Create Charts in ReactJS Using Chart.js
Join the DZone community and get the full member experience.
Join For FreeIn this article, we will learn how to create charts in a React application using the Chart.js library. Chart.js is an open-source JavaScript library for creating charts. Chart.js makes it easier to draw different types of charts like line, bar, doughnut, and area charts. In this article, we will create a line chart, bar chart, pie chart, and polar area using React and Web API. See chart.js' docs for more information.
Prerequisites
- Basic knowledge of ReactJS and Web API.
- Visual Studio and Visual Studio Code can be installed.
- SQL Server Management Studio.
This article covers:
- Creating a database and table.
- Creating an ASP.NET Web API Project.
- Creating a ReactJS Project.
- Installing the Chart.js library
- Installing Bootstrap and Axios.
- Adding routes in ReactJS.
Create a Table in the Database
Open SQL Server Management Studio, create a database named, "DemoTest," and in this database, create a table. Give that table a name like "Ipltopscorer."
CREATE TABLE [dbo].[Ipltopscorer](
[id] [int] IDENTITY(1,1) NOT NULL,
[Playername] [varchar](50) NULL,
[Runscore] [int] NULL,
CONSTRAINT [PK_Ipltopscorer] PRIMARY KEY CLUSTERED
(
[id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
Add some demo data into this table.
You may also like: Cube.js: Ultimate Guide to the Open-Source Dashboard Framework.
Create a New Asp.net Web API Project
Open Visual Studio and create a new project.
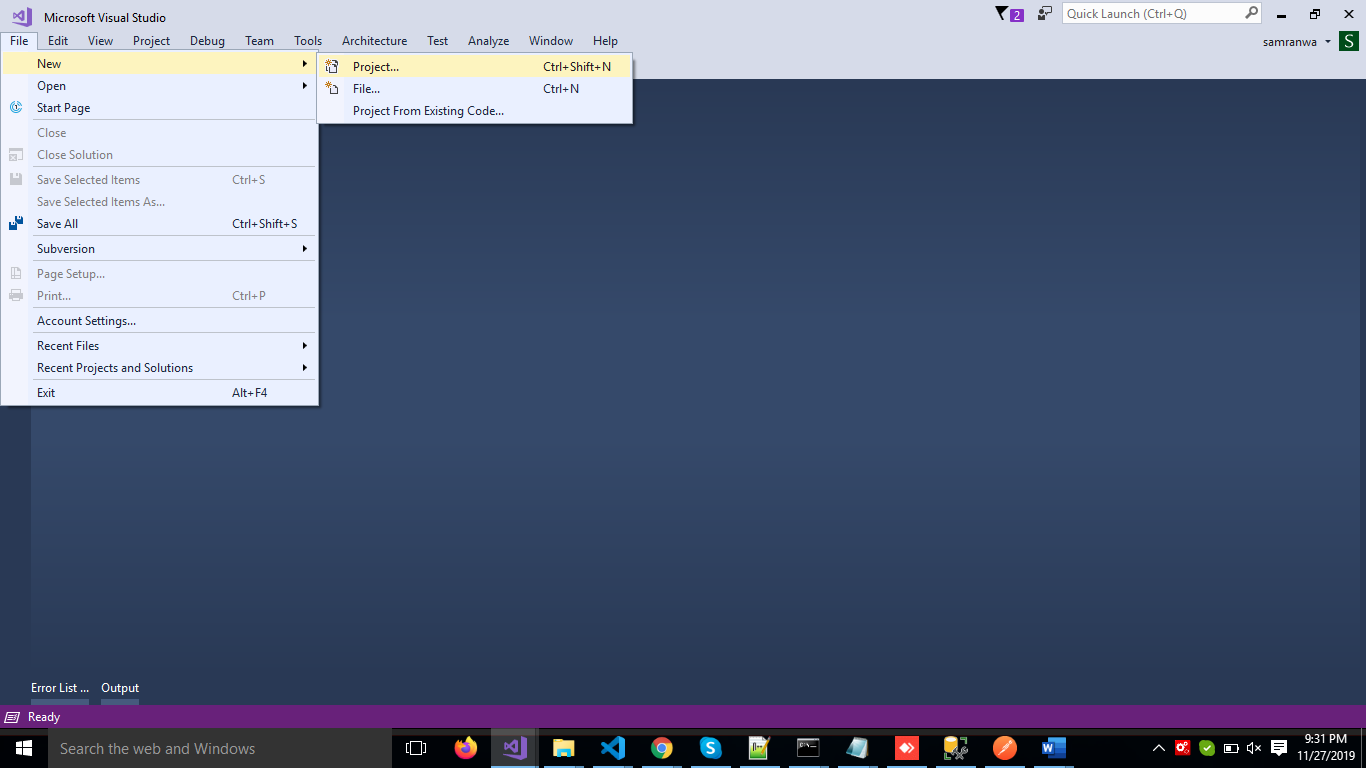
Change the name to "ChartDemo" and click on Ok.
Select Web API as its template.
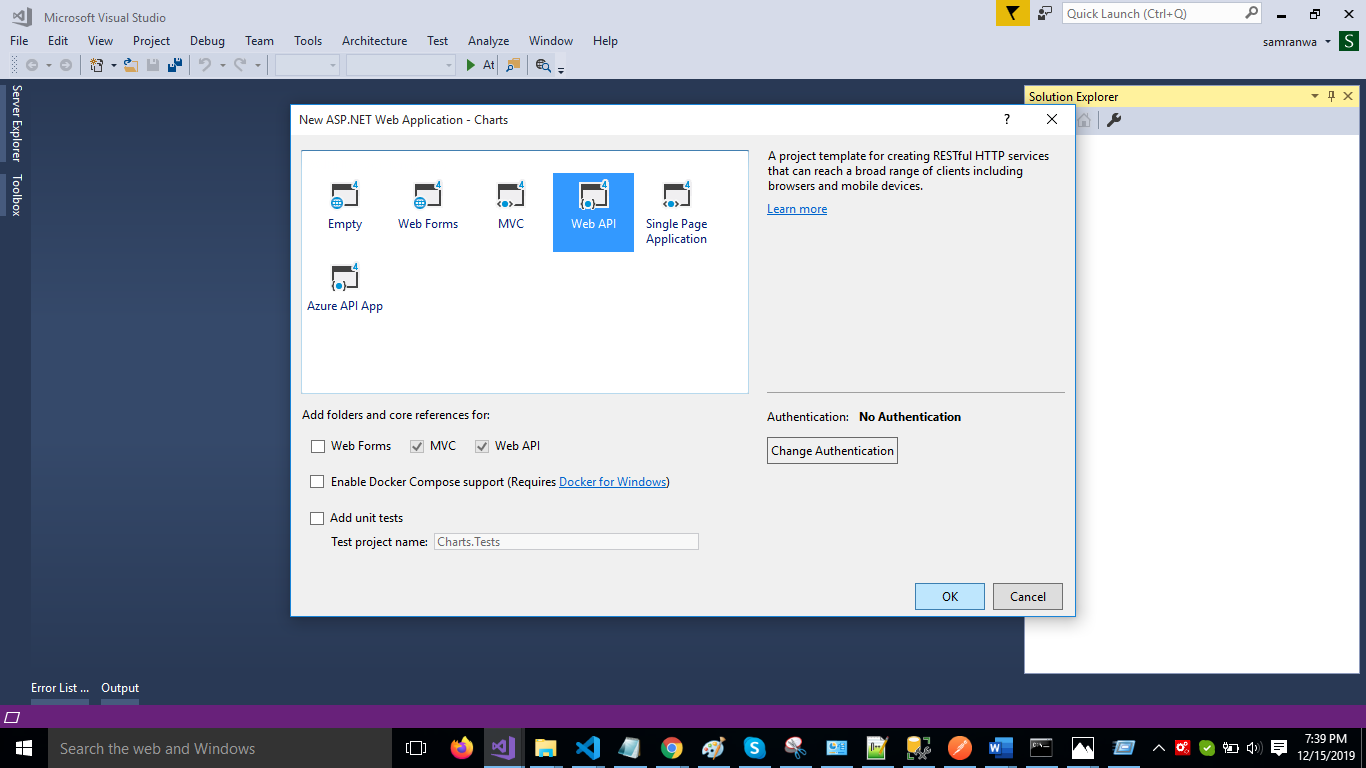
Right-click the Models folder from Solution Explorer and go to Add >> New Item >> data.
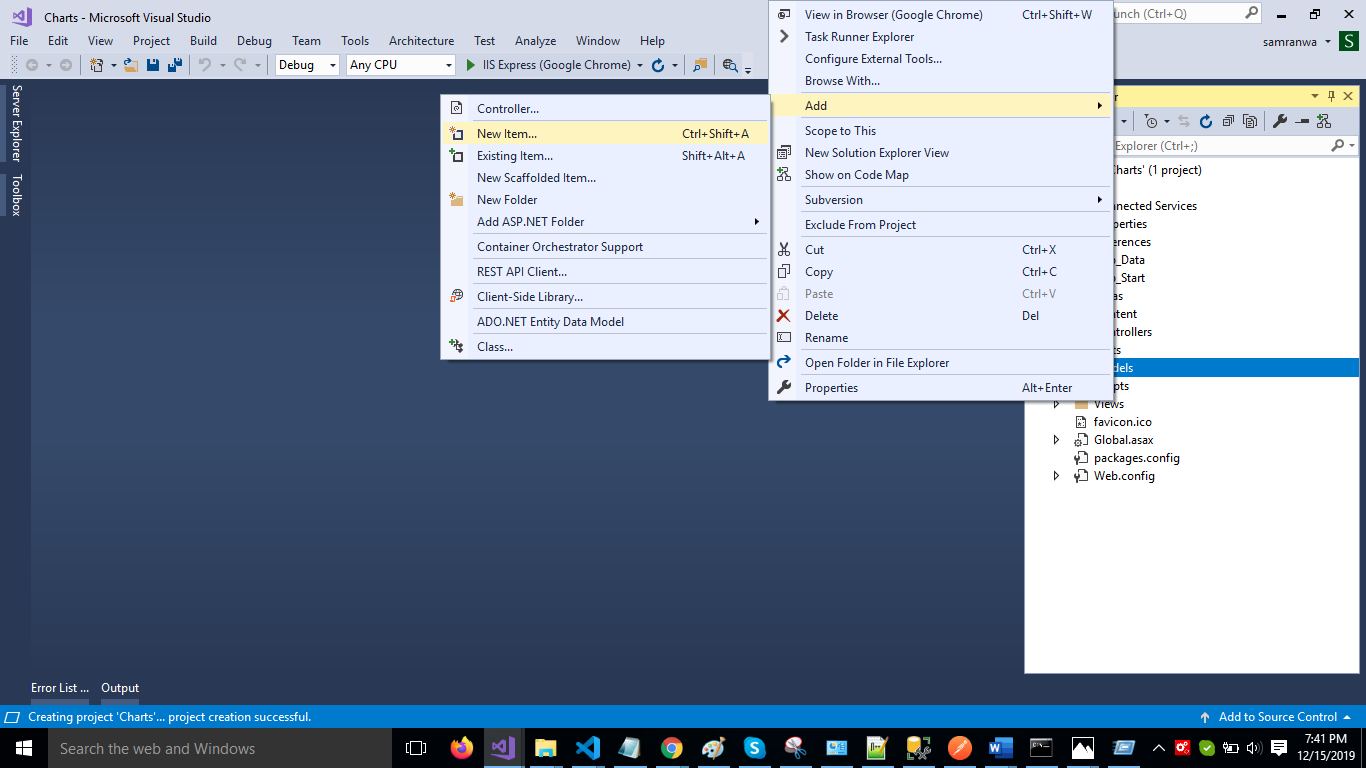
Click on the ADO.NET Entity Data Model option and click Add.
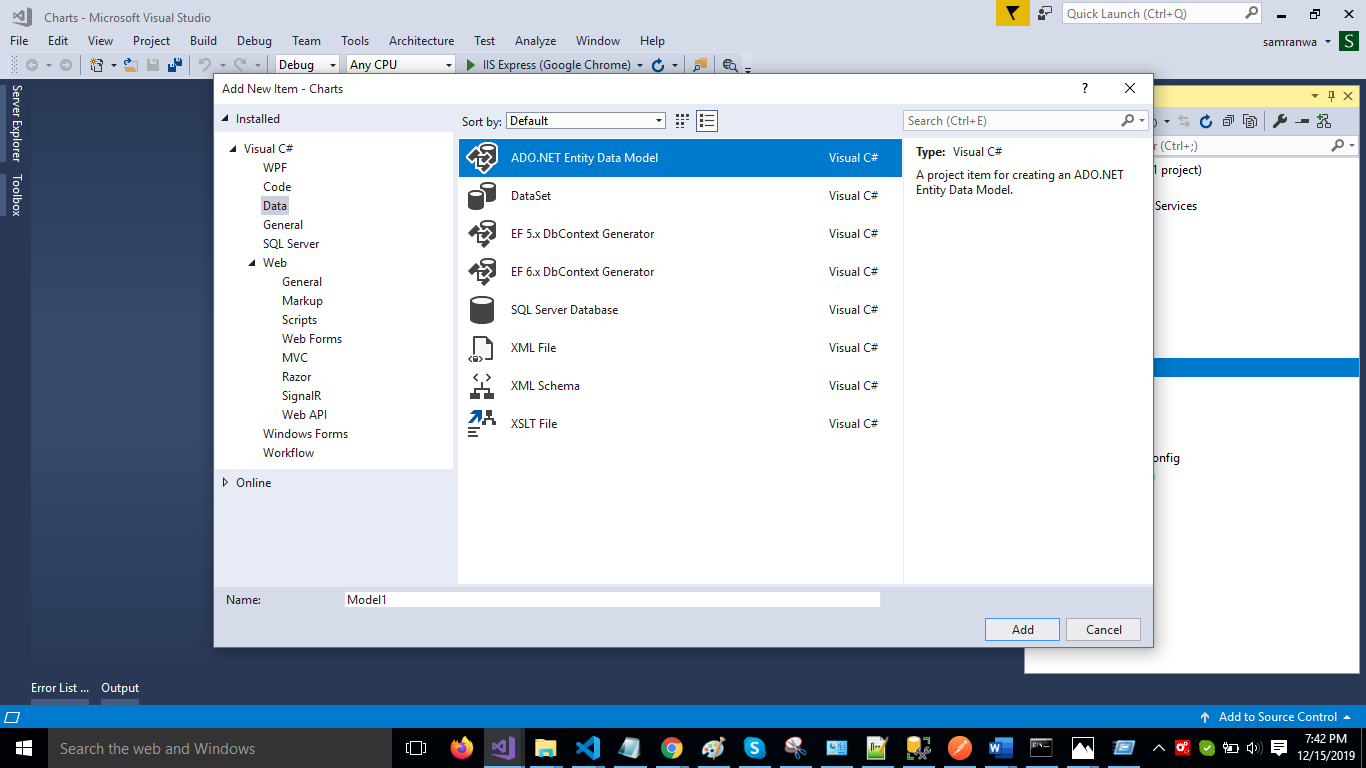
Select EF Designer from the database and click the Next button.
Add the connection properties and select the database name on the next page and click OK.
