a4j:ajax
Upgrades the standard f:ajax tag/behavior with more features.
<h:inputText value=”#{bean.input}”>
<a4j:ajax execute=”#{bean.process}” render=”#{bean.update}”/>
</h:inputText>
<h:panelGrid id=”list1”>...</h:panelGrid>
Execute & Render EL Resolution
JSF 2.0 determines the values for execute and render attributes when the current view is rendered. In the example above if #{bean.update} changes on the server the older value will be used. RichFaces processes attribute values on the server side so you will always be using the most current value.
Addition Common Enhancements
All RichFaces components that fire Ajax requests share the features above, and all of the ones from below:
Attribute |
Description |
limitRender |
Turns off all auto-rendered panels (see Render Options section). |
bypassUpdates |
When set to true, skips Update Model and Invoke Application phases. Useful for form validation requests. |
on begin |
JavaScript code to be invoked before Ajax request is sent. |
onbeforedomupdate |
JavaScript code to be invoked after response is received but before Ajax updates happen. |
incomplete |
JavaScript code to be invoked after Ajax request processing is complete. |
status |
Name of status component to show during Ajax request. |
a4j:commandButton, a4j:commandLink
Similar to standard h:commandButton and h:commandLink tags but with Ajax behavior built-in.
<a4j:commandButton value=”Add”
action=”#{bean.add}” render=”cities”/>
<h:panelGrid id=”cities”>...</h:panelGrid>

Default execute value for both controls is @form.
a4j:poll
Periodically fires an Ajax request based on polling interval defined via interval attribute and can be enabled/disabled via enabled attribute (true|false). For example, in the following code snippet, an Ajax request will be sent every 2 seconds and render the time component:
<a4j:poll interval=”2000” enabled=”#{bean.active}”
action=”#{bean.count}” render=”time”/>
<h:outputText id=”time” value=”#{bean.time}”/>
a4j:jsFunction
Allows the sending of an Ajax request from any JavaScript function.
<a4j:jsFunction name=”set drink” render=”drink”>
<a4j:param name=”param1” assignTo=”#{bean.drink}”/>
</a4j:jsFunction>
...
<td onmouseover=”setdrink(‘Espresso’)”
onmouseout=”setdrink(‘’)”>Espresso</td>
<h:outputText id=”drink” value=”I like #{bean.drink}” />
When the mouse hovers or leaves a drink, the setdrink() JavaScript function is called. The function is defined by an a4j:jsFunction tag which sets up standard Ajax call. You can also invoke an action. The drink parameter is passed to the server via a4j:param tag.
a4j:status
Displays Ajax request status. The component can display content based on Ajax start, stop, and error conditions. Status can be defined in the following three ways: status per view, status per form and named statuses. The following example shows named status:
<a4j:status name=”ajaxStatus”>
<f:facet name=”start”>
<h:graphicImage value=”/ajax.gif” />
</f:facet>
</a4j:status>
<a4j:commandButton value=”Save” status=”ajaxStatus”/>
All RichFaces controls which fire an Ajax request have status attribute available.
a4j:repeat
Works just like ui:repeat but also supports partial table update (see Data Iteration):
<ul>
<a4j:repeat value=”#{bean.list}” var=”city”>
<li>#{city.name}</li>
</a4j:repeat>
</ul>
a4j:push
“Push” server-side events to client using Comet or WebSockets. This is implemented using Atmosphere (http://atmosphere.java.net), and uses JMS for message processing (such as JBoss’s HornetQ - http://www.jboss.org/hornetq). This provides excellent integration with EE containers, and advanced messaging services.
The <a4j:push> tag allows you to define named topics for messages delivery and actions to perform:
<a4j:push address=”topic@chat”
ondataavailable=”alert(event.rf.data)” />
Server side messages are published and topics are created/configured using a class similar to this:
@PostConstruct
public void init() {
topicsContext = TopicsContext.lookup();
}
private void say(String message) throws
MessageException {
TopicKey key = new TopicKey(“chat”,”topic”);
topicsContext.publish(key, message);
}
private void onStart() {
topicsContext.getOrCreateTopic(new
TopicKey(“chat”));
}
For more details on usage and setup, including examples please see the RichFaces Component Guide (http://docs.jboss.org/richfaces/latest_4_0_X/Component_Reference/en-US/html/).
a4j:param
Works like <f:param> also allows client side parameters and will assign a value automatically to a bean property set in assignTo :
<a4j:commandButton value=”Select”>
<a4j:param value=”#{rowIndex}”
assignTo=”#{bean.row}”/>
</a4j:commandButton>
a4j:log
Client-side Ajax log and debugging.
<a4j:log/>
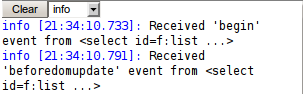
a4j:region
Provides declarative definition of components to be executed during Ajax request instead of using component ids. The following example wouldn’t work without a4j:region as no execute ids are defined on the a4j:poll which defaults to execute=”@this”:
<a4j:region>
<a4j:poll interval=”10000”/>
<h:inputText value=”#{bean.name}”/>
<h:inputText value=”#{bean.email}”/>
</a4j:region>
If components are wrapped inside a4j:region without execute id defined, then the default value is execute=”@region”. You can also explicitly set execute=”@region”.