Spring Boot Config Documentation, Two Ways With IntelliJ IDEA
One Spring Boot documentation, two ways.
Join the DZone community and get the full member experience.
Join For FreeIn modern software development, applications are driven by configuration. If configurable properties are large in numbers, then it is difficult to remember the purpose, structure, or type of each property. It then becomes a necessity to invest in the documentation of these properties.
This article explores IDE-integrated options to assist in writing Spring Boot YAML-based configuration.
You may also like: 7 Things to Know for Getting Started With Spring Boot
Spring Configuration Processor¹
Spring Boot provides an out-of-the-box mechanism for configuration documentation: include a jar named spring-boot-configuration-processor
and trigger build.
annotationProcessor 'org.springframework.boot:spring-boot-configuration-processor'
How It Works
- You trigger build
- It scans
@ConfigurationProperties
and constructs a JSON file that describes the overall properties.{ "groups": [ { "name": "db", "type": "mighty.config.Database", "sourceType": "mighty.config.Database" } ], "properties": [ { "name": "db.hostname", "type": "java.lang.String", "sourceType": "mighty.config.Database" }, { "name": "db.password", "type": "java.lang.Character[]", "sourceType": "mighty.config.Database" }, { "name": "db.port", "type": "java.lang.Integer", "sourceType": "mighty.config.Database", "defaultValue": 0 }, { "name": "db.username", "type": "java.lang.String", "sourceType": "mighty.config.Database" } ], "hints": [] }
- Your IDE intercepts the generated file and helps you write the configuration.
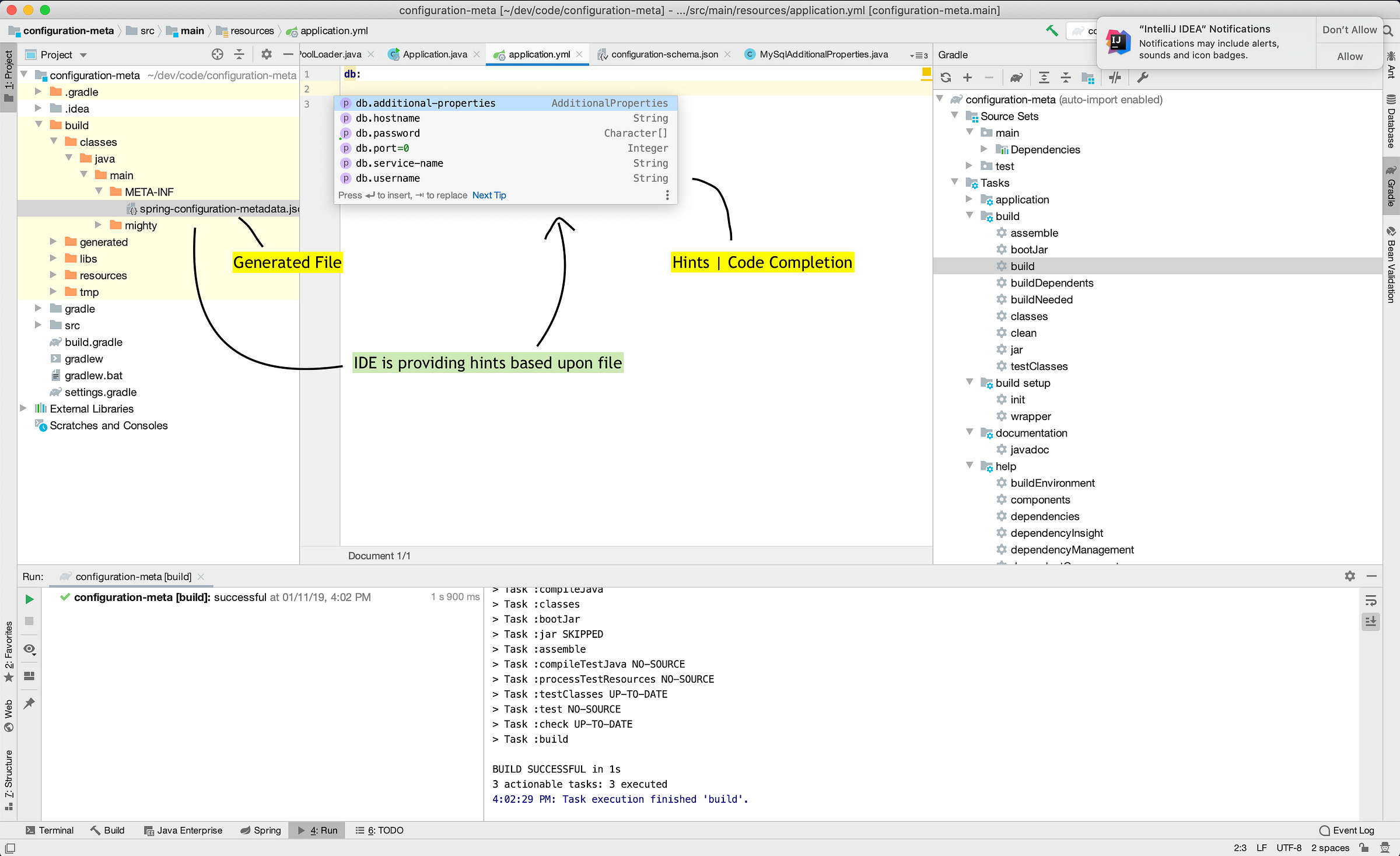
IntelliJ IDEA — Ultimate Edition detects the Spring meta processor on the classpath and provides hints based upon the metadata file generated.
However, there is no support on IntelliJ IDEA — Community Edition, Spring Tools 4 (Eclipse with Spring tools), and Visual Code (with Spring tools).
YAML Schema
The YAML schema is a newer, still evolving, and powerful option. In reality, "the YAML schema is written in JSON format"² (strange!).
At the time of writing, Draft#8 or 2019–09 has been released , including tools like Visual Studio Code (with the YAML extension by Red Hat), IntelliJ IDEA 2019.x support Draft#7.
How It Works
- You write your schema by hand.
{ "$schema": "http://json-schema.org/draft-07/schema#", "$id": "mighty/db", "title": "Database Configuration", "description": "Applicable database properties", "type": "object", "properties": { "db": { "type": "object", "description": "DB configuration", "properties": { "hostname": { "type": "string", "description": "Hostname of db" }, "port": { "type": "integer", "default": 0, "description": "port of db" }, "serviceName": { "type": "string" }, "username": { "type": "string", "description": "DB user" }, "password": { "type": "string", "description": "DB Password" } }, "required": [ "hostname", "port"] } } }
- You create schema-mapping: mapping of files against schema governance.
Mapping Definition | IntelliJ IDEA Community Edition - IDE provides hints based upon schema provided to helps you write schema efficiently.
-
Spring Configuration Metadata Vs. YAML Schema
Now that we have seen both ways, let us now compare.
Case #1: Static Properties
Consider a configuration that accepts some fixed sets of properties related to database connection.
db:
hostname:
port:
username:
password:
Here is the IDE performance on both approaches:

The types of properties are not shown in suggestions in the case of the YAML schema, yet it suggests the type while validating a property. With Spring Boot Configuration Metadata, the Java exception message is shown on "Type Mismatch."
Case #2: Dynamic Properties
Let’s extend the previous case configuration. Consider the extension of the configuration shown below.
Based on the type, additional properties can be varied. Consider two types of DB supported: Oracle and MySQL.
Oracle-specific properties if type
is oracle
or MySQL-specific properties if type
is mysql
.
db:
hostname:
port:
type: # type of db
.
# DB specific properties
.
----
# in case of mysql
db:
hostname: localhost
port: 9991
type: mysql
dumpQueriesOnException: true
callableStmtCacheSize: 10
---
# in case of oracle
db:
hostname: localhost
port: 9992
type: oracle
batchPerformanceWorkaround:
UML Class diagram for a possible solution:
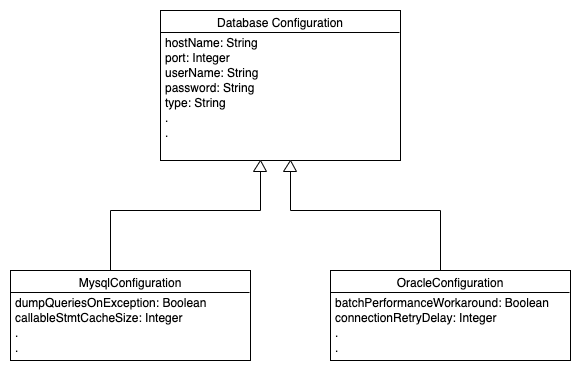
The IDE is unable to distinguish Oracle properties with MySQL Properties in the case of Spring Configuration Metadata. The generated Spring metadata JSON file does not describe or command the conditional nature of properties. Also, the Spring Configuration metadata specification does not specify any conditional construct.

The IDE is able to intercept the conditional properties in the case of the YAML Schema.

Wrapping Up
The Spring Configuration metadata programmer is equivalently as good as the YAML Schema when it comes to static configurable properties. Moreover, it is automatically generated; however, this is supported by the paid edition of IntelliJ IDEA.
YAML provides excellent conditional schema support, which makes it powerful; however, writing such a complex schema is not an easy task.
The YAML schema is not limited to Spring configuration files application.yml
or bootstrap.yml
but any YAML file. It enables a broad scope of applicability; it is a better choice for applications requiring external configuration.
So, Which Is Better?
Spring developers should include the conditional properties construct, or they can adopt a YAML/ JSON schema, which will be the future standard and be understood by a broader audience (even with different language backgrounds!). Also, it would simplify the IDE implementation.
References
- Spring Configuration Meta https://docs.spring.io/spring-boot/docs/2.1.7.RELEASE/reference/html/configuration-metadata.html
- JSON Schemahttps://json-schema.org/understanding-json-schema/
- Polymorphic Spring Configuration https://stackoverflow.com/questions/53962547/polymorphic-configuration-properties-in-spring-boot
- Oracle Driver https://docs.oracle.com/cd/E13222_01/wls/docs81/jdbc_drivers/oracle.html
Further Reading
Opinions expressed by DZone contributors are their own.
Comments