HashSet vs. TreeSet vs. LinkedHashSet
Join the DZone community and get the full member experience.
Join For Freein a set, there are no duplicate elements. that is one of the major reasons to use a set. there are 3 commonly used implementations of set in java: hashset, treeset and linkedhashset. when and which to use is an important question. in brief, if we want a fast set, we should use hashset; if we need a sorted set, then treeset should be used; if we want a set that can be read by following its insertion order, linkedhashset should be used.
1. set interface
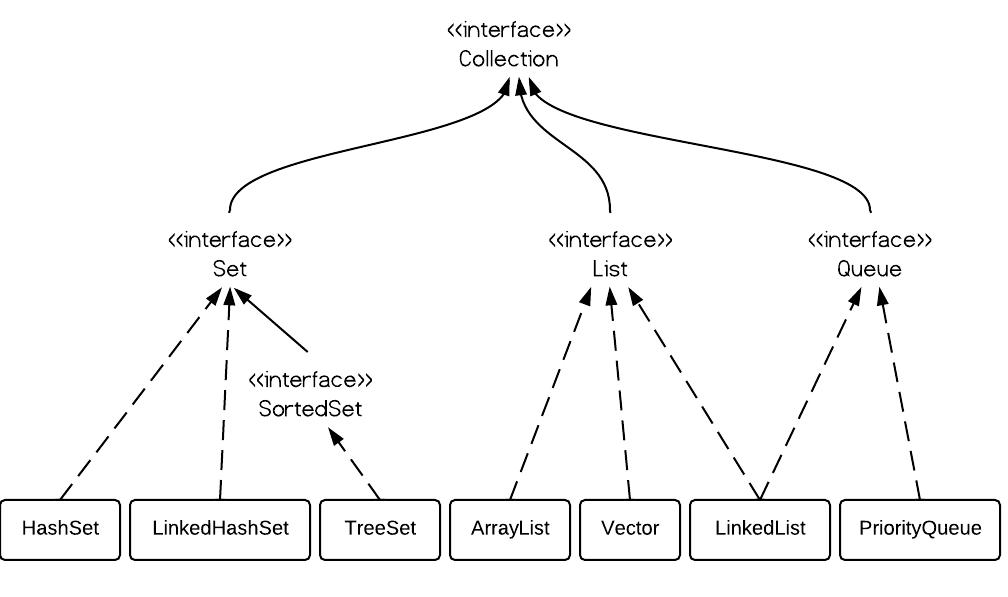
2. hashset vs. treeset vs. linkedhashset
3. treeset example
treeset tree = new treeset();
tree.add(12);
tree.add(63);
tree.add(34);
tree.add(45);
iterator iterator = tree.iterator();
system.out.print("tree set data: ");
while (iterator.hasnext()) {
system.out.print(iterator.next() + " ");
}
output is sorted as follows:
tree set data: 12 34 45 63
now let's define a dog class as follows:
class dog {
int size;
public dog(int s) {
size = s;
}
public string tostring() {
return size + "";
}
}
let's add some dogs to treeset like the following:
import java.util.iterator;
import java.util.treeset;
public class testtreeset {
public static void main(string[] args) {
treeset dset = new treeset();
dset.add(new dog(2));
dset.add(new dog(1));
dset.add(new dog(3));
iterator iterator = dset.iterator();
while (iterator.hasnext()) {
system.out.print(iterator.next() + " ");
}
}
}
compile ok, but run-time error occurs:
exception in thread "main" java.lang.classcastexception:
collection.dog cannot be cast to java.lang.comparable
at java.util.treemap.put(unknown source)
at java.util.treeset.add(unknown source)
at collection.testtreeset.main(testtreeset.java:22)
class dog implements comparable{
int size;
public dog(int s) {
size = s;
}
public string tostring() {
return size + "";
}
@override
public int compareto(dog o) {
return size - o.size;
}
}
the output is:
1 2 3
4. hashset example
hashset dset = new hashset();
dset.add(new dog(2));
dset.add(new dog(1));
dset.add(new dog(3));
dset.add(new dog(5));
dset.add(new dog(4));
iterator iterator = dset.iterator();
while (iterator.hasnext()) {
system.out.print(iterator.next() + " ");
}
output:
5 3 2 1 4
note the order is not certain.
5. linkedhashset example
linkedhashset dset = new linkedhashset();
dset.add(new dog(2));
dset.add(new dog(1));
dset.add(new dog(3));
dset.add(new dog(5));
dset.add(new dog(4));
iterator iterator = dset.iterator();
while (iterator.hasnext()) {
system.out.print(iterator.next() + " ");
}
the order of the output is certain and it is the insertion order.
2 1 3 5 4
6. performance testing
public static void main(string[] args) {
random r = new random();
hashset hashset = new hashset();
treeset treeset = new treeset();
linkedhashset linkedset = new linkedhashset();
// start time
long starttime = system.nanotime();
for (int i = 0; i < 1000; i++) {
int x = r.nextint(1000 - 10) + 10;
hashset.add(new dog(x));
}
// end time
long endtime = system.nanotime();
long duration = endtime - starttime;
system.out.println("hashset: " + duration);
// start time
starttime = system.nanotime();
for (int i = 0; i < 1000; i++) {
int x = r.nextint(1000 - 10) + 10;
treeset.add(new dog(x));
}
// end time
endtime = system.nanotime();
duration = endtime - starttime;
system.out.println("treeset: " + duration);
// start time
starttime = system.nanotime();
for (int i = 0; i < 1000; i++) {
int x = r.nextint(1000 - 10) + 10;
linkedset.add(new dog(x));
}
// end time
endtime = system.nanotime();
duration = endtime - starttime;
system.out.println("linkedhashset: " + duration);
}
from the output below, we can clearly wee that hashset is the fastest one.
hashset: 2244768
treeset: 3549314
linkedhashset: 2263320
if you enjoyed this article and want to learn more about java collections, check out this collection of tutorials and articles on all things java collections.
Published at DZone with permission of Ryan Wang. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments