Integrate Cucumber in Playwright With Java
Integrating Cucumber with Playwright combines natural language scenarios with browser automation, boosting project performance.
Join the DZone community and get the full member experience.
Join For FreeCucumber is a popular behavior-driven development (BDD) framework that allows you to write executable specifications in a natural language format. It promotes collaboration between stakeholders, developers, and testers by providing a common language that everyone can understand. Cucumber supports various programming languages, including Java, and provides a rich set of features for defining and executing test scenarios.
Playwright, on the other hand, is a powerful open-source automation framework for web browsers that supports multiple programming languages, including Java. It provides a high-level API for automating web browsers such as Chrome, Firefox, and Safari. Playwright offers robust browser automation capabilities, including interactions with web pages, taking screenshots, handling cookies, and much more.
By integrating Cucumber with Playwright, you can leverage the expressive power of Cucumber’s natural language scenarios and combine it with Playwright’s browser automation capabilities. This combination allows you to write tests in a human-readable format and execute them against real browsers, enabling end-to-end testing of web applications.
Integrating Cucumber with Playwright in Java provides a seamless way to express and automate acceptance criteria, making it easier to collaborate and ensure the quality of your web applications.
Pre-Condition
Scenarios Covered Under This Blog With Cucumber
- Launch application
- Verify the title of the application.
- Login into the site.
- Verify the product
- Click on Hamburger and Logout of the application.
High-Level Steps to Implement the Above Scenario Using Playwright With Java
- Create Maven Project
- Create packages for the pages class.
- Create packages for testrunner, features, and stepdefinitions.
- Create a feature file under ->features.
- Create page class for HomePage and LoginPage.
- Create methods under the LoginPage class.
- Create methods under the HomePage class.
- Create testclass under ->stepdefinitions.
- Create testrunner class under ->testrunner.
- Run the test cases.
Explanation of Steps
Add Playwright and Other Dependencies in POM.XML
Add the below dependency in POM.XML
<dependencies>
<dependency>
<groupId>com.microsoft.playwright</groupId>
<artifactId>playwright</artifactId>
<version>1.17.1</version>
</dependency>
</dependencies>
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-java</artifactId>
<version>6.9.1</version>
</dependency>
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-junit</artifactId>
<version>6.9.1</version>
<scope>compile</scope>
</dependency>
Step 1: Create Maven Project
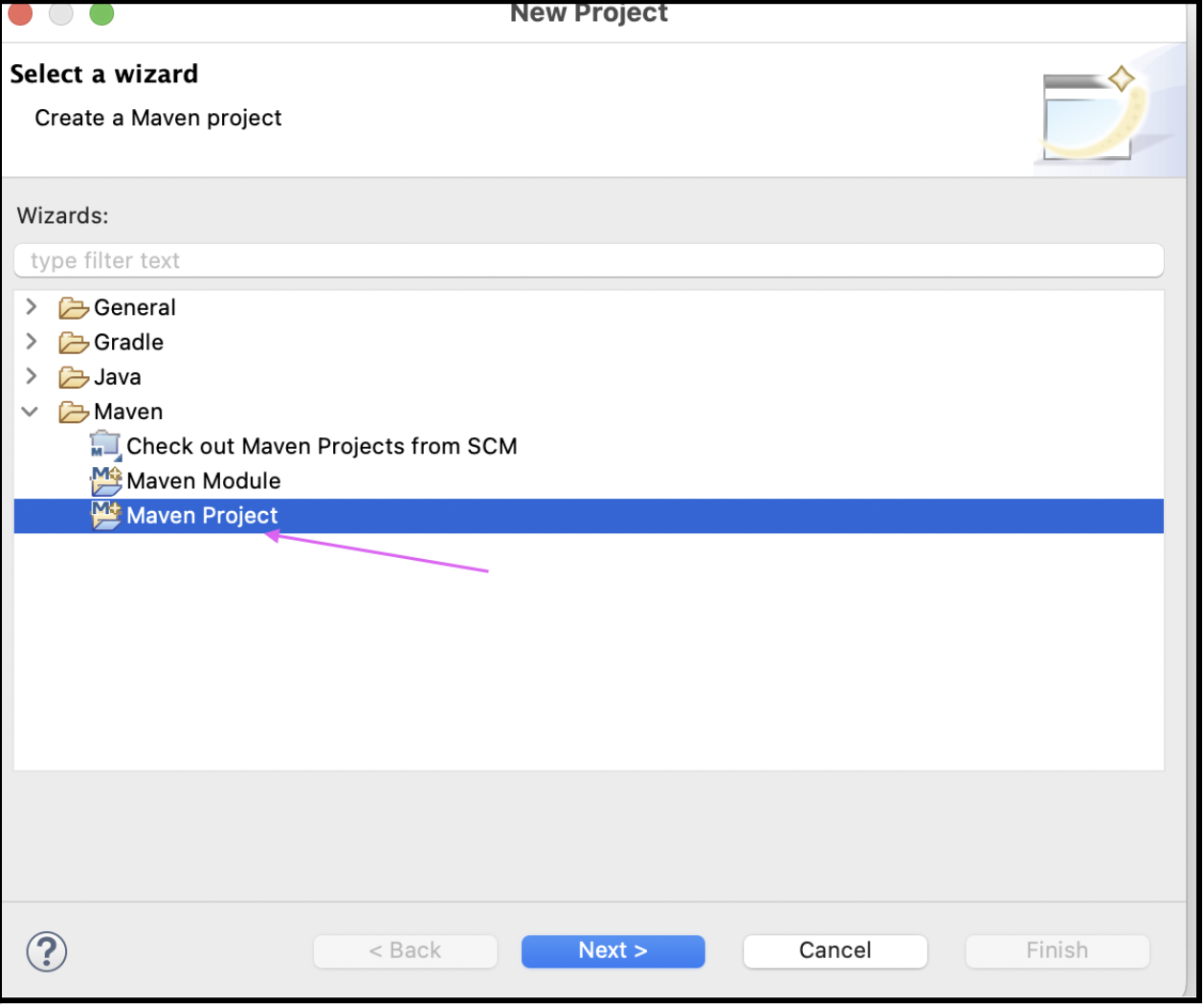
We can see below project has been created with the Name “NewPlayWrightProject.”
Step 2: Create Packages for the Pages Class
Create package under src/main/java -> with name “com.saucedemo.pages.”
Step 3: Create Packages for Testrunner, Features, and Stepdefinitions
Create three packages, “testrunner,” “features,” and “stepdefinitions,” under -> src/test/java.
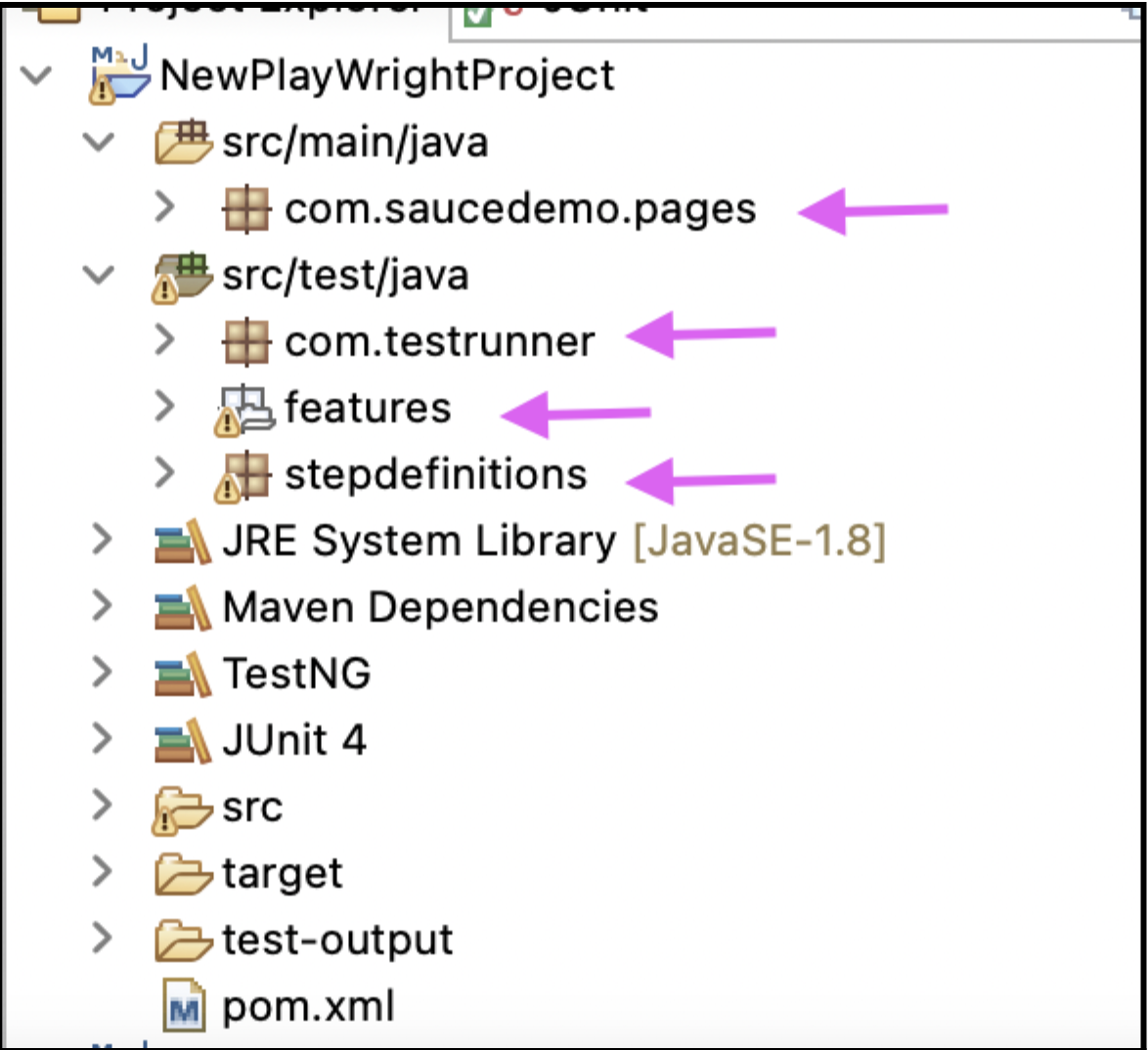
Step 4: Create a Feature File
Under the features package, create a .feature file with the name “LoginAndHomeTest.feature”.

Feature: Login
Scenario Outline: Login to the SwagLabs Application with the correct credentials.
Given User launched SwagLabs application.
When User verifies the Page title.
When User logged in to the app using the username “<UserName>” and password “<Password>.”
Then User verifies the product name “<ProductName>.”
Then User logout from the application.
Examples:
| UserName | Password | ProductName |
| standard_user | secret_sauce | Sauce Labs Backpack |
Step 5: Create Page Class for HomePage and LoginPage
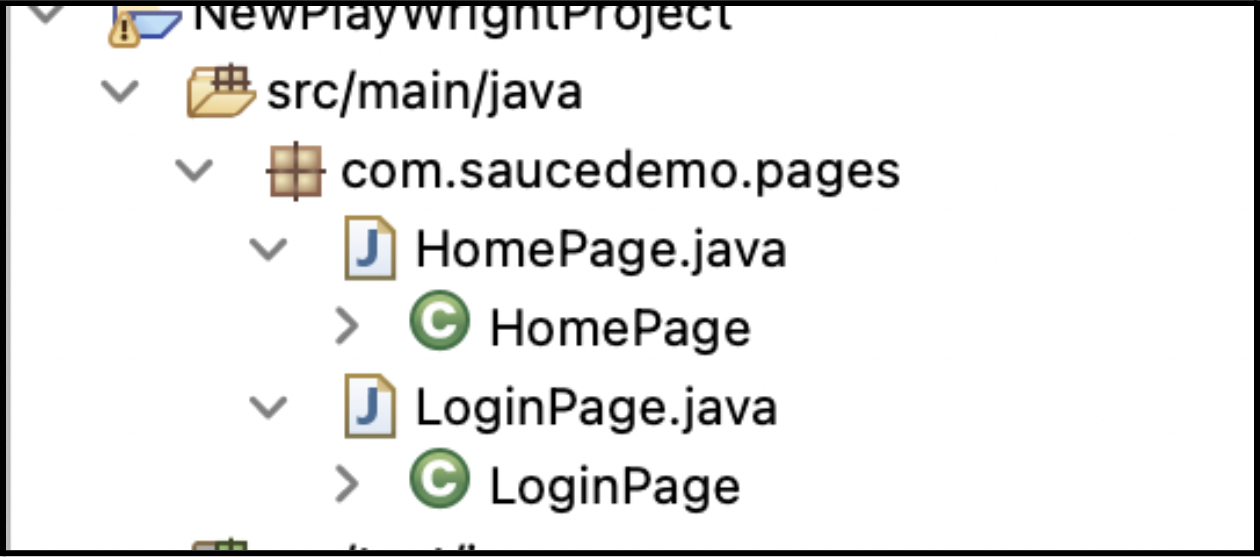
Step 6: Create Methods Under the LoginPage Class
Under LoginPage.java, create methods to login /verify the product and logout from the site.
Create Methods under package com.saucedemo.pages.
1. verifyTitle();
2. loginIntoApplication(String email, String pass);
3. logoutFromApplication();
Other Methods:
enterUserName(String email),enterPassword(String pass),clickLoginButton(),clickOnHamburger() and clickOnLogout().
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserType;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
import com.saucedemo.pages.HomePage;
import com.saucedemo.pages.LoginPage;
import io.cucumber.java.en.Given;
import io.cucumber.java.en.Then;
import io.cucumber.java.en.When;
public class LoginCucumberTest {
LoginPage login;
HomePage home;
Playwright playwright = Playwright.create();
BrowserType firefox = playwright.firefox();
Browser browser = firefox.launch(new BrowserType.LaunchOptions().setHeadless(false));
Page page = browser.newPage();
@Given(“User launched SwagLabs application”)
public void setUp() {
page.navigate(“https://www.saucedemo.com/“);
home = new HomePage(page);
login = new LoginPage(page);
}
@When(“User verify the Page title”)
public void verifyPageTitle() {
String title = login.verifyTitle();
Assert.assertEquals(title, “Swag Labs”);
}
//Login into the application
@When(“User logged in the app using username {string} and password {string}”)
public void loginIntoTheApplication(String username,String password ) {
login.loginIntoApplication(username, password);
}
//Verify product name after login
@Then(“User verify the product name {string}”)
public void verifyProductsName(String productname) {
String productName = home.getProductName();
Assert.assertEquals(productName, productname);
}
//Logout from application
@Then(“User logout from the application”)
public void logoutFromApplication() {
login.logoutApplication();}
}
Step 7: Create Methods for the HomePage Class
Under HomePage.java create the below methods.
productName(); //To verify the product name on the site.
package com.saucedemo.pages;
import com.microsoft.playwright.Page;
public class HomePage {
Page page;
// Locator — — — –
String productName_1 =”id=item_4_title_link”;
//initialize Page using constructor
public HomePage(Page page) {
this.page =page;}
//Method
public String productName() {
String productName = page.textContent(productName_1);
return productName;}}
Step 8: Create a Test Class
Under the stepdefinitions -> Create “LoginCucumberTest.java” class to call all the methods that are created under LoginPage.java and HomePage.java.

package stepdefinitions;
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserType;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
import com.saucedemo.pages.HomePage;
import com.saucedemo.pages.LoginPage;
import io.cucumber.java.en.Given;
import io.cucumber.java.en.Then;
import io.cucumber.java.en.When;
public class LoginCucumberTest {
LoginPage login;
HomePage home;
Playwright playwright = Playwright.create();
BrowserType firefox = playwright.firefox();
Browser browser = firefox.launch(new BrowserType.LaunchOptions().setHeadless(false));
Page page = browser.newPage();
@Given(“User launched SwagLabs application”)
public void setUp() {
page.navigate(“https://www.saucedemo.com/“);
home = new HomePage(page);
login = new LoginPage(page);
}
@When(“User verify the Page title”)
public void verifyPageTitle() {
String title = login.verifyTitle();
Assert.assertEquals(title, “Swag Labs”);
}
//Login into the application
@When(“User logged in the app using username {string} and password {string}”)
public void loginIntoTheApplication(String username,String password ) {
login.loginIntoApplication(username, password);
}
//Verify product name after login
@Then(“User verify the product name {string}”)
public void verifyProductsName(String productname) {
String productName = home.getProductName();
Assert.assertEquals(productName, productname);
}
//Logout from application
@Then(“User logout from the application”)
public void logoutFromApplication() {
login.logoutApplication();}
}
Step 9: Create testrunner Class to Run the Test Cases

package com.testrunner;
import org.junit.runner.RunWith;
import io.cucumber.junit.Cucumber;
import io.cucumber.junit.CucumberOptions;
@RunWith(Cucumber.class)
@CucumberOptions(
features = “src/test/java/features/”,
glue = {“stepdefinitions”},
plugin = {“pretty”})
public class SauceDemoTestRunner {
}
Step 10: Run the Test Case
Two ways of running the test case.
Method 1:
Open testrunner class right-click and Run as -> Junit Test.
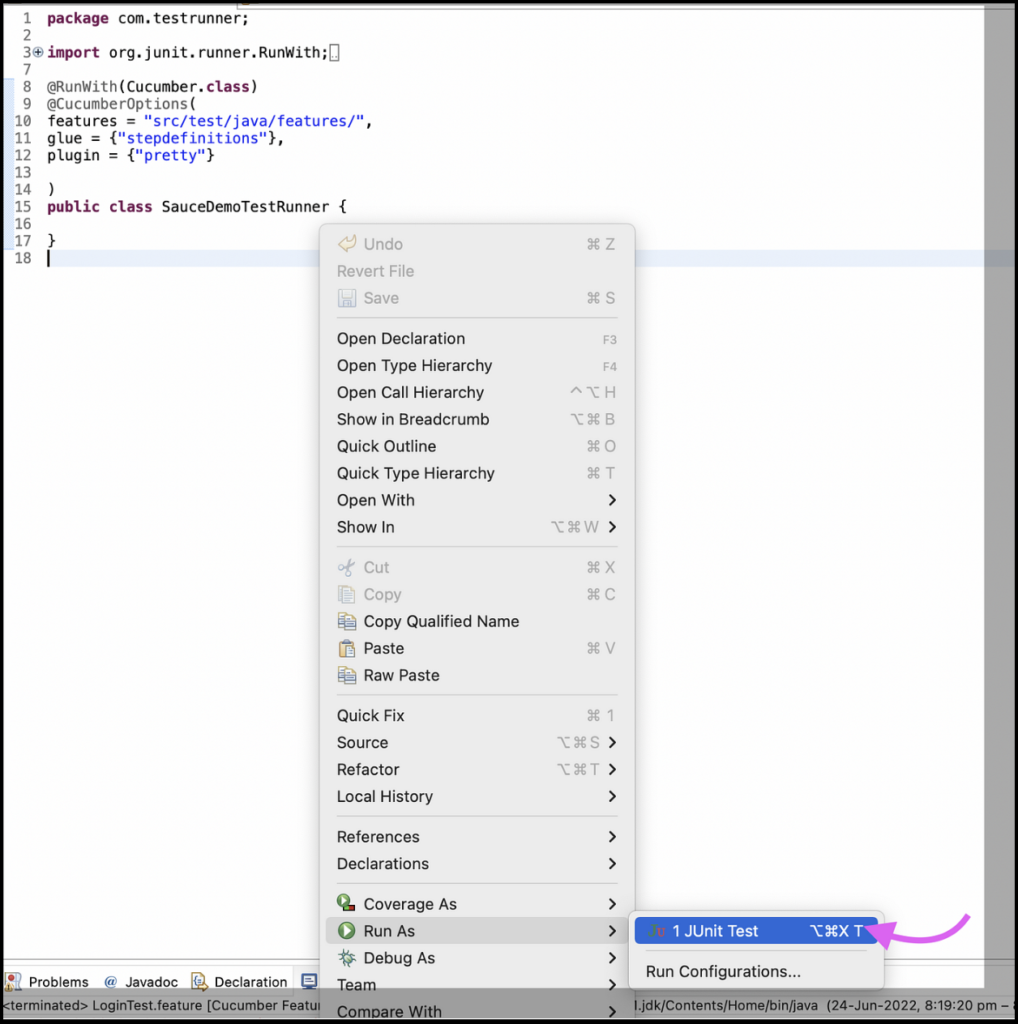
Method 2:
We can run it by right-clicking on the .feature file.
Report After Running the Test Cases
Conclusion
Integrating Cucumber with Playwright, you can leverage the expressive power of Cucumber’s feature files and step definitions to drive your Playwright automation tests. This combination allows you to write readable and maintainable tests that cover the behavior of your web application in a business-oriented language. Cucumber with Playwright in Java provides a seamless way to express and automate acceptance criteria, making it easier to collaborate and ensure the quality of your web applications.
Opinions expressed by DZone contributors are their own.
Comments