Openshift and AWS Lambda Deployment With Quarkus
Nowadays Quarkus is known as Supersonic Subatomic Java. It provides a lot of features to facilitate build and deployment.
Join the DZone community and get the full member experience.
Join For FreeNowadays Quarkus is known as Supersonic Subatomic Java. It provides a lot of features to facilitate build and deployment. I did my best by creating a small blog application with quarkus with installed features: rest-client, security, spring-data-jpa, smallrye-health and openapi, Kubernetes, and AWS deployment to try it real.
There are many things that one can do with the Quarkus system if they are interested in getting the most life out of it that they possibly can. It is important to understand the prerequisites as well as what the system can and cannot do at this time. It is certainly not easy to get everything done with it until you know about that.
Today, we will explore exactly what you need to do to make a difference using your Quarkus system. We will also look at all of the technical specifications that are necessary to get it up and running at this time. That should prove helpful for you as you work on making the most of every coding program that comes your way.
Prerequisites
JDK 11— for running app
Gradle 6+ — for packaging
Docker
OpenShift CLI — for deploying on OpenShift
Docker
Quarkus provides extensions for building (and pushing) container images. Currently, it supports Jib, S2I, Docker.
To working with Docker containerization add Gradle dependency:
implementation 'io.quarkus:quarkus-container-image-docker'
Configure the docker image and remote registry in application.properties
:
xxxxxxxxxx
quarkus.container-image.name=blog-api
quarkus.container-image.tag=latest
quarkus.container-image.registry=docker.io
quarkus.container-image.username=<USERNAME>
quarkus.container-image.password=<PASSWORD>
quarkus.container-image.push=true
Or override properties on Gradle command:
xxxxxxxxxx
./gradlew quarkusBuild
-Dquarkus.container-image.username=<USERNAME> \
-Dquarkus.container-image.password=<PASSWORD> \
-Dquarkus.container-image.push=true
As it’s possible to create a multistage Dockerfile to avoid packaging:
xxxxxxxxxx
####
# This Dockerfile.multistage is used in order to build a container that runs the Quarkus application in JVM mode
# docker build -t quarkus-quickstart:jvm -f src/main/docker/Dockerfile.multistage .
# docker run -i --rm -p 8080:8080 quarkus-quickstart:jvm
###
# Build jar with gradle
FROM gradle:jdk11 AS build_image
ENV APP_HOME=/root/dev/myapp/
USER root
RUN mkdir -p $APP_HOME/src/main/java
WORKDIR $APP_HOME
COPY build.gradle gradlew gradlew.bat $APP_HOME
COPY gradle $APP_HOME/gradle
COPY . .
RUN ./gradlew -PawsLambdaEnabled=false quarkusBuild
FROM registry.access.redhat.com/ubi8/ubi-minimal:8.1
ARG JAVA_PACKAGE=java-11-openjdk-headless
ARG RUN_JAVA_VERSION=1.3.5
ENV LANG='en_US.UTF-8' LANGUAGE='en_US:en'
# Install java and the run-java script
# Also set up permissions for user `1001`
RUN microdnf install curl ca-certificates ${JAVA_PACKAGE} \
&& microdnf update \
&& microdnf clean all \
&& mkdir /deployments \
&& chown 1001 /deployments \
&& chmod "g+rwX" /deployments \
&& chown 1001:root /deployments \
&& curl https://repo1.maven.org/maven2/io/fabric8/run-java-sh/${RUN_JAVA_VERSION}/run-java-sh-${RUN_JAVA_VERSION}-sh.sh -o /deployments/run-java.sh \
&& chown 1001 /deployments/run-java.sh \
&& chmod 540 /deployments/run-java.sh \
&& echo "securerandom.source=file:/dev/urandom" >> /etc/alternatives/jre/lib/security/java.security
# Configure the JAVA_OPTIONS, you can add -XshowSettings:vm to also display the heap size.
ENV JAVA_OPTIONS="-Dquarkus.http.host=0.0.0.0 -Djava.util.logging.manager=org.jboss.logmanager.LogManager"
COPY --from=build_image /root/dev/myapp/build/lib/* /deployments/lib/
COPY --from=build_image /root/dev/myapp/build/*-runner.jar /deployments/app.jar
EXPOSE 8090
USER 1001
ENTRYPOINT [ "/deployments/run-java.sh" ]
OpenShift Deployment
To deploy on Openshift without Quarkus possible to define template.yaml:
xxxxxxxxxx
apiVersion v1
kind Template
metadata
name my-template
objects
kind DeploymentConfig
apiVersion v1
metadata
labels
${TAG} $ SELECTOR_APP_NAME
name $ APP_NAME
namespace $ NAMESPACE_NAME
spec
replicas1
revisionHistoryLimit10
selector
app $ APP_NAME
deploymentconfig $ APP_NAME
strategy
activeDeadlineSeconds21600
resources
rollingParams
intervalSeconds1
maxSurge 25%
maxUnavailable 25%
timeoutSeconds600
updatePeriodSeconds1
type Rolling
template
metadata
annotations
openshift.io/generated-by OpenShiftNewApp
creationTimestamp null
labels
app $ APP_NAME
deploymentconfig $ APP_NAME
spec
containers
env
name $ APP_NAME
ports
containerPort8090
protocol TCP
resources
dnsPolicy ClusterFirst
restartPolicy Always
schedulerName default-scheduler
securityContext
terminationGracePeriodSeconds30
testfalse
triggers
type ConfigChange
imageChangeParams
automatictrue
containerNames
$ APP_NAME
from
kind ImageStreamTag
name'blog-api:latest'
namespace $ NAMESPACE_NAME
type ImageChange
kind Service
apiVersion v1
metadata
labels
${TAG} $ SELECTOR_APP_NAME
name $ APP_NAME
namespace $ NAMESPACE_NAME
spec
ports
name 80-tcp
port80
protocol TCP
targetPort8090
selector
app $ APP_NAME
deploymentconfig $ APP_NAME
sessionAffinity None
type ClusterIP
status
loadBalancer
kind Route
apiVersion v1
metadata
labels
${TAG} $ SELECTOR_APP_NAME
name $ APP_NAME
namespace $ NAMESPACE_NAME
spec
host $ HOST_NAME
port
targetPort 80-tcp
to
kind Service
name $ APP_NAME
weight100
wildcardPolicy None
parameters
name NAMESPACE_NAME
name SELECTOR_APP_NAME
name APP_NAME
value quarkus-blog-api
name HOST_NAME
name TAG
value app
And processed:
xxxxxxxxxx
oc tag elvaliev/blog-api:latest
oc process NAMESPACE_NAME=<OPENSHIFT-PROJECT> \
SELECTOR_APP_NAME=<SELECTOR> \
HOST_NAME=<APPLICATION_HOST> \
-f template.yaml | oc apply -f-
By using docker containerization it’s also possible to deploy an application using image-stream from the docker registry.
xxxxxxxxxx
oc new-app elvaliev/blog-api:latest
oc expose svc/blog-api
But, Quarkus offers the ability to automatically generate Kubernetes resources. It currently supports generating resources for Kubernetes, OpenShift, and Knative.
Gradle dependency:
implementation 'io.quarkus:quarkus-kubernetes'
To enable the generation of necessary templates you need to include the target in application.properties
:
quarkus.kubernetes.deployment-target=kubernetes,openshift
Optionally you could set up the custom configuration for your template:
xxxxxxxxxx
quarkus.kubernetes.annotations.app=blog
quarkus.kubernetes.labels.app=blog
# To create route for your application
quarkus.openshift.expose=true
After packaging quarkus generate target templates:
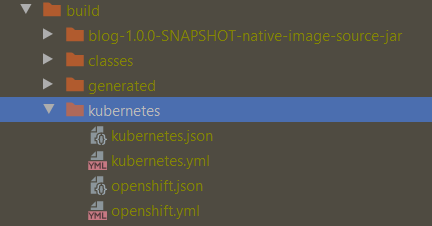
Deploy application via command:
oc create -f build/kubernetes/openshift.yml
xxxxxxxxxx
oc new-app quay.io/quarkus/ubi-quarkus-native-s2i:19.3.1-java11~<GIT REPOSITORY>
--context-dir=. --name=quarkus-blog-api
oc expose svc/quarkus-blog-a
AWS Deployment
Note: use only the last version of quarkus — 1.4.1.
Dependency for Gradle:implementation 'io.quarkus:quarkus-amazon-lambda-http'
After packaging quarkus generate sam templates for AWS:
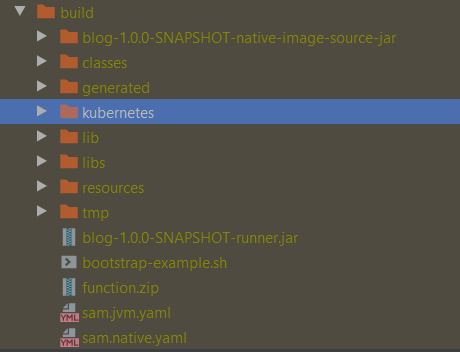
For deploying an application as an AWS Lambda use template from the build. For native build use template build/sam.native.yaml
.
I followed quarkus-documentation:
xxxxxxxxxx
sam local start-api --template build/sam.jvm.yaml
sam package --template-file build/sam.jvm.yaml --output-template-file packaged.yaml --s3-bucket <S3_BUCKET>
sam deploy --template-file packaged.yaml --capabilities CAPABILITY_IAM --stack-name <YOUR_STACK_NAME>
Note: To avoiding timeout error (502 — BAD GATEWAY) — increase Timeout
in build/sam.jvm.yaml
quarkus-amazon-lambda-http
dependency on jar packaging (packaging with the condition properties awsLambdaEnabled=false
from gradle.properties
):
xxxxxxxxxx
if (project.property(“awsLambdaEnabled”) != “true”) {
configurations {
runtime.exclude group: ‘io.quarkus’,
module: ‘quarkus-amazon-lambda-http’
}
}
Opinions expressed by DZone contributors are their own.
Comments