How to Test GET Requests With Playwright Java for API Testing
This tutorial teaches how to test GET requests using the Playwright Java framework for API testing, with examples of fetching data from an API and handling errors.
Join the DZone community and get the full member experience.
Join For FreePlaywright is a popular open-source test automation framework created by Microsoft. It enables developers and test automation engineers to automate web applications on different browsers and platforms. It supports major programming languages, including JavaScript, TypeScript, Java, C#, and Python. It also uses API Automation Testing, which is seen as a major benefit over other web automation frameworks.
In this tutorial blog, we will learn to use Playwright with Java and test GET API requests in automation testing.
Getting Started
I recommend you check out my previous blog to familiarize yourself with creating a project, setting up, and configuring Playwright with Java for API automation testing.
Application Under Test
We will use free-to-use RESTful e-commerce APIs from demo E-Commerce applications that are available over GitHub.
The project can be set up locally using NodeJS or Docker. It has multiple APIs related to order management, such as creating, updating, fetching, and deleting orders.
What Is a GET Request?
A GET request retrieves data from the specified source. It is the most commonly used HTTP method and does not require sending a request body to fetch data from the source. However, filter parameters called Query Params and Path Params may be required to be provided as a header to filter and fetch the specified record.
The GET request generally returns the Status Code 200 along with the data that was requested. Depending upon the Content-Type
the data may be received in JSON or XML format.
In the following example of RESTful e-commerce APIs, there are two API endpoints that we will test in this tutorial blog.
1. GET /getAllOrders
Endpoint
This gets all the available orders in the system.
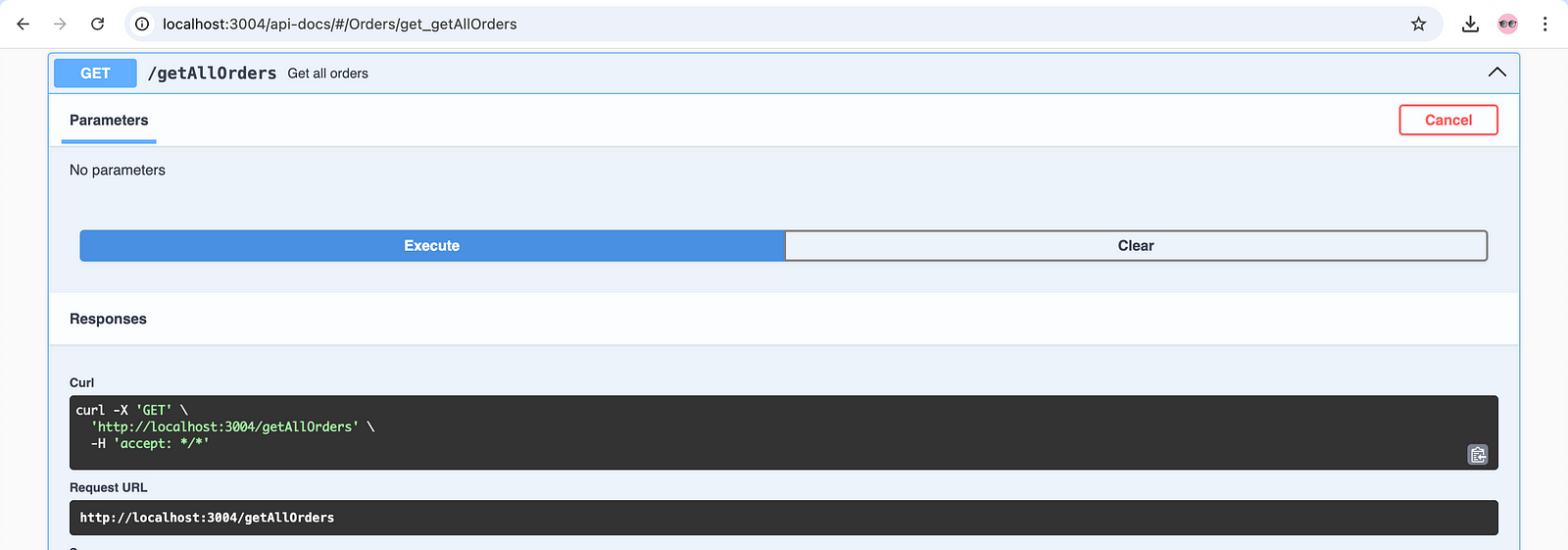
This API returns Status Code 200 in the response with the order data in JSON format.