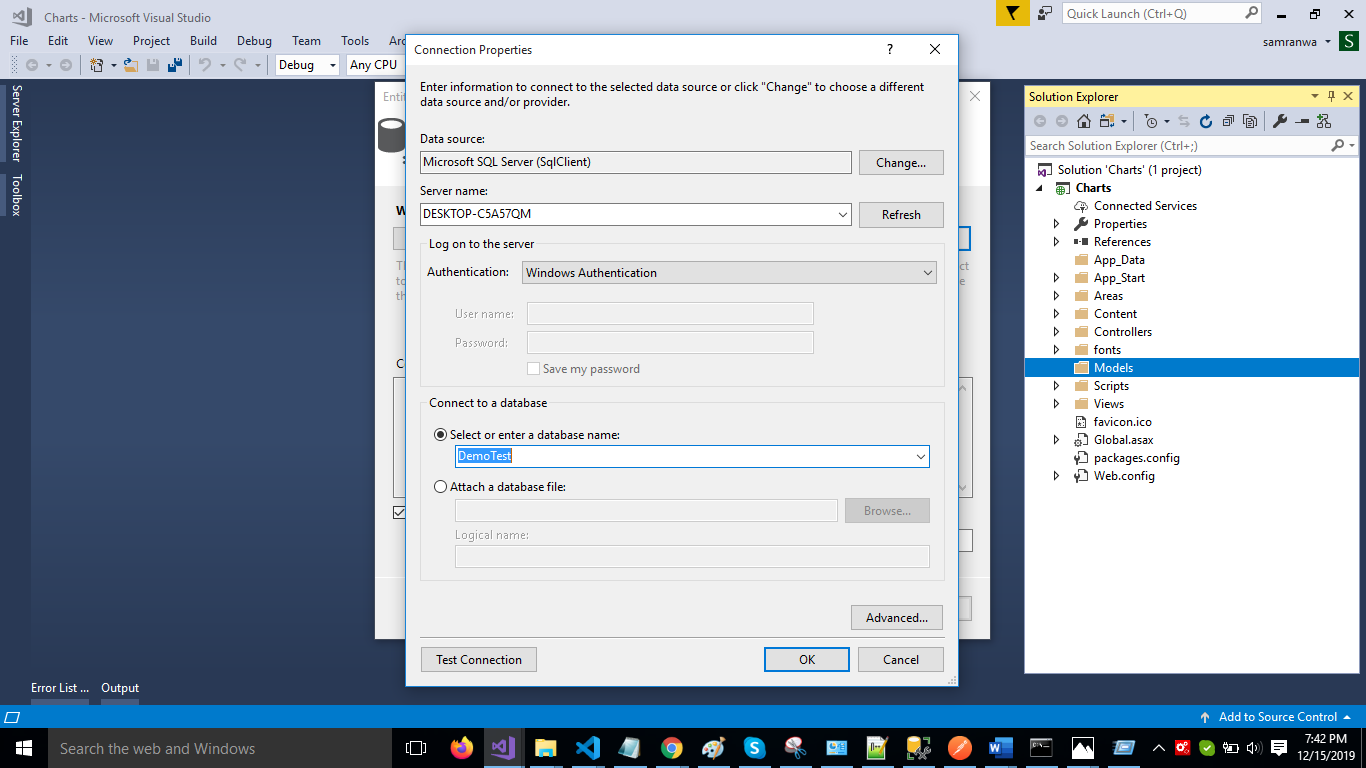
Check the Table checkbox. The internal options will be selected by default. Now, click the Finish button.
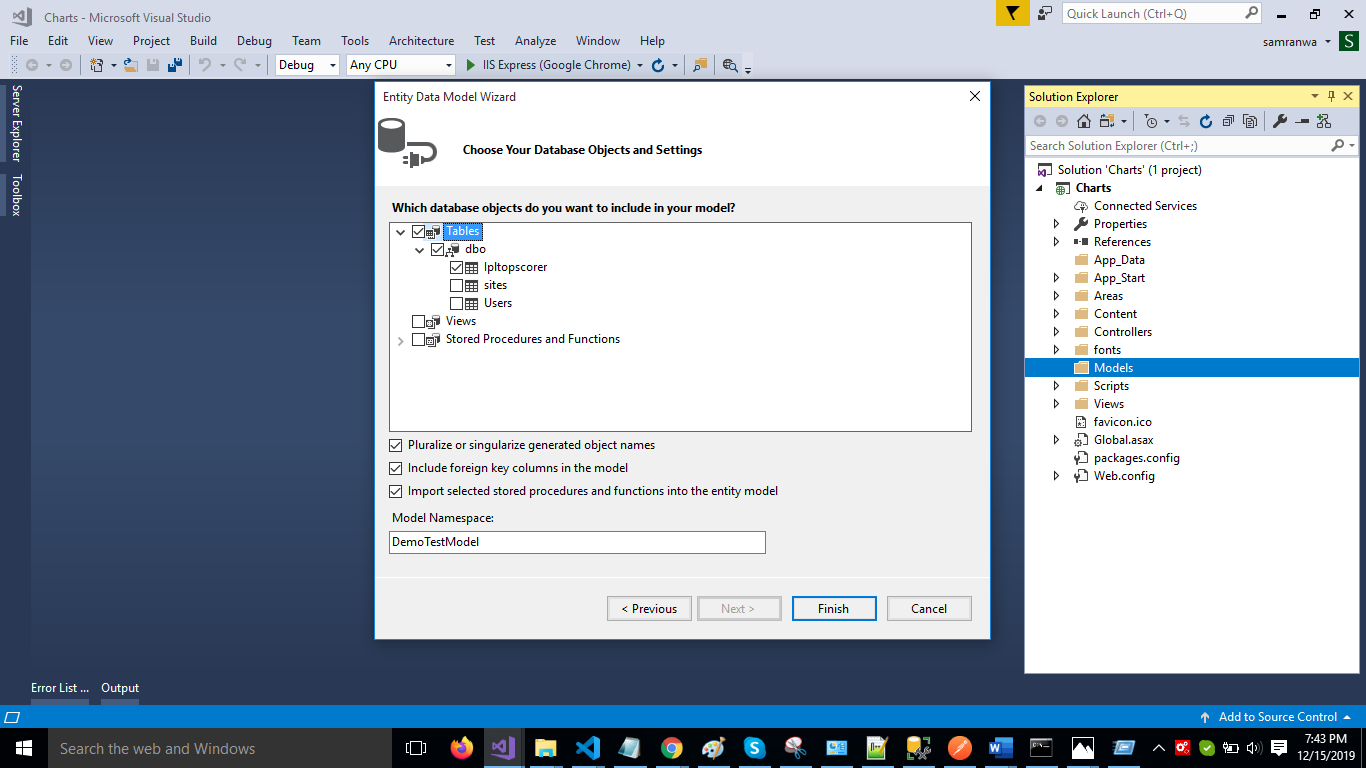
Our data model is successfully created now. Right-click on the Controllers folder and add a new controller. Name it "Chartcontroller."
Add the following namespace in the Charts Controller.
xxxxxxxxxx
using ChartDemo.Models;
Add a new method to fetch data from the database.
xxxxxxxxxx
public object Getrecord()
{
DemoTestEntities DB = new DemoTestEntities();
return Json(DB.Ipltopscorers.ToList());
}
Complete the Chartcontroller code.
xxxxxxxxxx
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using ChartDemo.Models;
namespace ChartDemo.Controllers
{
[RoutePrefix("API/Charts")]
public class ChartController : ApiController
{
[Route("Getrecord")]
[HttpGet]
public object Getrecord()
{
DemoTestEntities DB = new DemoTestEntities();
return Json(DB.Ipltopscorers.ToList());
}
}
}
Now, let's enable Cors. Go to Tools, open NuGet Package Manager, search for Cors, and install the Microsoft.Asp.Net.WebApi.Cors package.
xxxxxxxxxx
EnableCorsAttribute cors = new EnableCorsAttribute("*", "*", "*");
config.EnableCors(cors);
Create a ReactJS Project
Now create a new React.js project by using the following command:
xxxxxxxxxx
npx create-react-app reactcharts
Open the newly created project in Visual Studio code.
Install the Chart.js Library
Now, install the Chart.js library in the React project by typing the following command:
xxxxxxxxxx
npm install react-chartjs-2 chart.js
Install Bootstrap in this project by using the following command:
xxxxxxxxxx
npm install --save bootstrap
Now, open the index.js file and import Bootstrap.
xxxxxxxxxx
import 'bootstrap/dist/css/bootstrap.min.css';
Now, install the Axios library by using the following command. Learn more about Axios fromt their docs.
-
Shell
xxxxxxxxxx
11npm install --save axios
Now go to src folder and add 5 new components.
- Linechart.js.
- Barchart.js.
- Doughnut.js.
- Piechart.js.
- Polararea.js.
Now, open Linechart.js, import Line chart from the Chart.js library, and add the following code.
xxxxxxxxxx
import React, { Component } from 'react'
import axios from 'axios';
import { Line } from 'react-chartjs-2';
export class Linecharts extends Component {
constructor(props) {
super(props);
this.state = { Data: {} };
}
componentDidMount() {
axios.get(`http://localhost:61022/Api/Charts/Getrecord`)
.then(res => {
console.log(res);
const ipl = res.data;
let playername = [];
let runscore = [];
ipl.forEach(record => {
playername.push(record.Playername);
runscore.push(record.Runscore);
});
this.setState({
Data: {
labels: playername,
datasets: [
{
label: 'IPL 2018/2019 Top Run Scorer',
data: runscore,
backgroundColor: [
"#3cb371",
"#0000FF",
"#9966FF",
"#4C4CFF",
"#00FFFF",
"#f990a7",
"#aad2ed",
"#FF00FF",
"Blue",
"Red"
]
}
]
}
});
})
}
render() {
return (
<div>
<Line
data={this.state.Data}
options={{ maintainAspectRatio: false }} />
</div>
)
}
}
export default Linecharts
Now, open Barchart.js and add following code:
xxxxxxxxxx
import React, { Component } from 'react'
import axios from 'axios';
import { Bar } from 'react-chartjs-2';
export class Barchart extends Component {
constructor(props) {
super(props);
this.state = { Data: {} };
}
componentDidMount() {
axios.get(`http://localhost:61022/Api/Charts/Getrecord`)
.then(res => {
console.log(res);
const ipl = res.data;
let playername = [];
let runscore = [];
ipl.forEach(record => {
playername.push(record.Playername);
runscore.push(record.Runscore);
});
this.setState({
Data: {
labels: playername,
datasets: [
{
label: 'IPL 2018/2019 Top Run Scorer',
data: runscore,
backgroundColor: [
"#3cb371",
"#0000FF",
"#9966FF",
"#4C4CFF",
"#00FFFF",
"#f990a7",
"#aad2ed",
"#FF00FF",
"Blue",
"Red"
]
}
]
}
});
})
}
render() {
return (
<div>
<Bar data={this.state.Data}
options={{ maintainAspectRatio: false }} ></Bar>
</div>
)
}
}
export default Barchart
Now, open Doughnut.js and add the following code:
xxxxxxxxxx
import React, { Component } from 'react'
import axios from 'axios';
import {Doughnut} from 'react-chartjs-2';
export class Doughnutchart extends Component {
render() {
return (
<div>
</div>
)
} constructor(props) {
super(props);
this.state = { Data: {} };
}
componentDidMount() {
axios.get(`http://localhost:61022/Api/Charts/Getrecord`)
.then(res => {
console.log(res);
const ipl = res.data;
let playername = [];
let runscore = [];
ipl.forEach(record => {
playername.push(record.Playername);
runscore.push(record.Runscore);
});
this.setState({
Data: {
labels: playername,
datasets: [
{
label: 'IPL 2018/2019 Top Run Scorer',
data: runscore,
backgroundColor: [
"#3cb371",
"#0000FF",
"#9966FF",
"#4C4CFF",
"#00FFFF",
"#f990a7",
"#aad2ed",
"#FF00FF",
"Blue",
"Red"
]
}
]
}
});
})
}
render() {
return (
<div>
<Doughnut data={this.state.Data}
options={{ maintainAspectRatio: false }} ></Doughnut>
</div>
)
}
}
export default Doughnutchart
Now, open Piechart.js and add the following code:
xxxxxxxxxx
import React, { Component } from 'react'
import axios from 'axios';
import { Pie } from 'react-chartjs-2';
export class Piechart extends Component {
constructor(props) {
super(props);
this.state = { Data: {} };
}
componentDidMount() {
axios.get(`http://localhost:61022/Api/Charts/Getrecord`)
.then(res => {
console.log(res);
const ipl = res.data;
let playername = [];
let runscore = [];
ipl.forEach(record => {
playername.push(record.Playername);
runscore.push(record.Runscore);
});
this.setState({
Data: {
labels: playername,
datasets: [
{
label: 'IPL 2018/2019 Top Run Scorer',
data: runscore,
backgroundColor: [
"#3cb371",
"#0000FF",
"#9966FF",
"#4C4CFF",
"#00FFFF",
"#f990a7",
"#aad2ed",
"#FF00FF",
"Blue",
"Red"
]
}
]
}
});
})
}
render() {
return (
<div>
<Pie
data={this.state.Data}
options={{ maintainAspectRatio: false }} />
</div>
)
}
}
export default Piechart
Now, open Polararea.js and add the following code:
xxxxxxxxxx
import React, { Component } from 'react'
import { Polar } from 'react-chartjs-2';
import axios from 'axios';
export class Polararea extends Component {
constructor(props) {
super(props);
this.state = { Data: {} };
}
componentDidMount() {
axios.get(`http://localhost:61022/Api/Charts/Getrecord`)
.then(res => {
console.log(res);
const ipl = res.data;
let playername = [];
let runscore = [];
ipl.forEach(record => {
playername.push(record.Playername);
runscore.push(record.Runscore);
});
this.setState({
Data: {
labels: playername,
datasets: [
{
label: 'IPL 2018/2019 Top Run Scorer',
data: runscore,
backgroundColor: [
"#3cb371",
"#0000FF",
"#9966FF",
"#4C4CFF",
"#00FFFF",
"#f990a7",
"#aad2ed",
"#FF00FF",
"Blue",
"Red"
]
}
]
}
});
})
}
render() {
return (
<div>
<Polar data={this.state.Data}
options={{ maintainAspectRatio: false }} />
</div>
)
}
}
export default Polararea
Add Routing in ReactJS
Install the react-router-dom package by using the following command:
xxxxxxxxxx
npm install react-router-dom --save
Now, open app.js file and import all five components and the React router component. Add the following code in the app.js file:
xxxxxxxxxx
import React from 'react';
import './App.css';
import Piechart from './piechart'
import Doughnutchart from './Doughnutchart'
import Barchart from './Barchart'
import Linecharts from './linecharts'
import Polararea from './Polararea'
import { BrowserRouter as Router, Switch, Route, Link } from 'react-router-dom';
function App() {
return (
<div className="App">
<Router>
<div>
<div class="row" className="hdr">
<div class="col-sm-12 btn btn-warning">
Charts in ReactJS
</div>
</div>
<div class="row"> >
<div class="col-sm-1">
</div>
<div class="col-sm-2">
<Link to={'/Piechart'} className="nav-link btn btn-info">Piechart</Link>
</div>
<div class="col-sm-2">
<Link to={'/Barchart'} className="nav-link btn btn-info">Bar Chart</Link>
</div>
<div class="col-sm-2">
<Link to={'/Linecharts'} className="nav-link btn btn-info">Line Chart</Link>
</div>
<div class="col-sm-2">
<Link to={'/Doughnut'} className="nav-link btn btn-info">Doughnut Chart</Link>
</div>
<div class="col-sm-2">
<Link to={'/Polararea'} className="nav-link btn btn-info">Polar Chart</Link>
</div>
<div class="col-sm-1">
</div>
</div>
</div>
<div className="container">
<Switch>
<Route exact path='/Piechart' component={Piechart} />
<Route path='/Barchart' component={Barchart} />
<Route path='/Polararea' component={Polararea} />
<Route path='/Doughnut' component={Doughnutchart} />
<Route path='/Linecharts' component={Linecharts} />
</Switch>
</div>
</Router>
</div>
);
}
export default App;
Now, run the project by using npm start
command and check the result. Click on the buttons to see each chart.
Summary
In this article, we learned about Chart.js and how to add it in a ReactJS applications to create charts. In this article, we discussed the line chart, bar chart, pie chart, doughnut chart, and polar area chart. We can also use other Chart libraries in ReactJS to create more visualizations.
Further Reading
Opinions expressed by DZone contributors are their own.
Comments