How to Test DELETE Requests for API Testing With Playwright Java
This tutorial demonstrates how to test DELETE requests using the Playwright Java framework for API testing, using examples of deleting data using an API.
Join the DZone community and get the full member experience.
Join For FreeAPI testing has gained a lot of momentum these days. As UI is not involved, it is a lot easier and quicker to test. This is the reason why API testing is considered the first choice for performing end-to-end testing of the system. Integrating the automated API Tests with the CI/CD pipelines allows teams to get faster feedback on the builds.
In this blog, we'll discuss and learn about DELETE API requests and how to handle them using Playwright Java for automation testing, covering the following points:
- What is a DELETE request?
- How do you test DELETE APIs using Playwright Java?
Getting Started
It is recommended that you check out the earlier tutorial blog to learn about the details related to prerequisites, setup, and configuration.
Application Under Test
We will be using the free-to-use RESTful e-commerce APIs that offer multiple APIs related to order management functionality, allowing us to create, retrieve, update, and delete orders.
This application can be set up locally using Docker or NodeJS.
What Is a DELETE Request?
A DELETE API request deletes the specified resource from the server. Generally, there is no response body in the DELETE requests.
The resource is specified by a URI, and the server permanently deletes it. DELETE requests are neither considered safe nor idempotent, as they may cause side effects on the server, like removing data from a database.
The following are some of the limitations of DELETE requests:
- The data deleted using a DELETE request is not reversible, so it should be handled carefully.
- It is not considered to be a safe method as it can directly delete the resource from the database, causing conflicts in the system.
- It is not an idempotent method, meaning calling it multiple times for the same resource may result in different states. For example, in the first instance, when DELETE is called, it will return Status Code 204 stating that the resource has been deleted, and if DELETE is called again on the same resource, it may give a 404 NOT FOUND as the given resource is already deleted.
The following is an example of the DELETE API endpoint from the RESTful e-commerce project.
DELETE /deleteOrder/{id}
: Deletes an Order By ID
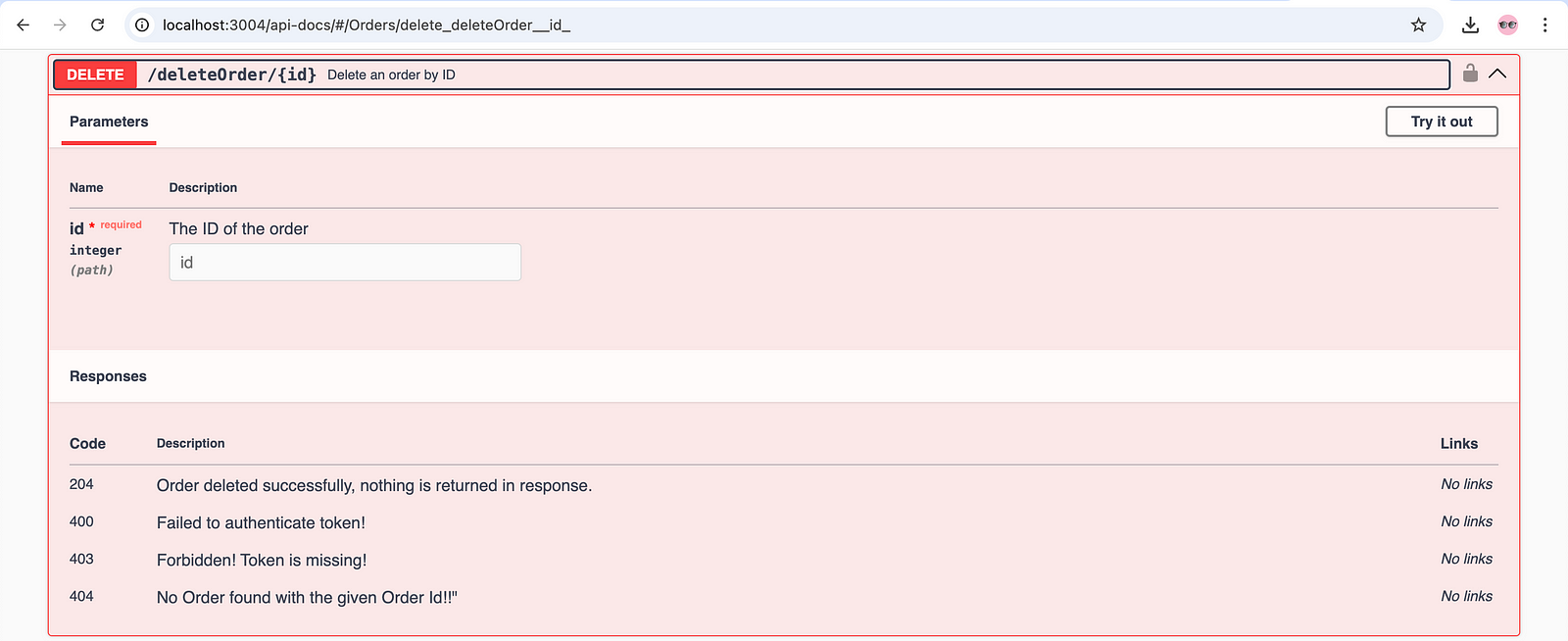
This API requires the order_id
to be supplied as Path Parameter in order to delete respective order from the system. There is no request body required to be provided in this DELETE API request. However, as a security measure, the token
is required to be provided as a header
to delete the order.
