How to Test PATCH Requests for API Testing With Playwright Java
This tutorial demonstrates how to test PATCH requests using the Playwright Java framework for API testing, using examples of partially updating data of an API.
Join the DZone community and get the full member experience.
Join For FreeAutomated API testing offers multiple benefits, including speeding up the testing lifecycle and providing faster feedback. It helps in enhancing the efficiency of the APIs and allows teams to deliver the new features speedily to the market.
There are multiple tools and frameworks available in the market today that offer automation testing of the APIs, including Postman, Rest Assured, SuperTest, etc. The latest entry on this list is the Playwright framework, which offers API and Web Automation Testing.
In this tutorial blog, we will discuss and cover the following points:
- What is a PATCH API request?
- How do you test PATCH API requests in automation testing using Playwright Java?
Getting Started
It is recommended to check out the earlier tutorial blog to know about the details related to prerequisite, setup and configuration.
Application Under Test
We will be using the free-to-use RESTful e-commerce APIs available over GitHub.
This application can be set up using NodeJS or Docker. It offers multiple APIs related to order management functionality that allows creating, retrieving, updating, and deleting orders.
What Is a PATCH Request?
A PATCH request is used for partially updating a resource. It is the same as a PUT request. However, the difference is that PUT requires the whole request body to be sent in the request, while with PATCH, we can send only the required fields in the request that need to be updated.
Another difference between a PUT and a PATCH request is that a PUT request is always idempotent; that is, making the same request repeatedly does not change the state of the resource, whereas a PATCH request may not always be idempotent.
The following is an example of updating the order with a PATCH request using the RESTful e-commerce API. The same PATCH API will be further used in this blog to write the automation tests using Playwright Java.
PATCH (/partialUpdateOrder/{id})
This partially updates the order using its Order ID.
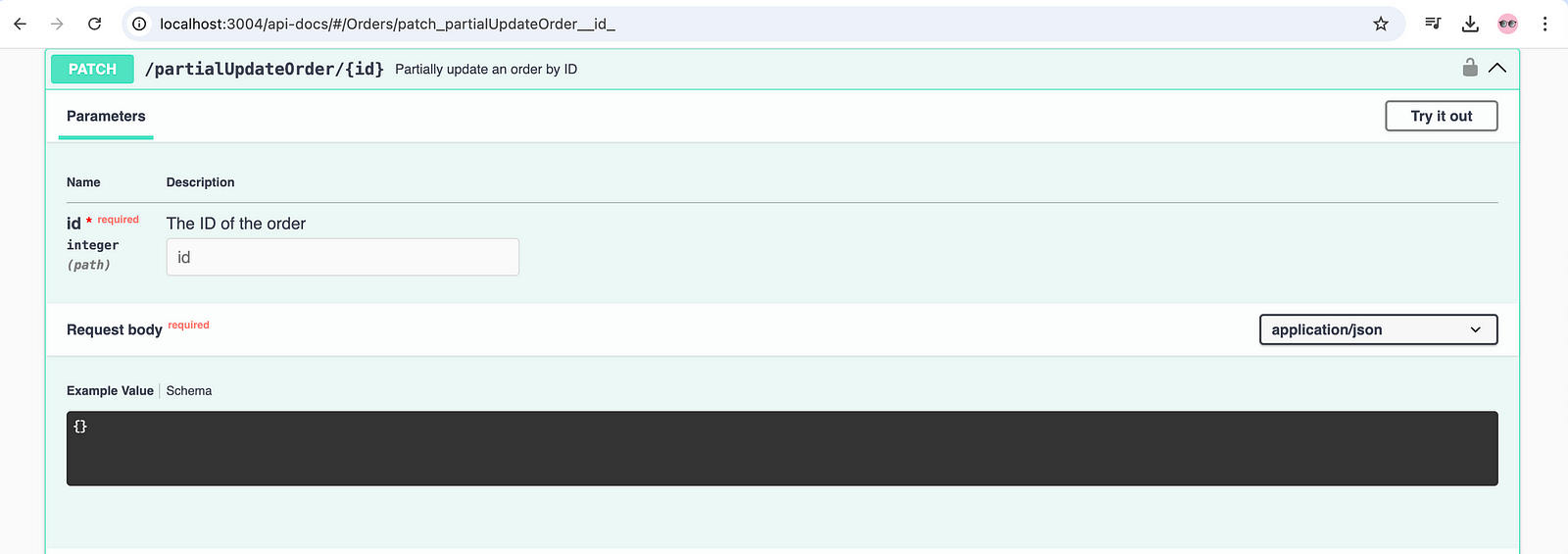
This API needs the id
i.e., order_id
as the Path Parameter to check for the existing order to partially update it. The partial details of the order must be supplied in the JSON format in the request body. Since it is a PATCH request, we just need to send the fields that we need to update; all other details do not need to be included in the request.
Additionally, as a security measure, a valid Authentication token must be supplied with the PATCH request, otherwise the request will fail.
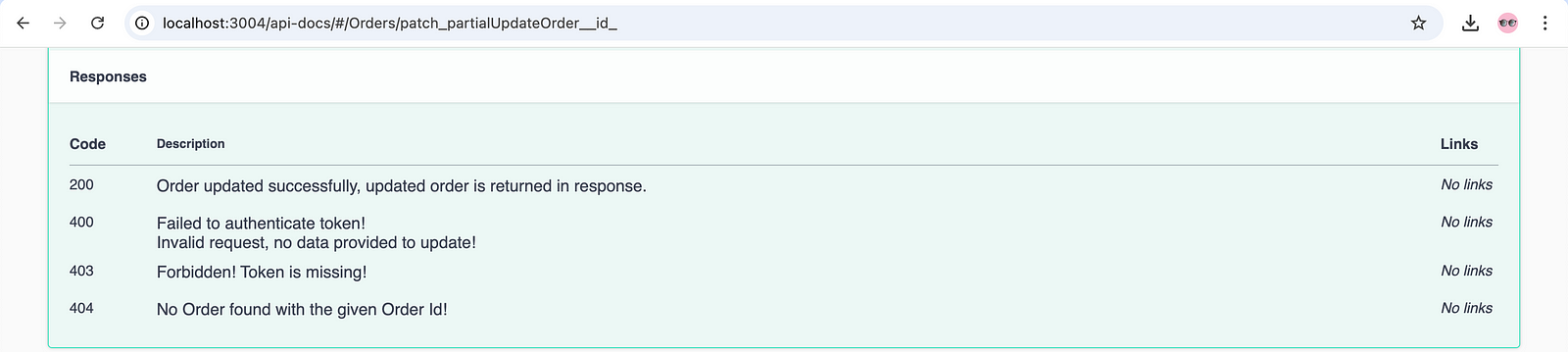
The PATCH request will return the updated order details in the response with a Status Code 200.
In case the update using the PATCH request fails, based on the criteria, the following status codes will be displayed:
Status Code | Criteria |
---|---|
404 |
When there are no order for the respective |
400 | Token Authenication Fails /Incorrect request body or No request body is sent in the request |
403 | No Authentication is supplied while sending the request |
How to Test PATCH APIs Using Playwright Java
Playwright offers the required methods that allow performing API testing seamlessly. Let’s now delve into writing the API automation tests for PATCH API requests using Playwright Java.
