Steps
At the most basic level, Pipelines are made up of multiple steps that allow you to build, test, and deploy applications. Think of a "step" like a single command which performs a single action. When a step succeeds it moves onto the next step. steps are declared in their own section to differentiate them from configuration settings. A steps section may only be contained within a stage.
Shell Steps
Jenkins is a distributed system meant to work across multiple nodes and executors allowing Jenkins to scale the number of Pipelines being run simultaneously and to orchestrate tasks on nodes with different operating systems, tools, environments, etc. Most Pipelines work best by running native CLI commands on different executors. This allows users to automate tasks run on their local machines by copying the commands or scripts directly into Pipeline.
Pipeline supports sh for Linux and macOS, and either bat or powershell on Windows.
Timeouts, Retries and Other Wrappers
There are some powerful steps that "wrap" other steps which can easily solve problems like retrying (retry) steps until successful or exiting if a step takes too long (timeout).
Wrappers may contain multiple steps or may be recursive and contain other wrappers. We can compose these steps together. For example, if we wanted to retry our deployment five times, but never want to spend more than 3 minutes in total before failing the stage:
Stages
A stage in a Pipeline is a collection of related steps that share a common execution environment. Each stage must be named and must contain a steps section. All stages are declared in the stages section of your Pipeline.
When
A stage may be optionally skipped in a Pipeline based on criteria defined in the when section of the stage. branch and environment are supported keywords while expression can be utilized to evaluate any Groovy expression with a Boolean return.
Multiple conditions may be combined in when by using anyOf, allOf or not to create complex skip conditions.
Parallel Stages
To maximize efficiency of your Pipeline some stages can be run in parallel if they do not depend on each other. Tests are a good example of stages that can run in parallel.
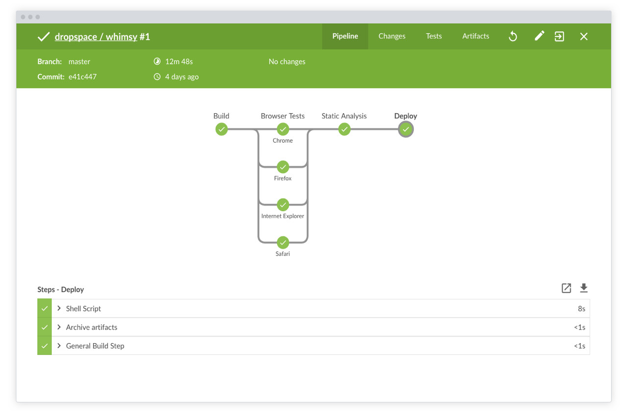
Stage settings
environment, post, agent, and tools may be optionally defined in a stage. These settings are defined in detail below: