Notepad++ with the C# compiler - you can do it
Join the DZone community and get the full member experience.
Join For Free
if you ever decided to to work a bit outside of visual studio and configure a plain text editor to work with the c# compiler, you most likely know that it's not so straightforward as it might seem. at first, i thought that it would be a relatively easy task to add a specific compiler reference to
notepad++
, but it needed some additionalwork, as notepad++ has no specific wizard or dialog to directly configure a custom compiler. however, there is a
run
box (found in the run menu) that can help you solve many problems:
there are two ways to use a compiler - either use the
...
button and specify the path to the compiler, and then save it, or you could configure the path environment variable and use the compiler directly from the command line by calling the executable name.
i am going to start with the second option - set the specific path variable and then use
csc
(the c# compiler) from inside notepad++. the reason behind this is that sometimes you will want to compile from the console (without an ide or text editor) and this will make the task a lot easier. not that you will encounter this scenario very often, but it is always a great plus to know how it can be automated - for example, you might want to have some compilation scripts later.
before going any further, i tried to see how to set all correct path references so that i will make the system console .net-enabled (excluding the option of running the visual studio command prompt bundled with the vs release you have). in the
program files
folder (or the host folder where you installed visual studio) there is the
visual studio x.0
folder (replace x with the most recent version of visual studio installed on the machine). in the
common7
folder you will find the
tools
folder and this is the final destination for now.
run the command prompt (
winkey+r
,
cmd
) and simply drag the
vsvars32.bat
file in the console. then, just press enter and the path variable will be automatically set so that you can run .net tools from the command line. technically, this would be the same procedure as if you would run the visual studio command prompt, but now you know how this process actually starts.
note:
don't run the bat file directly from windows explorer, as it will have no effect - it only sets the environment variables for the current session.
once you have the path variable set, you need to get the newly-set values to make them permanent, and not only for the current session. let's do it the simple way - just save the console output for the path in a text file. to do this, just type in the console (make sure you are in the folder you want the file to be saved into):
path > mypath.txt
close the command prompt and go to the text file you just saved. copy everything after
path=
. go to the
system properties
dialog (
winkey+pause break
) and find the
advanced sytem settings
(it's called so in windows vista and 7 - not entirely sure about previous versions). in the
advanced
tab is the
environment
button and this is exactly what's needed.
click it and you will see that there are two lists of environment variables in the new dialog. the first one is for the current user and the second one is for system-wide environment variables. select
path
from
system variables
and edit it. completely replace the existing string with the one you just copied and click ok. to test the new settings, try opening the command prompt and simply type csc. the output should look like this:
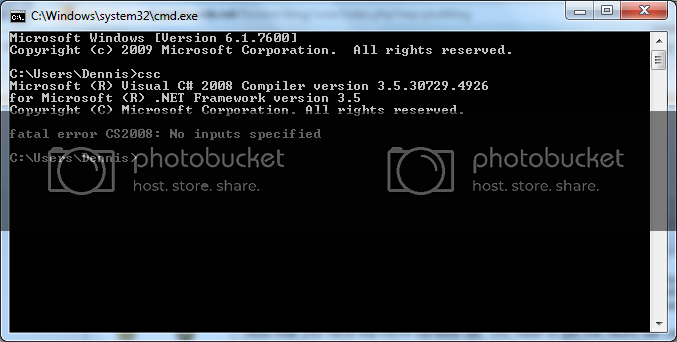
awesome! so the compiler can be accesed without explicitly specifying the path. let's go to notepad++ and configure it to work with csc . type a simple c# program in the editor:
using system;namespace consoleapplication{class program{static void main(string[] args){console.writeline("sample");console.readline();}}}
click on
run > run...
. you will now need to enter the program to be used to compile the file. there are several internal environment variables that you can use the supply the file name to the compiler, since passing the direct path to one file is not exactly what i wanted - after all, i want to re-use the capability. suppose that you saved your file (and it should be saved before compiling) as
c:\documents\myfiles\sample.cs
. there are a couple of internal variables (specific to notepad++) that can be used to reference the file you are currently working with:
full_current_path
- is the full path to file - c:\documents\myfiles\sample.cs
current_directory
- just the directory where the file is located - c:\documents\myfiles
file_name
- the file name only - sample.cs
name_part
- the part that only shows the name of the file without the extension - sample
ext_part
- the file extension - cs
you need to use the full path to compile the file, so here goes the actual command:
csc.exe $(full_current_path)
why type the
exe
extension? because there is a csc folder in the system folder, and typing only csc will result in opening the folder instead of launching the compiler.
the above command will create an executable with the same name as the source file in the user folder.
if you want to specify another folder for the executable to be built in, use the
out
parameter:
csc.exe /out:"d:\temporary\myfile.exe" $(full_current_path)
once the command is working you can save it in from the
run
dialog for easy re-use.
note: you can customize the command line parameters for the compiler as you want - after all, you might want to compile a library and reference additional assemblies.
Opinions expressed by DZone contributors are their own.
Comments