Sound Synthesis with Numpy
Join the DZone community and get the full member experience.
Join For FreePhysically, sound is an oscillation of a mechanical medium that makes the surrounding air also oscillate and transport the sound as a compression wave. Mathematically, the oscillations can be described as

where t is the time, and f the frequency of the oscillation. Each musical note vibrates at a particular frequency and to generate a tone we have to generate an oscillation with the appropriate frequency. The following table shows the complete musical scale between middle A and A-880, in the first column we have the tone and in the second the frequency that we have to use to generate the tone:
Sound on a computer is a sequence of numbers and we are going to see how to generate an array that represents a musical tone with numpy. The following function is able to generate a note using the formula above:
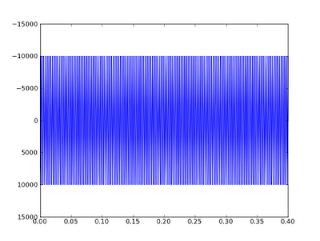
Blog Source: http://glowingpython.blogspot.com/2011/09/sound-synthesis.html
Article Type:
How-to

where t is the time, and f the frequency of the oscillation. Each musical note vibrates at a particular frequency and to generate a tone we have to generate an oscillation with the appropriate frequency. The following table shows the complete musical scale between middle A and A-880, in the first column we have the tone and in the second the frequency that we have to use to generate the tone:
Tone | Freq |
A | 440 |
B flat | 466 |
B | 494 |
C | 523 |
C sharp | 554 |
D | 587 |
D sharp | 622 |
E | 659 |
F | 698 |
F sharp | 740 |
G | 784 |
A flat | 831 |
A | 880 |
from numpy import linspace,sin,pi,int16 # tone synthesis def note(freq, len, amp=1, rate=44100): t = linspace(0,len,len*rate) data = sin(2*pi*freq*t)*amp return data.astype(int16) # two byte integersAnd we can use this function to generate an A tone of 2 seconds with 44100 samples per second in this way:
from scipy.io.wavfile import write from pylab import plot,show,axis # A tone, 2 seconds, 44100 samples per second tone = note(440,2,amp=10000) write('440hzAtone.wav',44100,tone) # writing the sound to a file plot(linspace(0,2,2*44100),tone) axis([0,0.4,15000,-15000]) show()The script put the sound into a wav file and we can play it with an external player. This plot shows a part of the signal generated by the script:
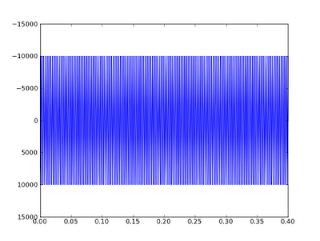
Blog Source: http://glowingpython.blogspot.com/2011/09/sound-synthesis.html
Article Type:
How-to
NumPy
Opinions expressed by DZone contributors are their own.
Comments