The PATCH API ( /partialUpdateOrder/{id}
) will be used for updating the order partially.
Test Scenario: Update Order Using PATCH
- Start the RESTful e-commerce service.
- Use POST requests to create some orders in the system.
- Update the
product_name, product_amt and qty
oforder_id
- "1." - Check that the Status Code 200 is returned in the response.
- Check that the order details have been updated correctly.
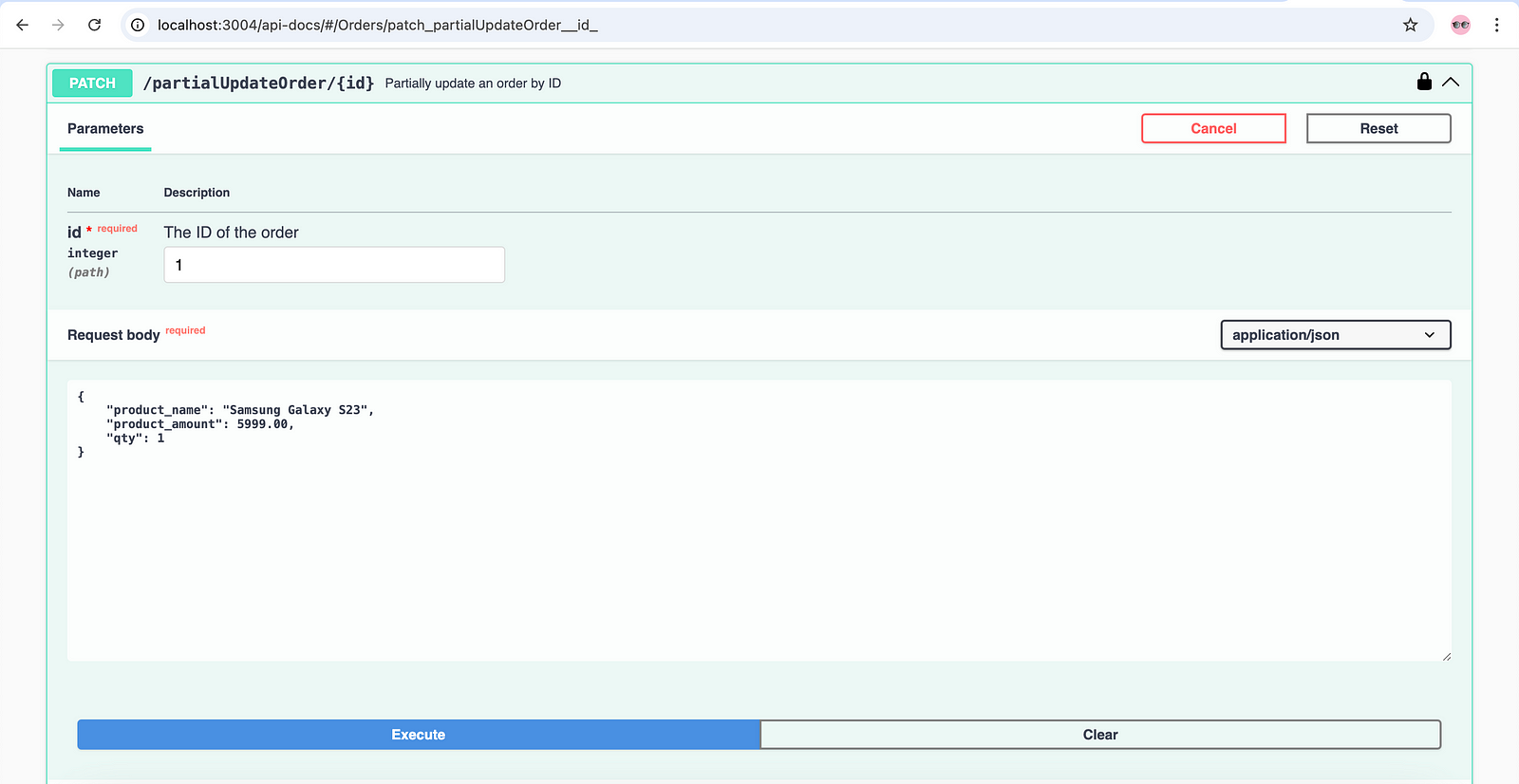
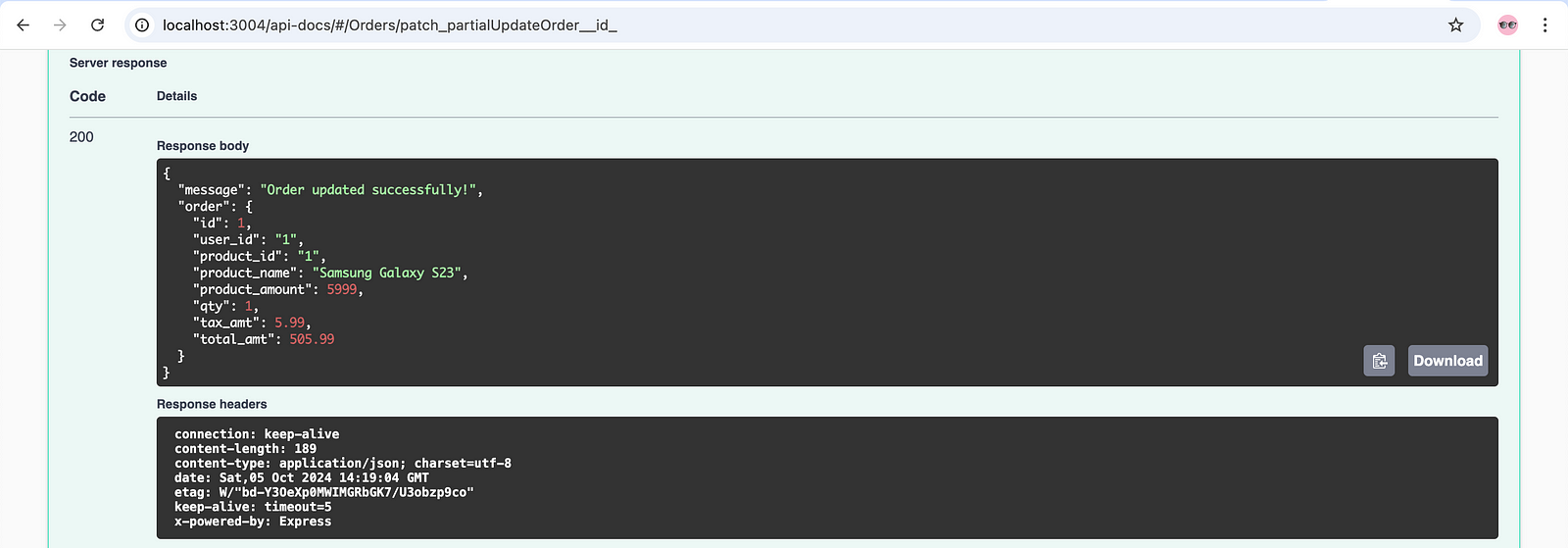
Test Implementation
To update the order partially, we need to send in the request body with partial fields to update and the authorization token. This token ensures that a valid user of the application is updating the order.
1. Generate the Authentication Token
The token can be generated using the POST /auth
API endpoint.
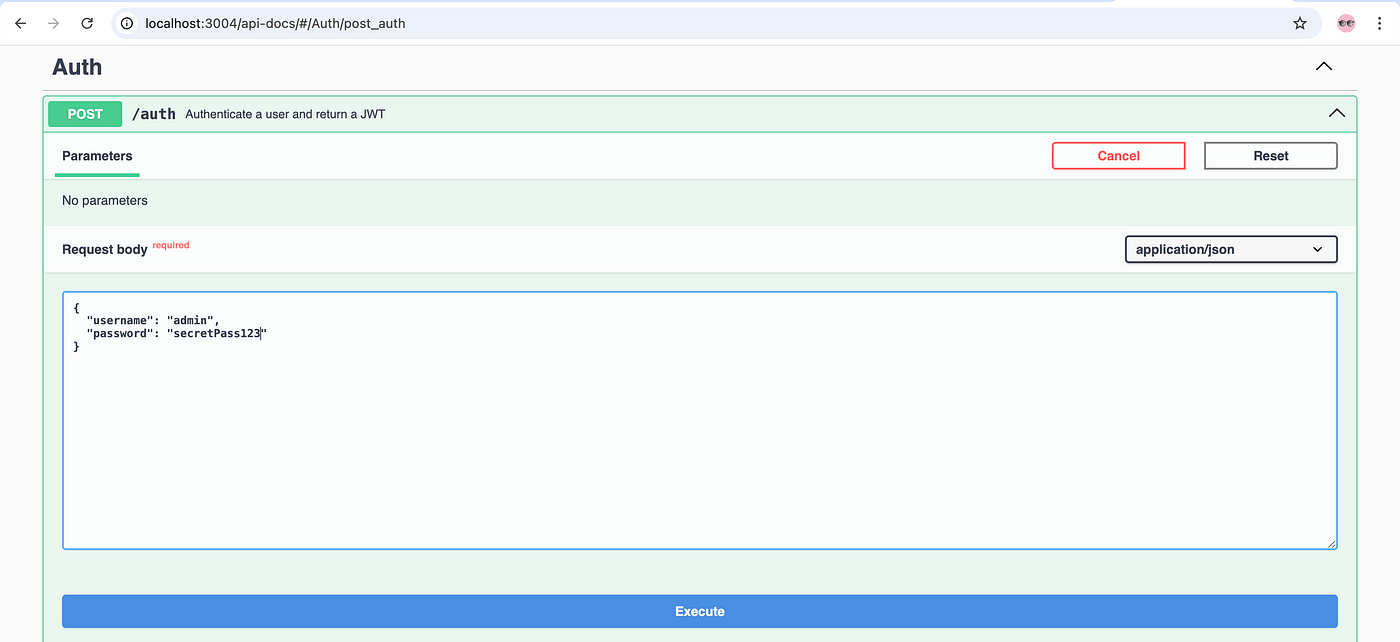
This API endpoint needs the login credentials to be supplied in the request body. The valid login credentials are as follows:
Field name | Value |
---|---|
Username | admin |
Password | secretPass123 |
On passing these valid login credentials, the API returns the JWT token in the response with Status Code 200.