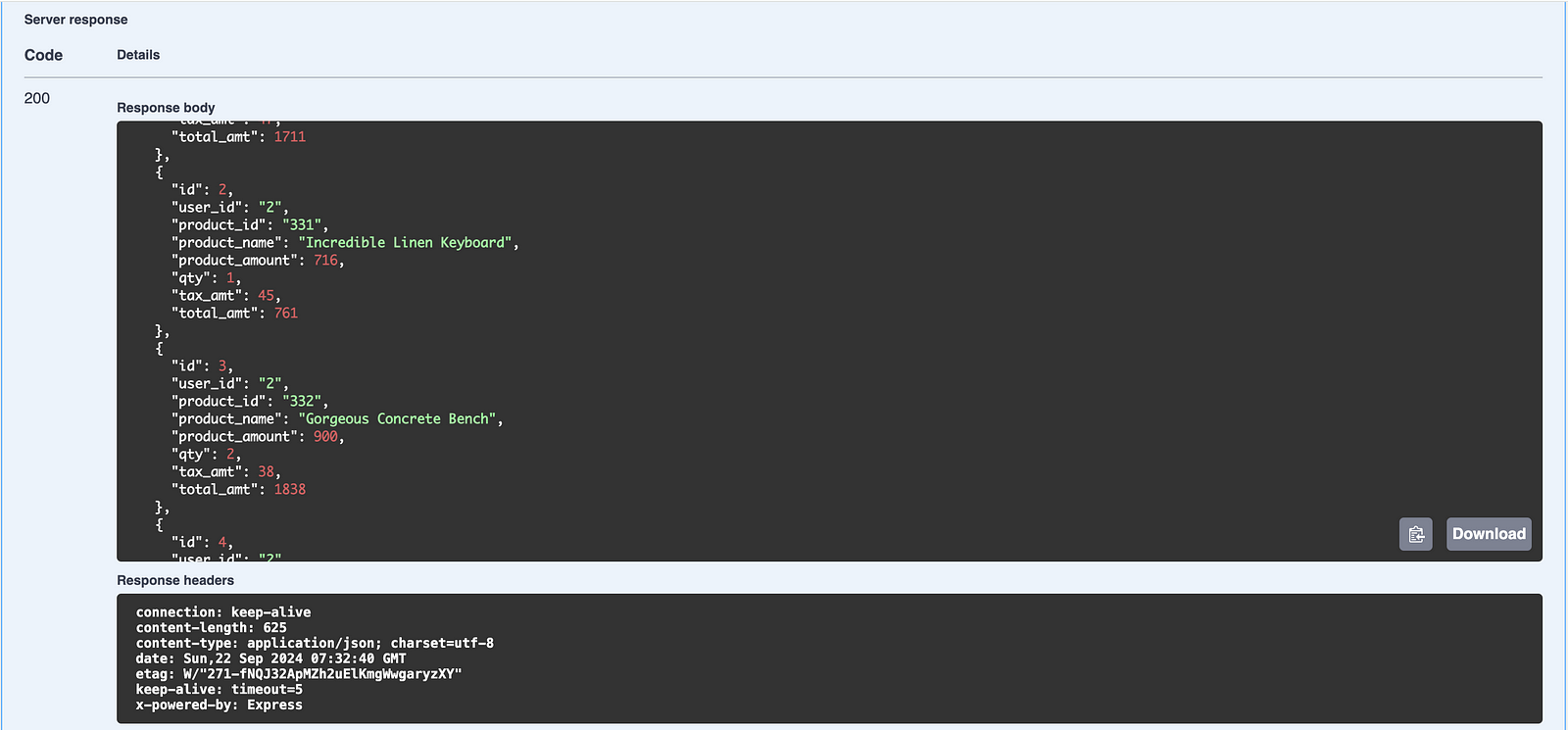
If no orders are found, it returns Status Code 404 with the message, “No order found!!”
2. GET /getOrder
Endpoint
This retrieves the order based on the filter applied on either order_id
, user_id
, product_id
, or all of them.
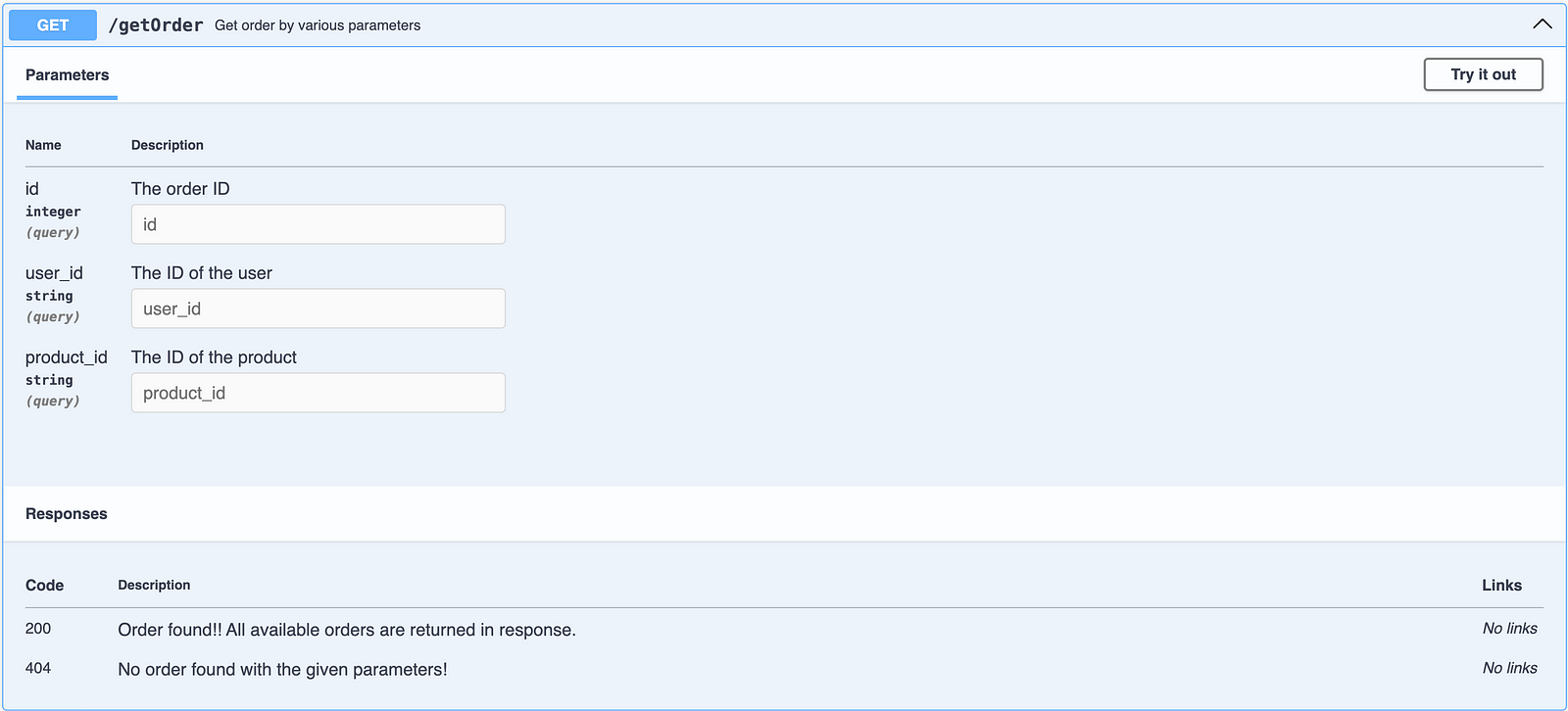
It will return Status Code 200 with the filtered order in the response; otherwise, it will return Status Code 404 with the message, “No order found with the given parameters!”
After learning about the APIs under test, let’s learn how to test GET APIs using Playwright Java for API automation testing.
How to Test GET APIs Using Playwright Java
Let’s first discuss the test scenarios we will use to write the API tests using Playwright Java.
Test Scenario 1: Getting All the Orders
- Start the service for a RESTful e-commerce app.
- Hit the GET
/getAllOrders
endpoint to retrieve all the available orders in the system. - Check that the Status Code 200 is received in the response.
- Check that the correct data is retrieved in the response.
Note: We had already generated four orders using the POST request previously in the POST request tutorial. We will be retrieving the same order of data using this GET /getAllOrders
endpoint.
Test Implementation
Let’s create a new test method testShouldGetAllOrders()
in the existing test class HappyPathTests
.
Just to reiterate, two test classes, namely, HappyPathTests and SadPathTests have been created previously , also a BaseTest class has been added to configure and set up Playwright.
@Test
public void testShouldGetAllOrders() {
final APIResponse response = this.request.get("/getAllOrders");
final JSONObject responseObject = new JSONObject(response.text());
final JSONArray ordersArray = responseObject.getJSONArray("orders");
assertEquals(response.status(), 200);
assertEquals(responseObject.get("message"), "Orders fetched successfully!");
assertEquals(this.orderList.get(0).getUserId(), ordersArray.getJSONObject(0).get("user_id"));
assertEquals(this.orderList.get(0).getProductId(), ordersArray.getJSONObject(0).get("product_id"));
assertEquals(this.orderList.get(0).getTotalAmt(), ordersArray.getJSONObject(0).get("total_amt"));
}
The result of the API request will be stored in the response
variable that is defined for the type APIResponse
The GET
request will be sent using the this.request.get("/getAllOrders")
which will handle the GET request and fetch the data from the system.
The response will be received using response.text()
method and will be stored in the responseObject
variable that is of JSONObject
type (it is a class of org.json
library).
The following is the response received in JSON format for the GET /getAllOrders
endpoint:
{
"message": "Orders fetched successfully!",
"orders": [
{
"id": 1,
"user_id": "3",
"product_id": "331",
"product_name": "Aerodynamic Cotton Bench",
"product_amount": 416,
"qty": 4,
"tax_amt": 47,
"total_amt": 1711
},
{
"id": 2,
"user_id": "2",
"product_id": "331",
"product_name": "Incredible Linen Keyboard",
"product_amount": 716,
"qty": 1,
"tax_amt": 45,
"total_amt": 761
},
{
"id": 3,
"user_id": "2",
"product_id": "332",
"product_name": "Gorgeous Concrete Bench",
"product_amount": 900,
"qty": 2,
"tax_amt": 38,
"total_amt": 1838
},
{
"id": 4,
"user_id": "2",
"product_id": "331",
"product_name": "Ergonomic Concrete Gloves",
"product_amount": 584,
"qty": 2,
"tax_amt": 21,
"total_amt": 1189
}
]
}
As the data retrieved in the response is a JSON Object, the values are stored using the JSONObject
class.
final JSONObject responseObject = new JSONObject(response.text());
However, the order details are fetched as JSON Array; hence, they are stored in the ordersArray
variable.
final JSONArray ordersArray = responseObject.getJSONArray("orders");
Finally, the assertions will be performed to check that the GET /getAllOrders
API returns Status Code 200. The response.status()
method will return the Status Code.
The responseObject
variable holds the response received, so we will get the message
field from this JSON object. We can then check that it has the text, “Orders fetched successfully!”
In this POST Request tutorial, we used the OrderData
class to generate the order data on run time using DataFaker and Lombok library. In the tests, we declared List<OrderData>
with a variable named orderList
. The same object will be called in the assertion to check the data integrity of the order details fetched.
The following is the JSON Array that is fetched in the response:
"orders": [
{
"id": 1,
"user_id": "3",
"product_id": "331",
"product_name": "Aerodynamic Cotton Bench",
"product_amount": 416,
"qty": 4,
"tax_amt": 47,
"total_amt": 1711
},
{
"id": 2,
"user_id": "2",
"product_id": "331",
"product_name": "Incredible Linen Keyboard",
"product_amount": 716,
"qty": 1,
"tax_amt": 45,
"total_amt": 761
},
{
"id": 3,
"user_id": "2",
"product_id": "332",
"product_name": "Gorgeous Concrete Bench",
"product_amount": 900,
"qty": 2,
"tax_amt": 38,
"total_amt": 1838
},
{
"id": 4,
"user_id": "2",
"product_id": "331",
"product_name": "Ergonomic Concrete Gloves",
"product_amount": 584,
"qty": 2,
"tax_amt": 21,
"total_amt": 1189
}
]
We would be checking the first object from the order array.
{
"id": 1,
"user_id": "3",
"product_id": "331",
"product_name": "Aerodynamic Cotton Bench",
"product_amount": 416,
"qty": 4,
"tax_amt": 47,
"total_amt": 1711
}
The following lines of code will fetch the user_id
, product_id
and total_amt
from the object and, accordingly, use the data that was supplied using the orderList
variable (data generated on runtime using Datafaker and Lombok):
assertEquals(this.orderList.get(0).getUserId(), ordersArray.getJSONObject(0).get("user_id"));
assertEquals(this.orderList.get(0).getProductId(), ordersArray.getJSONObject(0).get("product_id"));
assertEquals(this.orderList.get(0).getTotalAmt(), ordersArray.getJSONObject(0).get("total_amt"));
Test Execution
First, we need to ensure that the RESTful e-commerce app is up and working. Follow these steps to start the app on your local machine.
We will be executing the POST request (generated using the tutorial on testing POST Requests using Playwright Java) and the GET request using the following testng.xml
file. The POST request will generate the orders, and GET will retrieve the orders and perform the required assertion.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Restful ECommerce Test Suite">
<test name="Testing Happy Path Scenarios of Restful E-Commerce APIs">
<classes>
<class name="io.github.mfaisalkhatri.api.restfulecommerce.HappyPathTests">
<methods>
<include name="testShouldCreateNewOrders"/>
<include name="testShouldGetAllOrders"/>
</methods>
</class>
</classes>
</test>
</suite>
The following screenshot from IntelliJ IDE shows that the test execution was successful, and orders were fetched successfully:
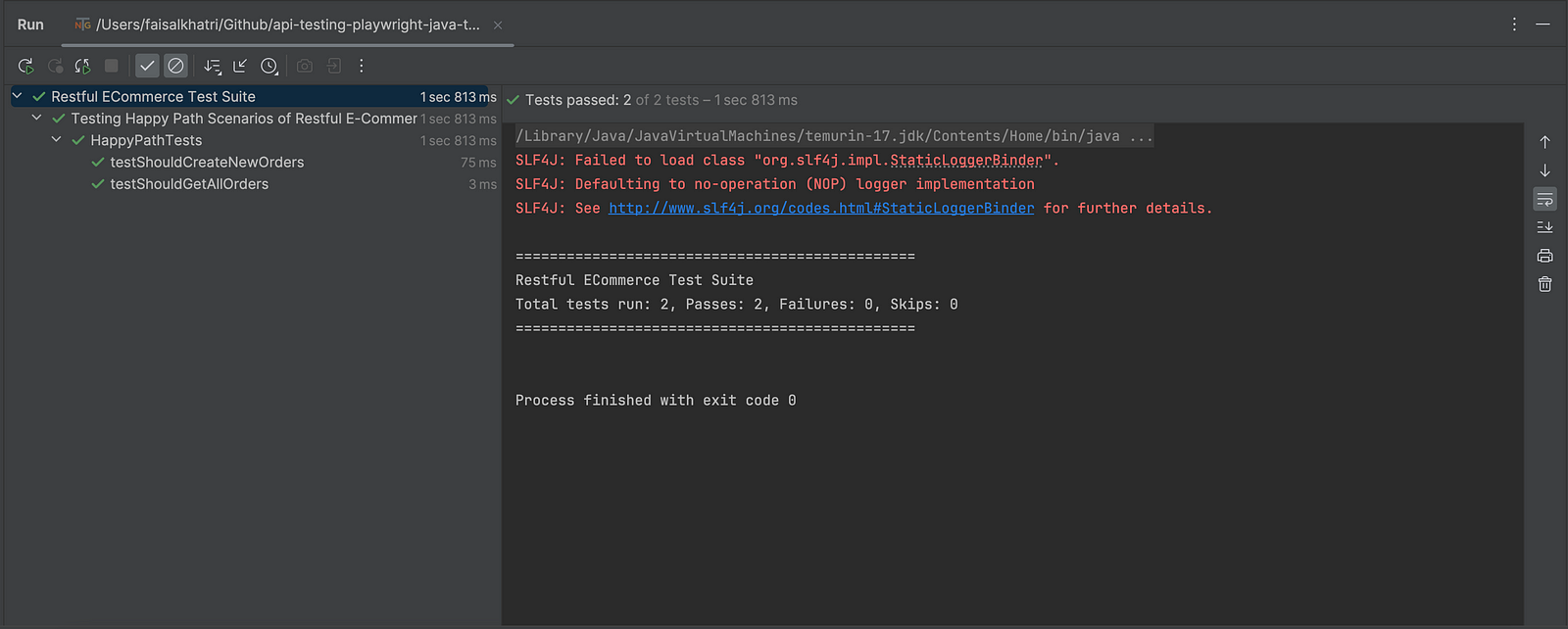
Test Scenario 2: Get Order Filtering on order_id
- Start the service for a RESTful e-commerce app.
- Hit the GET
/getOrder
endpoint to retrieve order filtering onorder_id
using a Query parameter. - Check that the Status Code 200 is received in the response.
- Check that the correct data is retrieved in the response.
Test Implementation
Let’s create a new test method testShouldGetOrderUsingOrderId()
to implement this test scenario.
@Test
public void testShouldGetOrderUsingOrderId() {
final int orderId = 1;
final APIResponse response = this.request.get("/getOrder", RequestOptions.create().setQueryParam("id", orderId));
final JSONObject responseObject = new JSONObject(response.text());
final JSONArray ordersArray = responseObject.getJSONArray("orders");
assertEquals(response.status(), 200);
assertEquals(ordersArray.getJSONObject(0).get("id"), orderId);
assertEquals(responseObject.get("message"), "Order found!!");
}
Since we already have some orders created in the system. We would be fetching the order with order_id = 1
.
For retrieving the order with order_id
filter we need to provide the order_id
as a query parameter. This can be achieved using the following line of code:

The query parameter can be passed using the setQueryParam()
method from the RequestOptions
interface available in Playwright. The parameter name and its required value need to be supplied as a parameter to the setQueryParam()
method.
Next, we need to parse the response object and get it in the JSON object format. The response.text()
returns the response of the API in String format. This String will be converted to the parsed JSON object using the JSONObject
class of org.json
library.
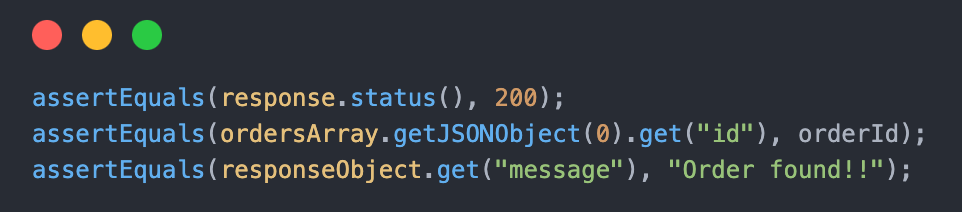
The parsed JSON object will be further used to perform assertions. There won’t be any check related to data integrity here; we are checking that the response object has the same order_id
that we gave in the request, and the Status Code 200 was returned well in the response.
One step ahead, we are also checking that themessage
field in the response has the text “Order found!!”
Test Execution
Let’s add this method to the same testng.xml
file, restart the RESTful e-commerce app so we have a fresh app with no data, and run all the tests again.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Restful ECommerce Test Suite">
<test name="Testing Happy Path Scenarios of Restful E-Commerce APIs">
<classes>
<class name="io.github.mfaisalkhatri.api.restfulecommerce.HappyPathTests">
<methods>
<include name="testShouldCreateNewOrders"/>
<include name="testShouldGetAllOrders"/>
<include name="testShouldGetOrderUsingOrderId"/>
</methods>
</class>
</classes>
</test>
</suite>
The following screenshot of the test execution shows that the tests were executed successfully, and orders were fetched as per the scenario:
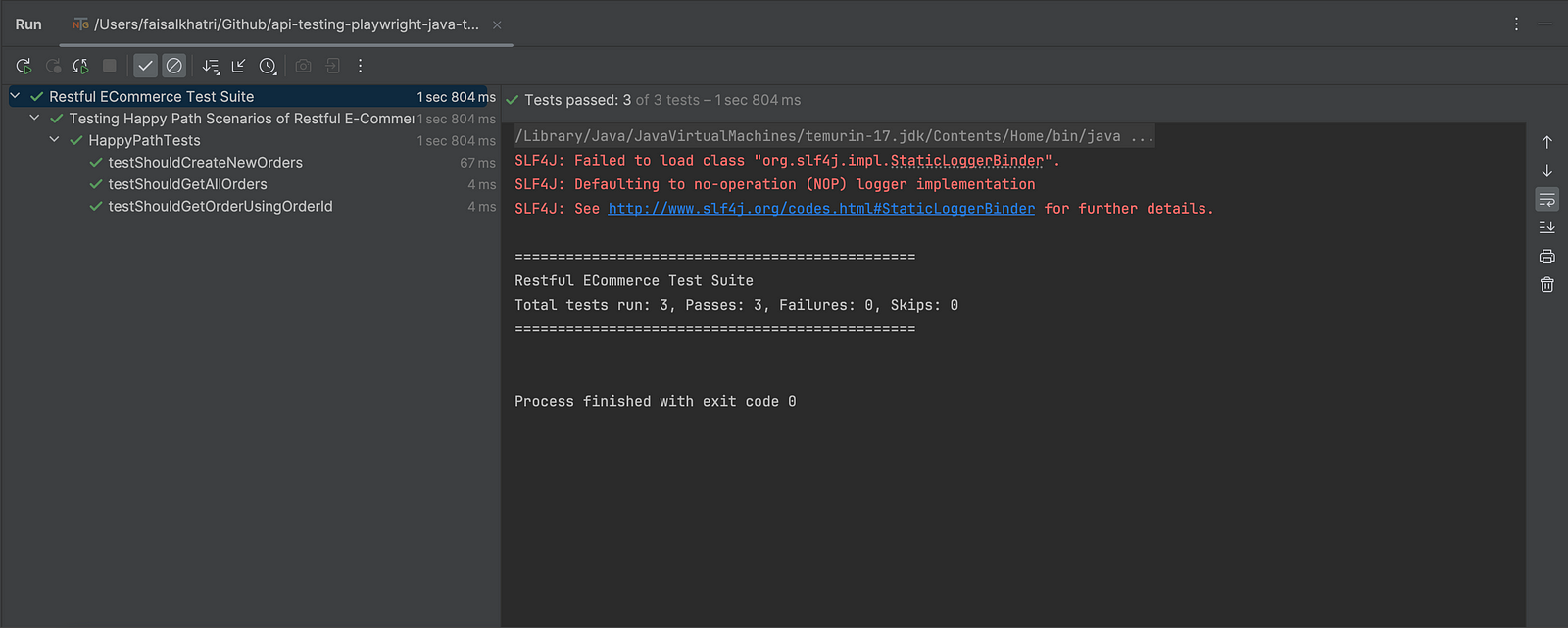
Test Scenario 3: Get Order Filtering on Multiple Query Parameters
- Start the service for a RESTful e-commerce app.
- Hit the GET
/getOrder
endpoint to retrieve order filtering onorder_id
,product_id
anduser_id
query parameters. - Check that the Status Code 200 is received in the response.
- Check that the correct data is retrieved in the response.
Test Implementation
The test implementation of this test scenario is pretty much the same as we did for Test Scenario 2. The only addition here would be to add product_id
and user_id
query parameters to the test method.
A new test method testShouldGetOrdersUsingOrderIdProductIdAndUserId()
is added to existing HappyPathTests
class.
public void testShouldGetOrdersUsingOrderIdProductIdAndUserId() {
final int orderId = 2;
final String productId = "332";
final String userId = "2";
final APIResponse response = this.request.get("/getOrder", RequestOptions.create()
.setQueryParam("id", orderId)
.setQueryParam("product_id", productId)
.setQueryParam("user_id", userId));
final JSONObject responseObject = new JSONObject(response.text());
final JSONArray ordersArray = responseObject.getJSONArray("orders");
assertEquals(response.status(), 200);
assertEquals(responseObject.get("message"), "Order found!!");
assertEquals(ordersArray.getJSONObject(0).get("id"), orderId);
assertEquals(ordersArray.getJSONObject(0).get("product_id"), productId);
assertEquals(ordersArray.getJSONObject(0).get("user_id"), userId);
}
In this test method, the setQueryParam
is called three times in order to pass on the required parameters.
The rest of the implementation related to parsing the response and checking the status code and message remains the same.
Additionally, there are assertions added in this method to check that the order details response received in the response has the given order_id
, product_id
and user_id
. This assertion will ensure that the correct order details were retrieved per the query parameters.
Test Execution
The following test execution result from IntelliJ shows that the order details, as per the query parameters, were fetched successfully:
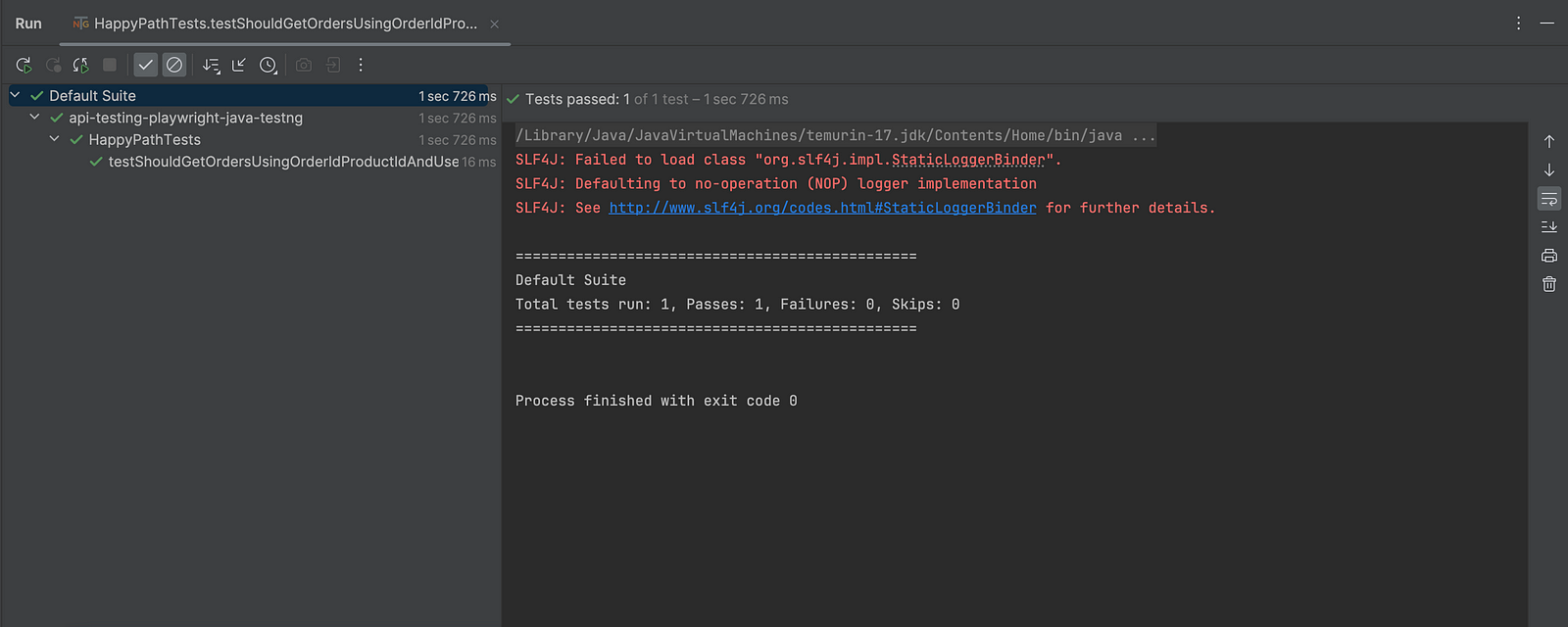
Summary
GET requests fetch the required data from the specified resource and is the most commonly used HTTP method. It generally returns the data in the response with Status Code 200. GET requests can also consider the required filters using query param or path param to query the data.
While testing GET requests, it is important to validate that the data received in the response is as expected. This validation would be for all the data fetched at once or per the query parameters provided.
Playwright exposes the get()
method in the APIRequestContext
interface that could be used to retrieve the data. There are certain Request parameters like headers, query parameters, etc., which are also supported.
I hope this blog provides you with sufficient knowledge to automate GET requests using Playwright Java. Try your hands-on API Automation testing with Playwright.
Published at DZone with permission of Faisal Khatri. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments