A developer specifies JSF components in JSF pages, combining JSF component tags with HTML and CSS for styling. Components are linked with managed beans—Java classes that contain presentation logic and connect to business logic and persistence backends.
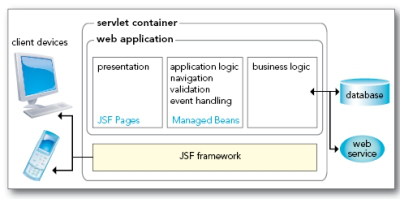
In JSF 2.0, it is recommended that you use the facelets format for your pages:
<?xml version=”1.0” encoding=”UTF-8”?>
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.0 Strict//EN”
“http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd”>
<html xmlns=”http://www.w3.org/1999/xhtml”
xmlns:f=”http://java.sun.com/jsf/core”
xmlns:h=”http://java.sun.com/jsf/html”
xmlns:ui=”http://java.sun.com/jsf/facelets”>
<h:head>...</h:head>
<h:body>
<h:form>
...
</h:form>
</h:body>
</html>
These common tasks give you a crash course into using JSF.
Text Field

page.xhtml
<h:inputText value=”#{bean1.luckyNumber}”>
WEB-INF/classes/com/corejsf/SampleBean.java
@ManagedBean(name=”bean1”)
@SessionScoped
public class SampleBean {
public int getLuckyNumber() { ... }
public void setLuckyNumber(int value) { ... }
...
}
Button

page.xhtml
<h:commandButton value=”press me” action=”#{bean1.login}”/>
WEB-INF/classes/com/corejsf/SampleBean.java
@ManagedBean(name=”bean1”)
@SessionScoped
public class SampleBean {
public String login() {
if (...) return “success”; else return “error”;
}
...
}
The outcomes success and error can be mapped to pages in faces-config.xml. if no mapping is specified, the page /success.xhtml or /error.xhtml is displayed.
Radio Buttons

page.xhtml
<h:selectOneRadio value=”#{form.condiment}>
<f:selectItems value=”#{form.items}”/>
</h:selectOneRadio>
WEB-INF/classes/com/corejsf/SampleBean.java
public class SampleBean {
private static Map<String, Object> items;
static {
items = new LinkedHashMap<String, Object>();
items.put(“Cheese”, 1); // label, value
items.put(“Pickle”, 2);
...
}
public Map<String, Object> getItems() { return items; }
public int getCondiment() { ... }
public void setCondiment(int value) { ... }
...
}
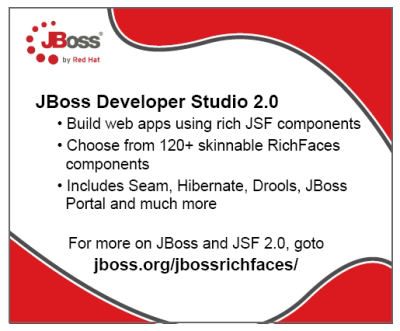
Validation and Conversion
Page-level validation and conversion:
<h:inputText value=”#{bean1.amount}” required=”true”>
<f:validateDoubleRange maximum=”1000”/>
</h:inputText>
<h:outputText value=”#{bean1.amount}”>
<f:convertNumber type=”currency”/>
</h:outputText>
The number is displayed with currency symbol and group separator: $1,000.00
Using the Bean Validation Framework (JSR 303) 2.0
public class SampleBean {
@Max(1000) private BigDecimal amount;
}
Error Messages

<h:outputText value=”Amount”/>
<h:inputText id=”amount” label=”Amount” value=”#{payment.amount}”/>
<h:message for=”amount”/>
Resources and Styles
page.xhtml
<h:outputStylesheet library=”css” name=”styles.css” target=”head”/>
...
<h:outputText value=”#{msgs.goodbye}!” styleClass=”greeting”>
faces-config.xml
<application>
<resource-bundle>
<base-name>com.corejsf.messages</base-name>
<var>msgs</var>
</resource-bundle>
</application>
WEB-INF/classes/com/corejsf/messages.properties
goodbye=Goodbye
WEB-INF/classes/com/corejsf/messages_de.properties
goodbye=Auf Wiedersehen
resources/css/styles.css
.greeting {
font-style: italic;
font-size: 1.5em;
color: #eee;
}
Table with links
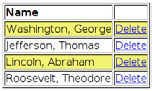
<h:dataTable value=”#{bean1.entries}” var=”row” styleClass=”table”
rowClasses=”even,odd”>
lt;h:column>
<f:facet name=”header”>
<h:outputText value=”Name”/>
</f:facet>
<h:outputText value=”#{row.name}”/>
</h:column>
<h:column>
<h:commandLink value=”Delete” action=”#{bean1.deleteAction}”
immediate=”true”>
<f:setPropertyActionListener target=”#{bean1.idToDelete}”
value=”#{row.id}”/>
</h:commandLink>
</h:column>
</h:dataTable>
WEB-INF/classes/com/corejsf/SampleBean.java
public class SampleBean {
private int idToDelete;
public void setIdToDelete(int value) { idToDelete = value; }
public String deleteAction() {
// delete the entry whose id is idToDelete
return null;
}
public List<Entry> getEntries() { ... }
...
}
Ajax 2.0
<h:commandButton value=”Update”>
<f:ajax execute=”
</h:commandButton>
{{ parent.title || parent.header.title}}
{{ parent.tldr }}
{{ parent.linkDescription }}
{{ parent.urlSource.name }}