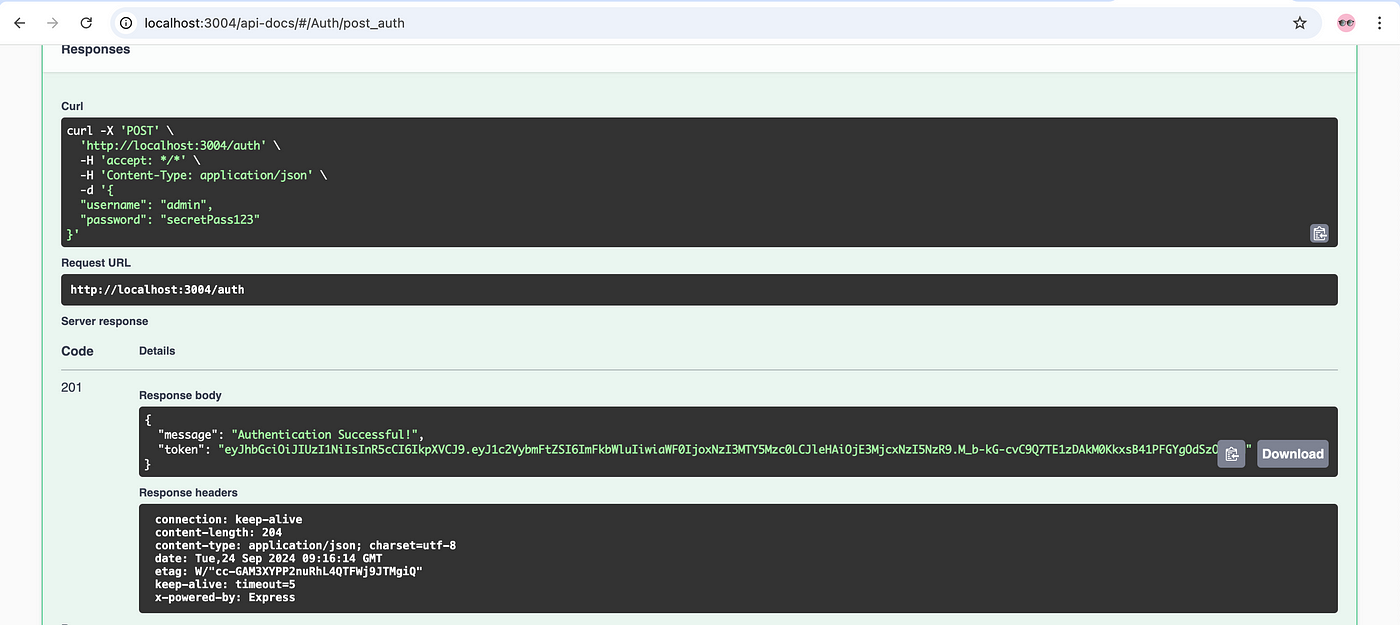
We would be generating and using the token using the getCredentials()
method from the TokenBuilder
class that is available in the testdata
package.
public static TokenData getCredentials() {
return TokenData.builder().username("admin")
.password("secretPass123")
.build();
}
This getCredentials()
method returns a TokenData
object containing the username
and password
fields.
@Getter
@Builder
public class TokenData {
private String username;
private String password;
}
Once the token is generated it can be used in the PATCH API request for partially updating the order.
2. Generate the Test Data for Updating Order
The next step in updating the order partially is to generate the request body with the required data.
As discussed in the earlier blog of POST request tutorial, we would be adding a new method getPartialUpdatedOrder()
in the existing class OrderDataBuilder
that generates that test data on runtime.
public static OrderData getPartialUpdatedOrder() {
return OrderData.builder()
.productName(FAKER.commerce().productName())
.productAmount(FAKER.number().numberBetween(550,560))
.qty(FAKER.number().numberBetween(3, 4))
.build();
}
This method will use only three fields, which are product_name
, product_amount
and qty
and accordingly, use them to generate a new JSON object that would be passed on as the request body to the PATCH API request.
3. Update the Order Using PATCH Request
We have come to the final stage now, where we will be testing the PATCH API request using Playwright Java.
Let’s create a new test method testShouldPartialUpdateTheOrderUsingPatch()
in the existing HappyPathTests
class.
@Test
public void testShouldPartialUpdateTheOrderUsingPatch() {
final APIResponse authResponse = this.request.post("/auth", RequestOptions.create().setData(getCredentials()));
final JSONObject authResponseObject = new JSONObject(authResponse.text());
final String token = authResponseObject.get("token").toString();
final OrderData partialUpdatedOrder = getPartialUpdatedOrder();
final int orderId = 1;
final APIResponse response = this.request.patch("/partialUpdateOrder/" + orderId, RequestOptions.create()
.setHeader("Authorization", token)
.setData(partialUpdatedOrder));
final JSONObject updateOrderResponseObject = new JSONObject(response.text());
final JSONObject orderObject = updateOrderResponseObject.getJSONObject("order");
assertEquals(response.status(), 200);
assertEquals(updateOrderResponseObject.get("message"), "Order updated successfully!");
assertEquals(orderId, orderObject.get("id"));
assertEquals(partialUpdatedOrder.getProductAmount(), orderObject.get("product_amount"));
assertEquals(partialUpdatedOrder.getQty(), orderObject.get("qty"));
}
This method will first hit the Authorization API to generate the token. The response from the Authorization API will be stored in the authResponseObject
variable that will further be used to extract the value from the token field available in response.
