Setting Title and Caption With ExifTool
Set photo titles and captions with ExifTool.
Join the DZone community and get the full member experience.
Join For Free
I recently needed to change the title and caption of some photos, so I turned to ExifTool, as it's the Swiss Army knife of image metadata.
It's a lovely tool with many options, so I wrote a script to make it easy, and while I was there, I used read to prompt me for the info to set. This is the script:
~/bin/exif-set:
#!/usr/bin/env bash
# determine current values
IMAGE_TITLE=$(exiftool -s3 -iptc:ObjectName "$1")
IMAGE_DESCRIPTION=$(exiftool -s3 -iptc:Caption-Abstract "$1")
IMAGE_KEYWORDS=$(exiftool -s -s -s -iptc:Keywords "$1")
# Ask user for new values
read -e -p "Title [$IMAGE_TITLE]: " NEW_TITLE
read -e -p "Description [$IMAGE_DESCRIPTION]: " NEW_DESCRIPTION
read -e -p "Keywords [$IMAGE_KEYWORDS]: " NEW_KEYWORDS
# set to defaults if we got a blank result
NEW_TITLE="${NEW_TITLE:-${IMAGE_TITLE}}"
NEW_DESCRIPTION="${NEW_DESCRIPTION:-${IMAGE_DESCRIPTION}}"
NEW_KEYWORDS="${NEW_KEYWORDS:-${IMAGE_KEYWORDS}}"
# Update
exiftool \
-overwrite_original \
-iptc:ObjectName="$NEW_TITLE" \
-iptc:Caption-Abstract="$NEW_DESCRIPTION" \
-iptc:Keywords="$NEW_KEYWORDS" \
"$1"
# display what we've set
exiftool -f \
-iptc:ObjectName \
-iptc:Caption-Abstract \
-iptc:Keywords \
"$1"
There are a few interesting things that I'd like to point out. Firstly, we used ExifTool to find the current values of the three properties I cared about. For example, for the title:
IMAGE_TITLE=$(exiftool -s3 -iptc:ObjectName "$1")
The IPTC property names are a little opaque, which is partly why I wanted a script, as I'm highly unlikely to remember that the title is the ObjectName property. The -s3 flag returns just the text of the property with no label, as that's what I want to store in the variable. It's a nice feature; I appreciate that I don't have to pipe through sed or awk to extract just the part I want.
Prompting for a new value is done using read. I decided that I also wanted a blank entry (i.e. just pressing return) to indicate that I wanted to keep the current value. This is done using the ":-" parameter substitution feature of bash:
read -e -p "Title [$IMAGE_TITLE]: " NEW_TITLE
NEW_TITLE="${NEW_TITLE:-${IMAGE_TITLE}}"
We now have values to set, which are either new values typed in via read or the original values, so I can just write then back to the file:
exiftool \
-overwrite_original \
-iptc:ObjectName="$NEW_TITLE" \
-iptc:Caption-Abstract="$NEW_DESCRIPTION" \
-iptc:Keywords="$NEW_KEYWORDS" \
"$1"
Exiftool knows you want to write metadata because of the "=" assignment of a value to the property name. By default, it will create a copy of the original file before modifying it. I don't need this copy, so overwrite_original prevents a proliferation of files that I would then have to delete.
That's It
I now have a handy command line tool that allows me to change the title, caption and keywords of a photo easily. Job done!
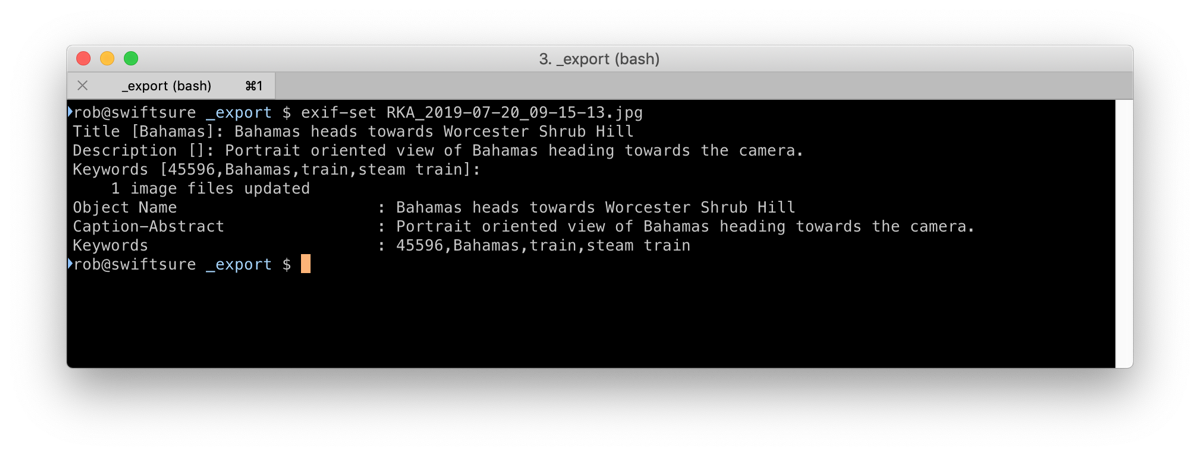
Published at DZone with permission of Rob Allen, DZone MVB. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments