it. Provide /bookstore as Base Path in Connector Properties.
2. Add a Transform Message (DataWeave) Component
Define input and output metadata using above JSON and XML files as examples.
3. Remove {} in the DataWeave Code Section
We do this because we want to define our own code. Don't worry if any errors are shown.
4. Define namespaces in the DataWeave Header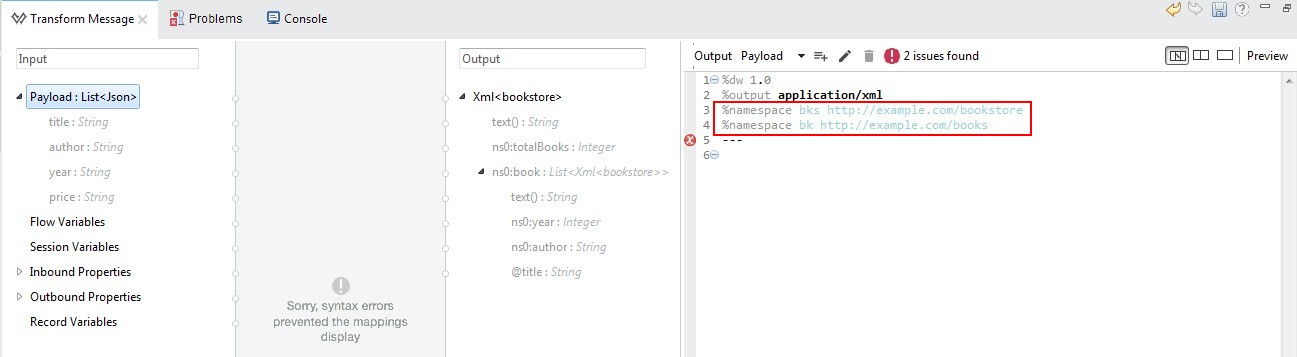
5. Define the bookstore Element
Check Preview to see bookstore element generated.
6. Enter payload in Curly Braces in the bookstore Element
We get errors because input payload is an array and XML is an object.
7. Add Brackets Around payload
This will fix our errors. In Preview, I can see that elements from all key-value pairs in payload are generated.
8. Add a Space and Then map {} After Payload
We do this because we want to iterate through all objects in input to create the book element. For this, we will have to use map operator.
9. Create book Element Inside Curly Braces in the map
Also define the year, price, and author elements as shown below.
10. Use the map Operator on the author Element
In Preview, I see that the author element with value Ashwin Sanghi is not generated. This is because there are 2 key-value pairs in the second object in payload. So, it should be mapped as an array. For this, use map operator on author element as shown below and as seen in Preview, two author elements are generated.
11. Define the Attribute title
We do this because we want to show the title of the book as an attribute of book.
12. Define a Variable With the Value as a URL
In the output XML, the title is displayed as a URL. For this, we will define the variable with the value as URL and then use a function for appending title to this URL, as shown below.
13. Change the title Attribute to use the newUrl Function
In Preview, I can see that title of the book is generated in URL form.
14. Use the when Operator to Check Price
In Preview, I can see that price element is not generated for all books. It is only generated if price is greater than 30.00. To handle this, use the when operator to check the price as shown below.
15. Use the orderBy Operator
Books are sorted by year in the output XML. I can achieve this using the orderBy operator and in Preview, we can see that books are sorted by year now.
16. Show the Total Number of Books in the Output
We also want to show the total number of books in the output. Use the sizeOf operator for this.
To test this project, run the project in Anypoint Studio first. Once the project is successfully deployed, send input JSON as input to http://localhost:8081/bookstore using Postman or some other client for sending REST requests. In the response, I should get desired output XML as shown below.
I have shown how to transform input JSON into output XML. I have also shown how to define namespaces used in output XML. Also, I have shown how we can declare variables and functions and how to use operators like map, when, orderBy, and sizeOf in DataWeave.
Opinions expressed by DZone contributors are their own.
Comments