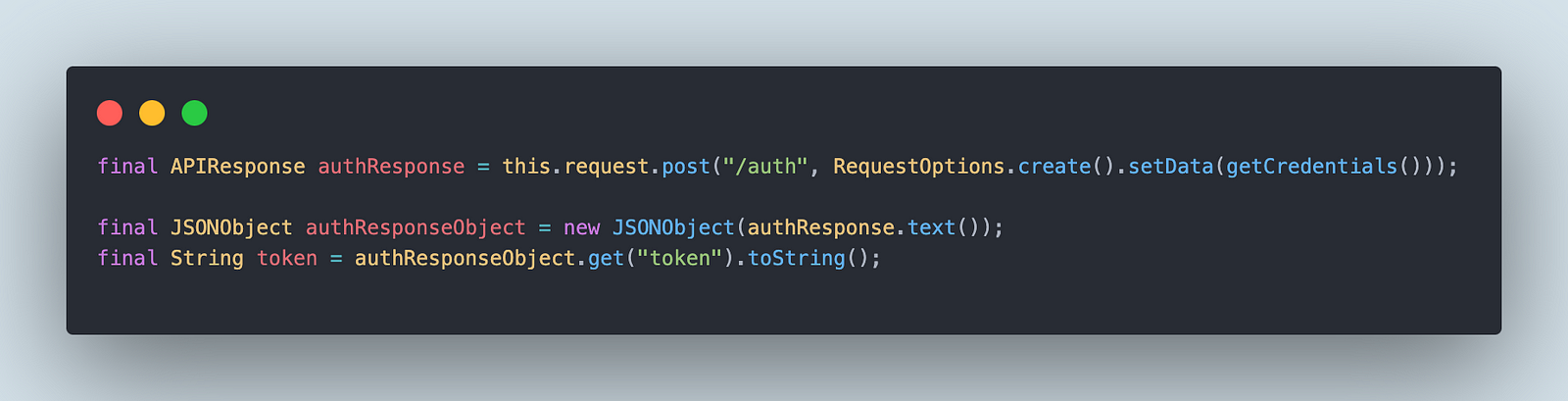
The request body required to be sent in the PATCH API will be generated and will be stored in the partialUpdateOrder
object. This is done so we can use this object further for validating the response.
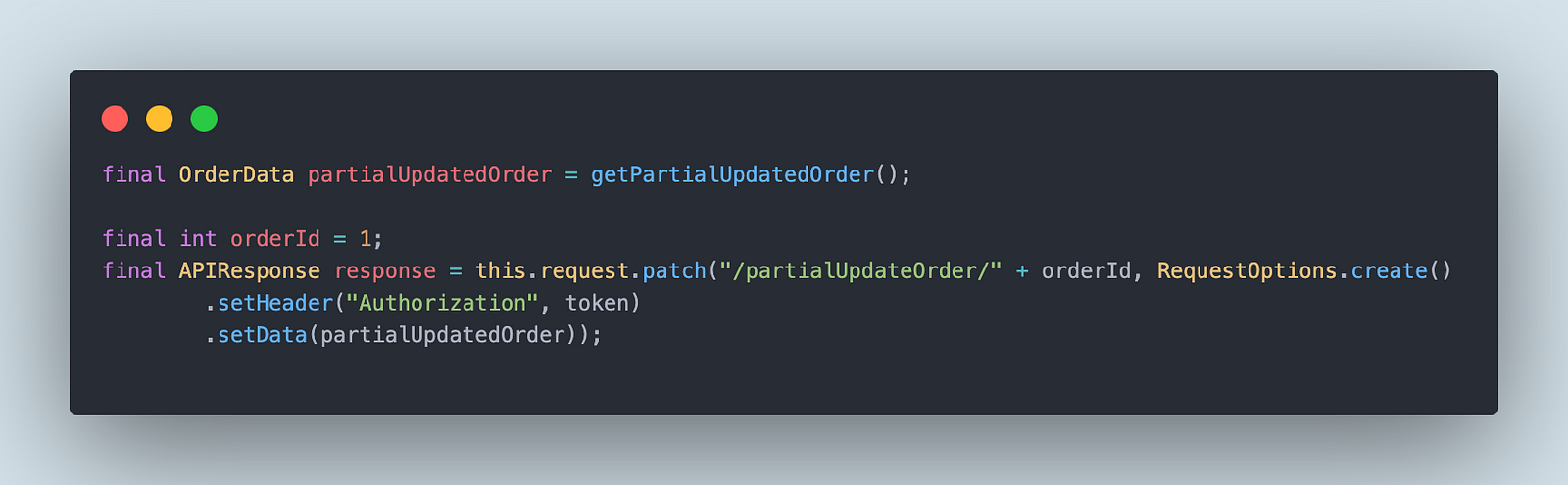
Next, the token will be set using the setHeader()
method, and the request body object will be sent using the setData()
method. The PATCH API request will be handled using the patch()
method of Playwright, which will allow partial updating of the order.
Response body:
{
"message": "Order updated successfully!",
"order": {
"id": 1,
"user_id": "1",
"product_id": "1",
"product_name": "Samsung Galaxy S23",
"product_amount": 5999,
"qty": 1,
"tax_amt": 5.99,
"total_amt": 505.99
}
}
The response received from this PATCH API will be stored in the response
variable and will be used further to validate the response.
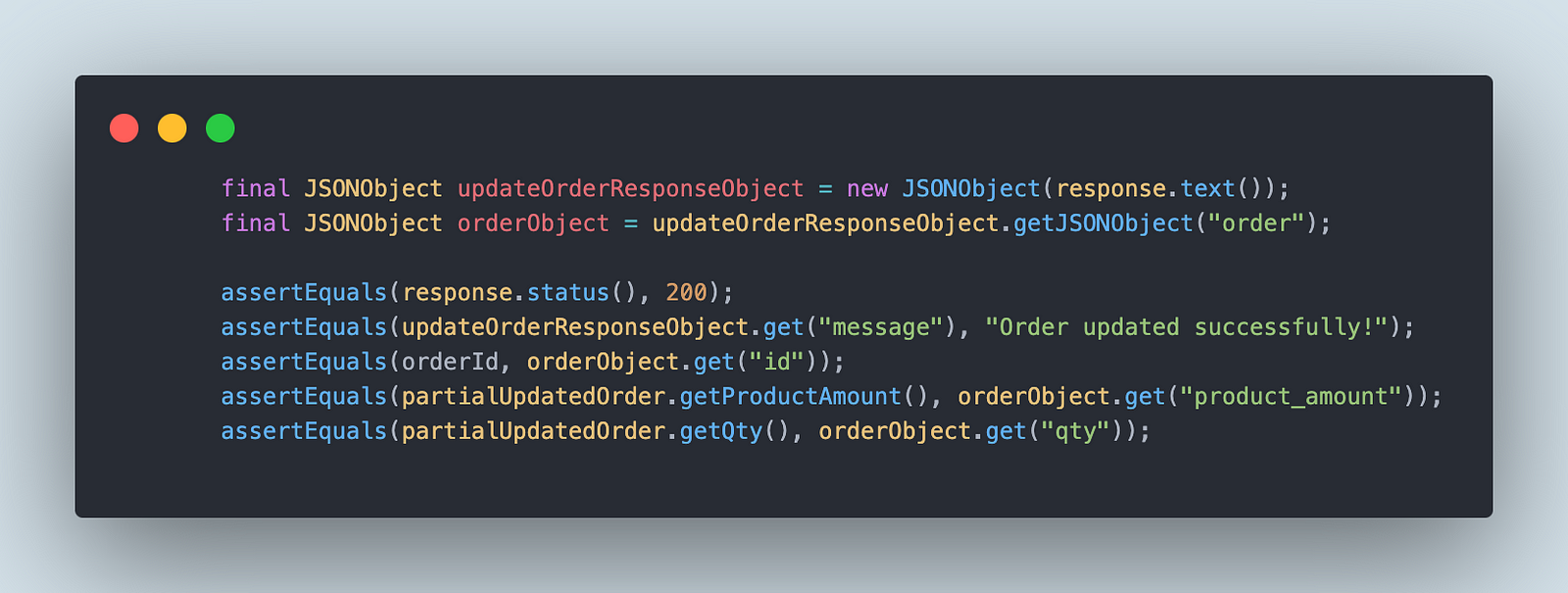
The last step is to perform assertions, the response from the PATCH API returns a JSON object that will be stored in the object named updateOrderResponseObject
.
The message
field is available in the main response body. Hence, it will be verified using the updateOrderResponseObject
calling the get()
method that will return the value of the message
field.
The JSON object order
received in the Response is stored in the object named orderObject
that will be used for checking the values of the order details.
The partialUpdateOrder
object that actually stores the request body that we sent to partially update the order will be used as expected values, and the orderObject
will be used for actual values finally performing the assertions.
Test Execution
We will be creating a new testng.xml
file ( testng-restfulecommerce-partialupdateorder.xml
) to execute the test sequentially, i.e., first calling the POST API test to generate orders and then calling the PATCH API test to partially update the order.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Restful ECommerce Test Suite">
<test name="Testing Happy Path Scenarios of Creating and Updating Orders">
<classes>
<class name="io.github.mfaisalkhatri.api.restfulecommerce.HappyPathTests">
<methods>
<include name="testShouldCreateNewOrders"/>
<include name="testShouldPartialUpdateTheOrderUsingPatch"/>
</methods>
</class>
</classes>
</test>
</suite>
The following test execution screenshot from IntelliJ IDE shows that the tests were executed successfully, and a partial update of the order was successful.
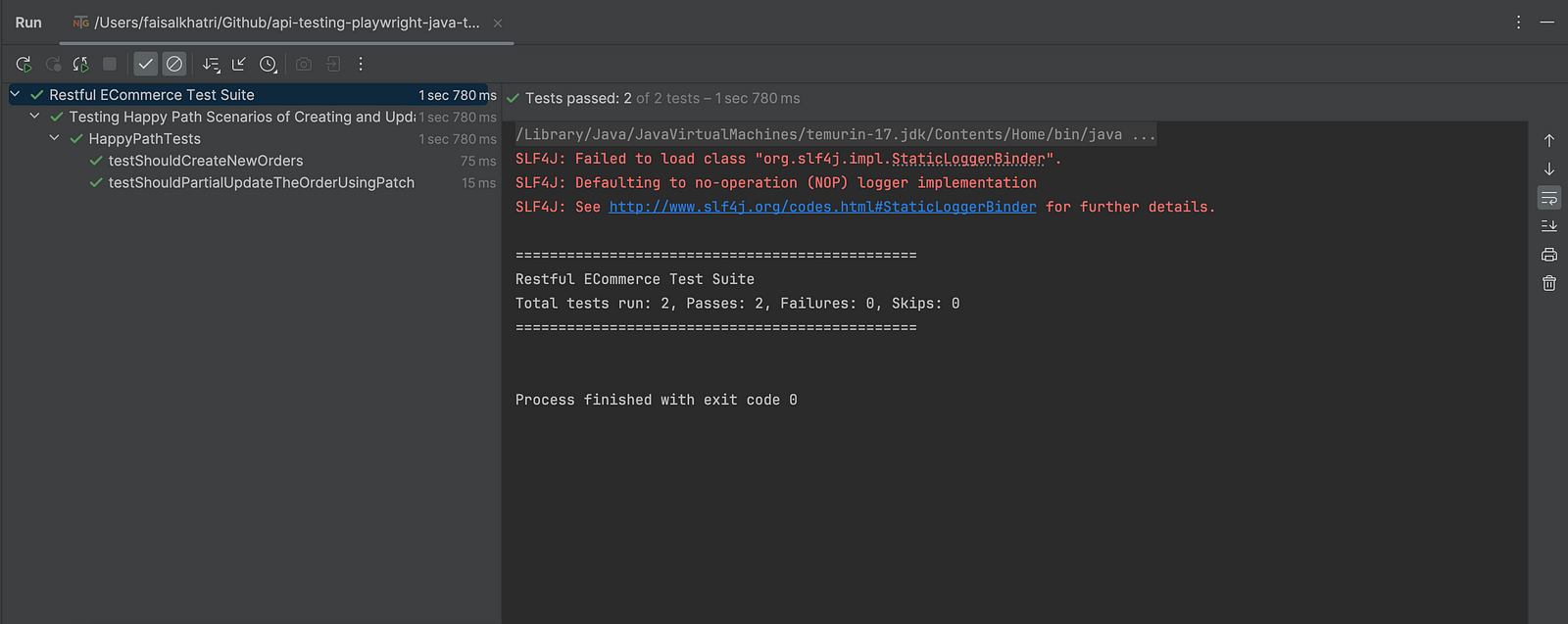
Summary
PATCH API requests allow updating the resource partially. It allows flexibility to update a particular resource as only the required fields can be easily updated using it. In this blog, we tested the PATCH API requests using Playwright Java for automation testing.
Testing all the HTTP methods is equally important while performing API testing. We should perform isolated tests for each endpoint as well as end-to-end testing for all the APIs to make sure that all APIs of the application are well integrated with each other and run seamlessly.
Published at DZone with permission of Faisal Khatri. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments