Test automation of REST APIs Using ZeroCode Open Source JSON-Based Test Framework
See how the ZeroCode testing library can make your life easier by reducing boilerplate code, automating your tests, and more.
Join the DZone community and get the full member experience.
Join For FreeMany folks tend not to write tests because sometimes it is harder to think up a use case scenario and put into actual executable test.
Testing need not be harder or slower, it should be easier and faster.
This post will explain how we can design better test cases whether it is TDD or BDD using Zerocode open source framework. You will find it effortless and fun when you don't have to write any step definitions, feature files, multiple assertThat, because the framework has simplified all these hassles for you amd made it implicit to write the tests.
It brings the simplicity in testing and validating APIs by eliminating repetitive code for test assertions, http calls and payload parsing. It's powerful JSON comparison and assertions make the testing cycle a lot easy and clean.
Developers find Zerocode very easy and intuitive to express the test intentions via the declarative JSON steps.
See Hello- GitHub API Test Step and HelloWorldTest, the README file.
The test cases are simple and looks like below JSON steps, the rest of the things like invoking the end point using the HttpClient, receiving the response and asserting the outcome is taken care by the framework.
Your Given-When-Then User-Journey Scenario/AC,
AC1:
GIVEN- the POST api end point '/api/v1/users' to create an user,
WHEN- I invoke the API,
THEN- I will receive the 201 status with the new user ID and headers
AND- I will assert the response
AC2:
GIVEN- the REST api GET end point '/api/v1/users/${created-User-Id}',
WHEN- I invoke the API,
THEN- I will receive the 200 status with body and headers
AND- I will assert the response
precisely translates to the below JSON test steps - Simple and clean !
The Pass and Fail cases shows how easily a test case can be represented n read-
Why?
Zerocode helps you to focus on test scenarios and ACs(Acceptance Criteria) , without any distractions of unnecessary syntax overhead.
Enables you to automate your API Contract tests, end-to-end integration-tests at the speed of writing unit tests.
Displays step's all assertions failures at once, than exiting at first failure.
Exampe of a scenario with POST-PUT-GET steps hooked with assertions, precisely looks like below:
{
"scenarioName": "Create, Update and GET Employee Details Happy and Sad test",
"steps": [
{
"name": "new_emp",
"url": "http://host:port/api/v1/persons",
"operation": "POST",
"request": {
"name": "Larry P",
"job": "Full Time"
},
"assertions": {
"status": 201,
"body": {
"id": "1001"
}
}
},
{
"name": "update_emp",
"url": "http://host:port/api/v1/persons/${$.new_emp.response.id}",
"operation": "PUT",
"request": {
"name": "Larry Page",
"job": "Co-Founder"
},
"assertions": {
"status": 200,
"body": {
"id": "${$.new_emp.response.id}" //This is optional but better than hardcoding ${JSON Path to created Id}
"name": "${$.update_emp.request.name}",
"job": "${$.update_emp.request.job}"
}
}
},
{
"name": "get_emp_details",
"url": "http://host:port/api/v1/persons/${$.new_emp.response.id}",
"operation": "GET",
"request": {},
"assertions": {
"status": 200,
"body": {
"id": "${$.new_emp.response.id}"
"name": "${$.update_emp.request.name}",
"job": "${$.update_emp.request.job}"
}
}
},
{
"name": "get_non_existing_emp_details",
"url": "http://host:port/api/v1/persons/9999",
"operation": "GET",
"request": {},
"assertions": {
"status": 404,
"body": {
"message": "No such employee exists"
}
}
}
]
}
Then you just stick these into a JSON file, for example, named "get_happy_and_sad.json"
, anywhere in the test/resources
folder. Then run the code like below, pointing to that JSON file
, and then you are done with testing. It's neat and as simple as running a JUnit @Test.
@TargetEnv("host_config.properties")
@RunWith(ZeroCodeUnitRunner.class)
public class MyRestApiTest{
@Test
@JsonTestCase("get_happy_and_sad.json")
public void testGetHappyAndSad() throws Exception {
}
}
Where the "host_config.properties" has the host/port/context details as below
web.application.endpoint.host=https://api.project.com
web.application.endpoint.port=443
web.application.endpoint.context=
Maven and Gradle:
- The
latest
Maven dependency can be found at maven-central-zerocode.
<dependency>
<groupId>org.jsmart</groupId>
<artifactId>zerocode-rest-bdd</artifactId>
<version>1.2.x</version>
</dependency>
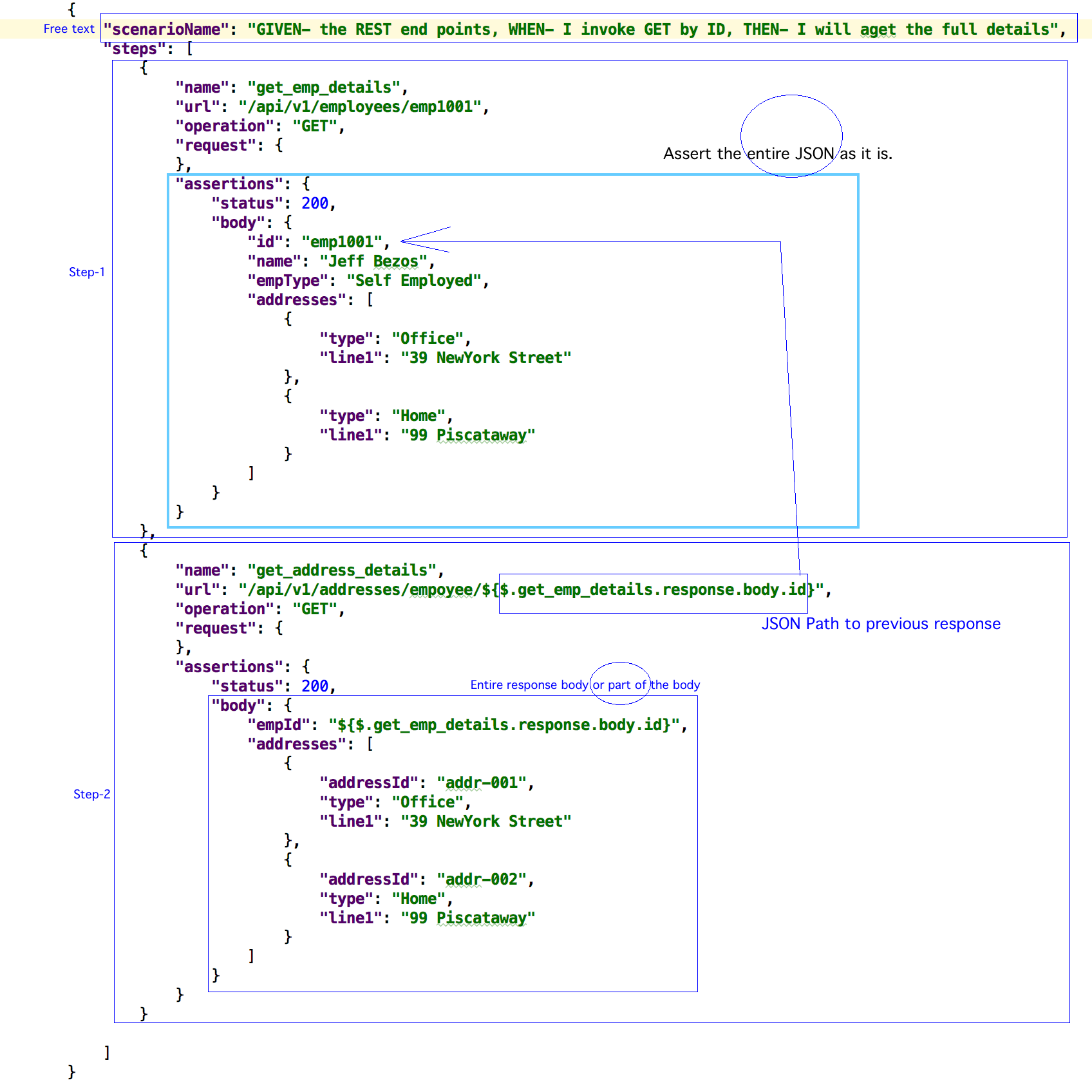
You can assert the entire JSON in the assertion block, however complex and hierarchical the structure might be, with a
copy paste
of the entire JSON. Hassle free, no serialize/deserialize as needed!You can also use only the particular section or even an element of a JSON using a JSON path like
$.get_emp_details.response.body.id
, which will resolve to1001
in the above case.You can test the consumer contract APIs by creating runners specific to clients.
Test Report
Test reports are generated into the /target
folder every time the tests are run. Sample reports are here as Interactive and Searchable(filter) HTML .html Spike Chart
and .csv tabular format. See more about reports here.
Test Logs
Test logs are generated in the console as well as into the log file in a readable JSON format, target/logs/zerocode_rest_bdd_logs.log
. In case of a test failure, it lists which field or fields didn't match with their JSON Path
in a tree structure.
For example, if the test passed:
If the test failed:
If there are more fields mismatches i.e. "assertions" block doesnt match with "actual" response, then the output displays like below-
(You can run yourself the below step via the unit test from the hello-world repo )
Examples
HelloWorldTest and more working examples are here.
You can use placeholders for various outcomes if you need.
Examples of some features are here:
Re-using Request/Response via JSON Path.
Generating custom IDs example here.
Source Code in GitHub
Visit the source here in GitHub ZeroCode.
Running tests parallelly
Tests can be run parallely both ways, Class wise or Method wise.
Contribute
Raise issues and contribute to improve the ZeroCode library and add more essential features you need by talking to the author.
For quick, concise and short answers on questions you can optionally put your queries in gitter.
Opinions expressed by DZone contributors are their own.
Comments