Once the API is executed, it deletes the specified order from the system and returns Status Code 204.
In case where the order is not found, or the token is not valid or not provided, it will accordingly show the following response:
Status Code | Description |
---|---|
400 |
Failed to authenticate the token |
404 |
No order with the given |
403 | Token is missing in the request |
How to Test DELETE APIs Using Playwright Java
Testing DELETE APIs is an important step in ensuring the stability and reliability of the application. Correct implementation of the DELETE APIs is essential to check for unintended data loss and inconsistencies, as the DELETE APIs are in charge of removing the resources from the system.
In this demonstration of testing DELETE APIs using Playwright Java, we'll be using the /deleteOrder/{id}
for deleting an existing order from the system.
Test Scenario 1: Delete a Valid Order
- Start the RESTful e-commerce service.
- Using a POST request, create some orders in the system.
- Delete the order with
order_id
“1” using DELETE request. - Check that the Status Code 204 is returned in the response.
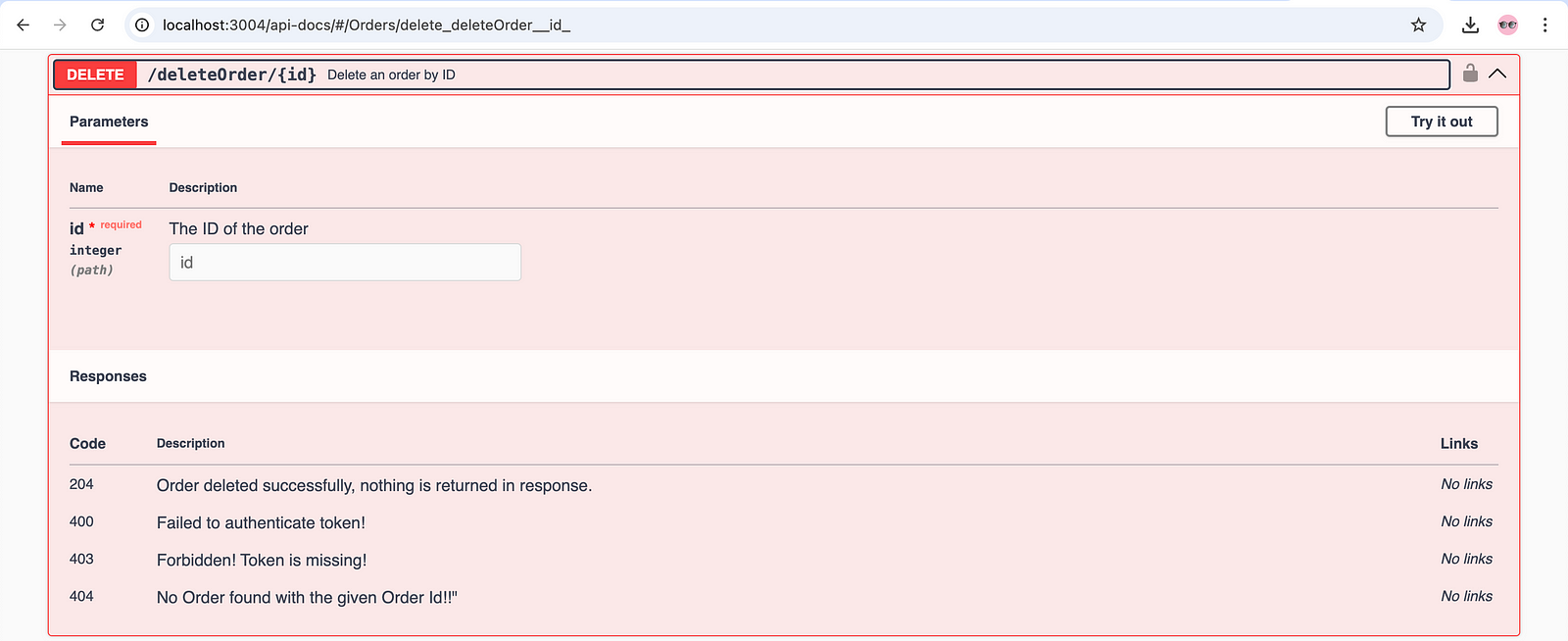
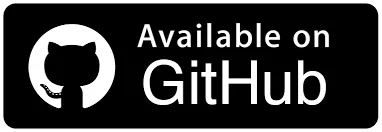
Test Implementation
The following steps are required to be performed to implement the test scenario:
- Add new orders using the POST request.
- Hit the
/auth
API to generate token. - Hit the
/deleteOrder/
API endpoint with the token and theorder_id
to delete the order. - Check that the Status Code 204 is returned in the response.
A new test method, testShouldDeleteTheOrder()
, is created in the existing test class HappyPathTests
. This test method implements the above three steps to test the DELETE API.
@Test
public void testShouldDeleteTheOrder() {
final APIResponse authResponse = this.request.post("/auth", RequestOptions.create().setData(getCredentials()));
final JSONObject authResponseObject = new JSONObject(authResponse.text());
final String token = authResponseObject.get("token").toString();
final int orderId = 1;
final APIResponse response = this.request.delete("/deleteOrder/" + orderId, RequestOptions.create()
.setHeader("Authorization", token));
assertEquals(response.status(), 204);
}
The POST /auth
API endpoint will be hit first to generate the token. The token
received in response is stored in the token variable to be used further in the DELETE API request.

Next, new orders will be generated using the testShouldCreateNewOrders()
method, which is already discussed in the previous tutorial, where we talked about testing POST requests using Playwright Java.
After the orders are generated, the next step is to hit the DELETE request with the valid order_id
that would delete the specific order.

We'll be deleting the order with the order_id
“1” using the delete()
method provided by Playwright framework.
After the order is deleted, the Status Code 204 is returned in response. An assertion will be performed on the Status Code to verify that the Delete action was successful. Since no request body is returned in the response, this is the only thing that can be verified.
Test Execution
We'll be creating a new testng.xml named testng-restfulecommerce-deleteorders.xml
to execute the tests in the order of the steps that we discussed in the test implementation.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Restful ECommerce Test Suite">
<test name="Testing Happy Path Scenarios of Creating and Updating Orders">
<classes>
<class name="io.github.mfaisalkhatri.api.restfulecommerce.HappyPathTests">
<methods>
<include name="testShouldCreateNewOrders"/>
<include name="testShouldDeleteTheOrder"/>
</methods>
</class>
</classes>
</test>
</suite>
First, the testShouldCreateNewOrders()
test method will be executed, and it will create new orders. Next, the testShouldDeleteTheOrder()
test method order will be executed to test the delete order API.
The following screenshot of the test execution performed using IntelliJ IDE shows that the tests were executed successfully.
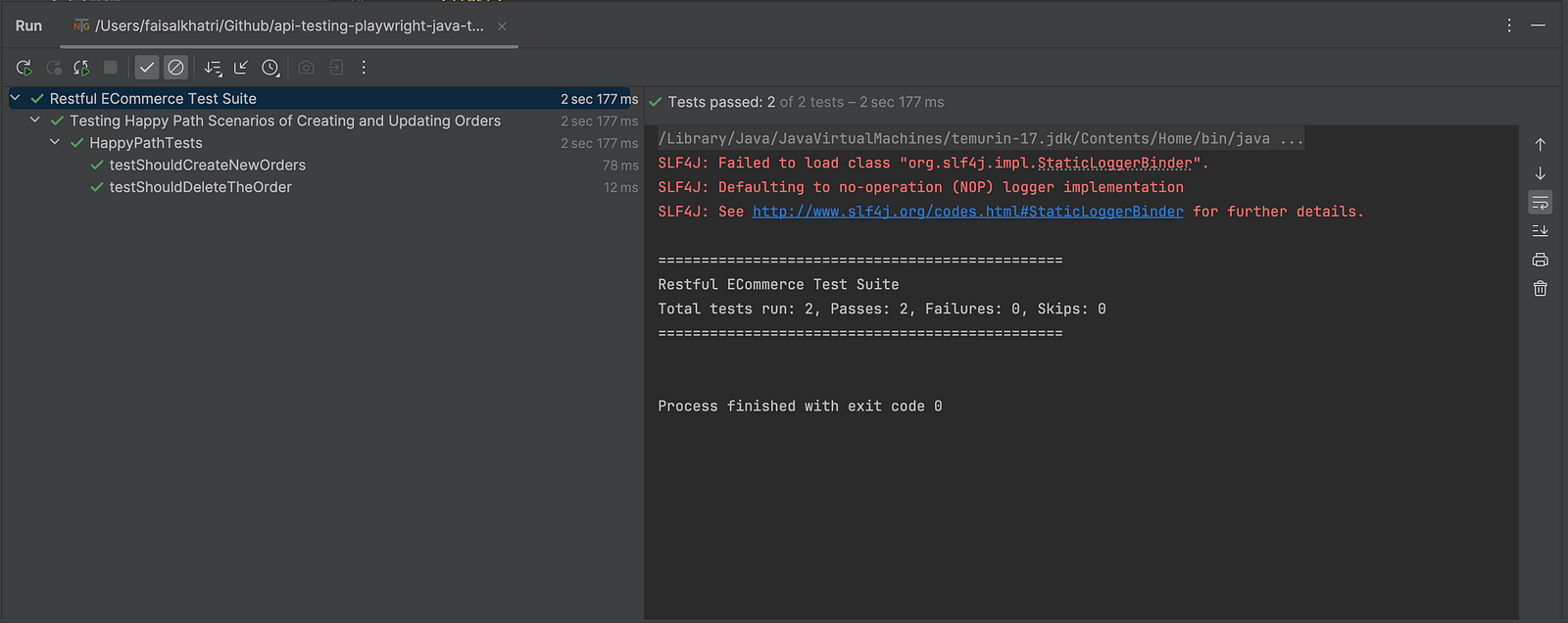
Now, let’s verify that the order was correctly deleted by writing a new test that will call the GET /getOrder
API endpoint with the deleted order_id
.
Test Scenario 2: Retrieve the Deleted Order
- Delete a valid order with
order_id
“1.” - Using GET
/getOrder
API, try retrieving the order withorder_id
“1.” - Check that the Status Code 404 is returned with the message “No Order found with the given parameters!” in the response.
Test Implementation
Let’s create a new test method, testShouldNotRetrieveDeletedOrder()
, in the existing class HappyPathTests
.
@Test
public void testShouldNotRetrieveDeletedOrder() {
final int orderId = 1;
final APIResponse response = this.request.get("/getOrder", RequestOptions.create().setQueryParam("id", orderId));
assertEquals(response.status(), 404);
final JSONObject jsonObject = new JSONObject(response.text());
assertEquals(jsonObject.get("message"), "No Order found with the given parameters!");
}
The test implementation of this scenario is pretty simple. We will be executing the GET /getOrder
API and to fetch the deleted order with order_id
“1.”
An assertion is applied next to verify that the GET API should return the Status Code 404 in the response with the message “No Order found with the given parameters!”
This test ensures that the delete order API worked fine and the order was deleted from the system.
Test Execution
Let’s update the testng.xml
file and add this test scenario at the end after the delete test.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Restful ECommerce Test Suite">
<test name="Testing Happy Path Scenarios of Creating and Updating Orders">
<classes>
<class name="io.github.mfaisalkhatri.api.restfulecommerce.HappyPathTests">
<methods>
<include name="testShouldCreateNewOrders"/>
<include name="testShouldDeleteTheOrder"/>
<include name="testShouldNotRetrieveDeletedOrder"/>
</methods>
</class>
</classes>
</test>
</suite>
Now, all three tests should run in sequence. The first one will create orders; the second one will delete the order with order_id
“1”; and the last test will hit the GET API to fetch the order with order_id
“1” returning Status Code 404.
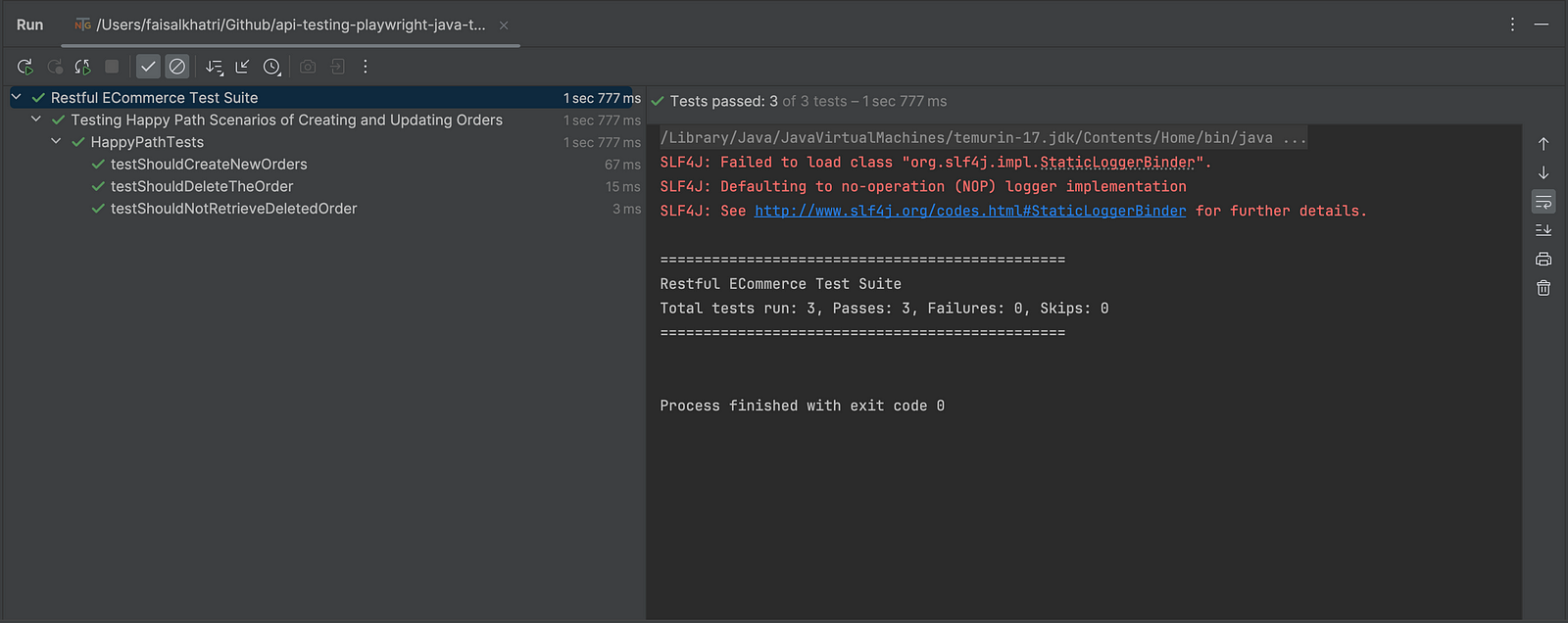
The screenshot above shows that all three tests were executed successfully, and the DELETE API worked fine as expected.
Summary
DELETE API requests allow the deletion of the resource from the system. As delete is an important CRUD function, it is important to test it and verify that the system is working as expected. However, it should be noted that DELETE is an irreversible process, so it should always be used with caution.
As per my experience, it is a good approach to hit the GET API after executing the DELETE request to check that the specified resource was deleted from the system successfully.
Happy testing!
Published at DZone with permission of Faisal Khatri. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments